py2exe is a simple way to convert Python scripts into Windows .exe applications. It is an utility based in Distutils that allows you to run applications written in Python on a Windows computer without requiring the user to install Python. It is an excellent option when you need to distribute a program to the end user as a standalone application. py2exe currently only works in Python 2.x. Also Read: Estimation and Costing Notes PDF First you need to download and install py2exe from the official sourceforge site Now, in order to be able to create the executable we need to create a file called setup.py in the same folder where the script you want to be executable is located: # setup.py from distutils.core import setup import py2exe setup(console=[‘myscript.py’]) In the code above, we are going to create an executable for myscript.py. The setup function receives a parameter console=[‘myscript.py’] telling py2exe that we have a console application called myscript.py. Then in order to create the executable just run python setup.py py2exe from the Windows command prompt (cmd). You will see a lot of output and then two folders will be created: dist and build. The build folder is used by py2exe as a temporary folder to create the files needed for the executable. The dist folder stores the executable and…
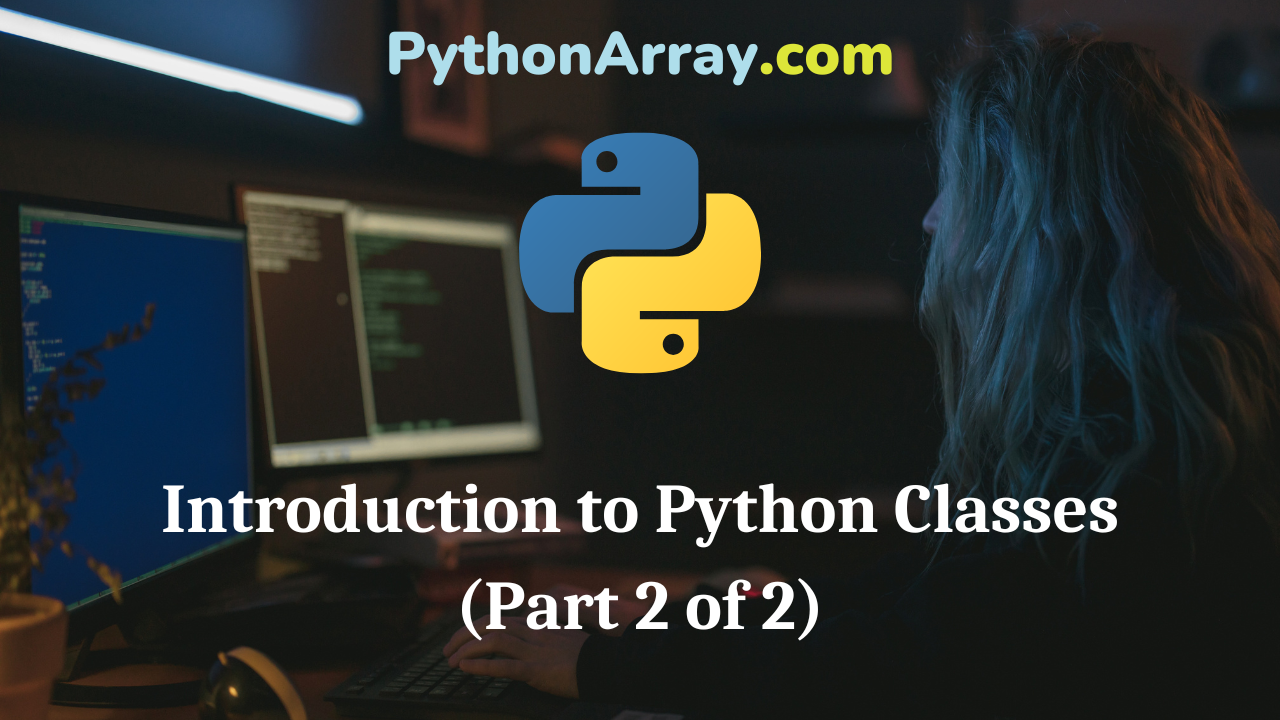
Introduction to Python Classes (Part 2 of 2)
In the first part of this series, we looked at the basics of using classes in Python. Now we’ll take a look at some more advanced topics. Also Read: Java Program to Find the Sum of Series x – x^3 + x^5 – x^7 + …… + N Python Class Inheritance Python classes support inheritance, which lets us take a class definition and extend it. Let’s create a new class that inherits (or derives) from the example in part 1: class Foo: def __init__(self, val): self.val = val def printVal(self): print(self.val) class DerivedFoo(Foo): def negateVal(self): self.val = -self.val This defines a class called DerivedFoo that has everything the Foo class has, and also adds a new method called negateVal. Here it is in action: >>> obj = DerivedFoo(42) >>> obj.printVal() 42 >>> obj.negateVal() >>> obj.printVal() -42 Python Programming – Method Overriding in Python Python Programming – Python Multilevel Inheritance Python Programming – Python Single Inheritance Inheritance becomes really useful when we re-define (or override) a method that is already defined in the base class: class DerivedFoo2(Foo): def printVal(self): print(‘My value is %s’ % self.val) We can test the class as follows: >>> obj2 = DerivedFoo2(42) >>> obj2.printVal() My value is 42 The derived class re-defines the printVal method to do something different,…
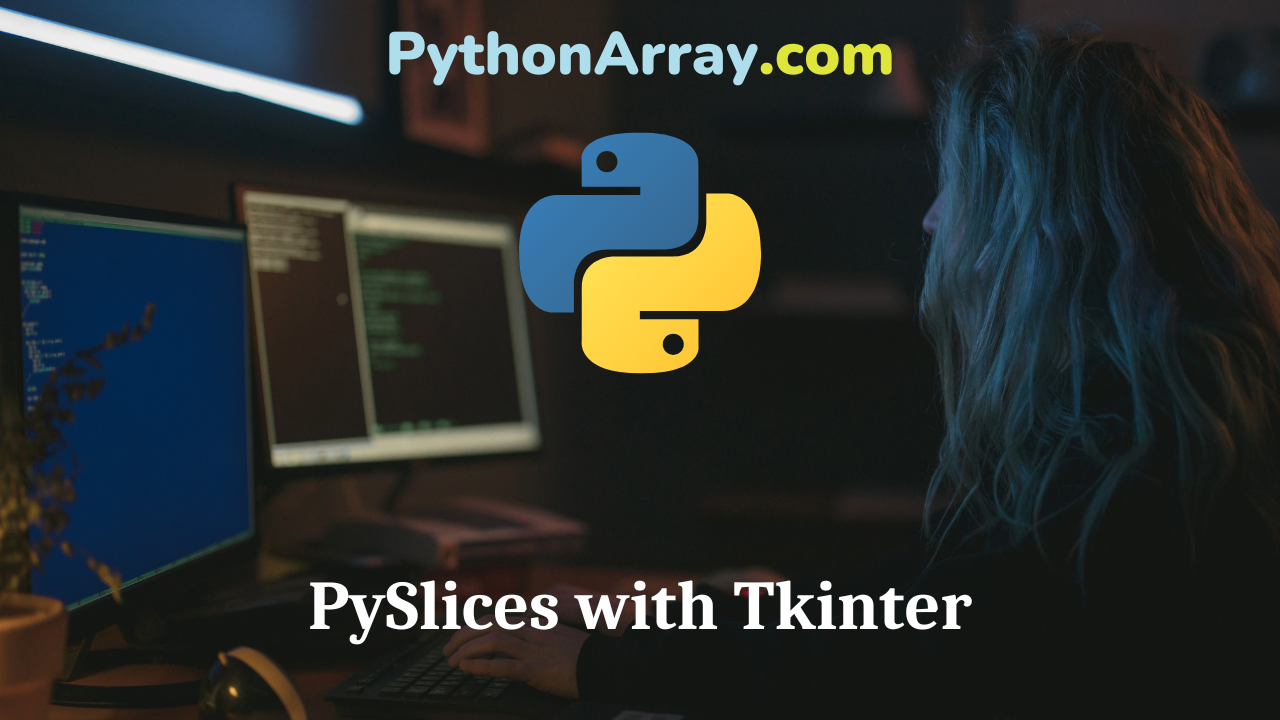
PySlices with Tkinter
Among the numerous libraries and software suites Python developers have created with and for Python is the Py Suite, a group of tools written in wxPython to assist Python enthusiasts with their programming endeavors. Also Read: Cloud Computing Notes Included in this suite is a Python shell worthy of mention called PySlices. Perhaps this shell’s most distinguishing feature is the capability to execute Python scripts written in notebook-style chunks of code without interfering with other chunks written in the same shell window (in addition to having a “normal” shell mode that can be activated in the Options > Settings menu). One way this can be extremely useful is when working with scripts that utilize the Tkinter library. PyInstaller: Package Python Applications (Windows, Mac and Linux) py2exe: Python to exe Introduction Overview of Python GUI development (Hello World) Using PySlices with Tkinter To illustrate the usefulness of PySlices, let’s examine the case where we were trying out some of the code in the Introduction to Tkinter article. In the standard Python IDLE, if we wanted to see the difference in output when incorporating the select() method for designating default RadioButtons, we’d have to type all of the code to initialize our widgets every time we wanted to change even something as small as selecting button “B”…
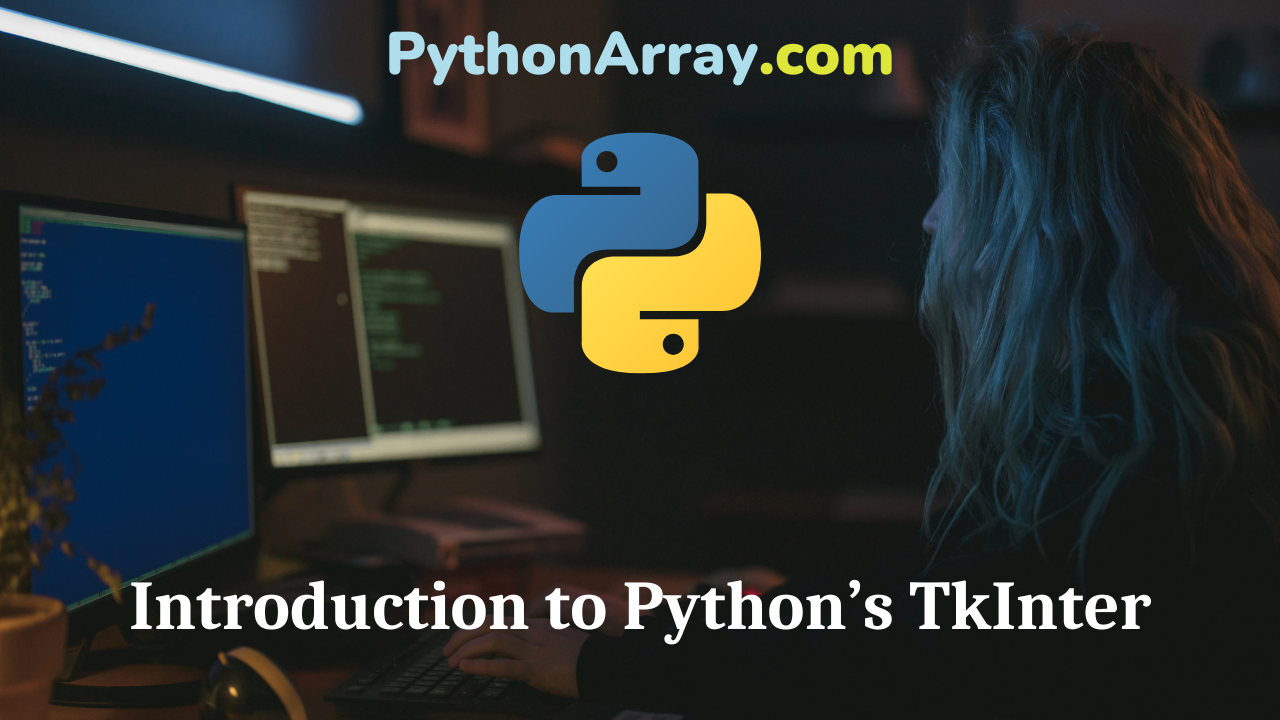
Introduction to Python’s TkInter
At some point in your Python experience, you might want to create a program with a graphical user interface or GUI (pronounced “gooey” by most). Many toolkits for Python exist to accomplish this, including Python’s own native GUI library, TkInter. Setting up GUIs in Tkinter isn’t complicated, though in the end it depends on the complexity of your own design. Here we will look at of the commonly used parts of the Tkinter module through some simple GUI examples. Before getting into the examples, let’s briefly cover the commonly used widgets (building blocks of the GUI) the Tkinter library has to offer. Also Read: Python: Add a Column to an Existing CSV File In a nutshell all of Tkinter’s widgets provide either simple input (user-input) to the GUI, output (graphical display of information) from the GUI, or a combination of the two. The following are two tables of the most commonly used widgets for input and output with brief descriptions. PySlices with Tkinter Overview of Python GUI development (Hello World) PyInstaller: Package Python Applications (Windows, Mac and Linux) Tkinter widgets commonly used for input: Widget Description Entry the Entry widget is the most basic text box, usually a one line-high…
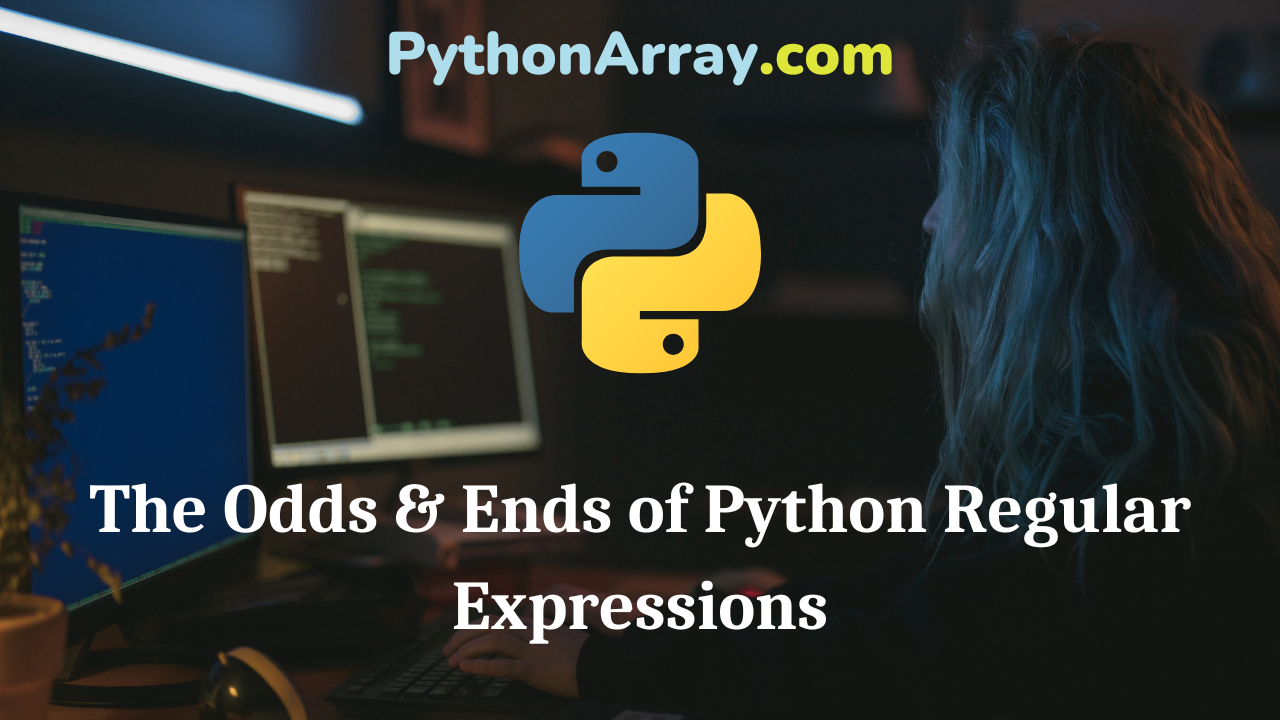
The Odds & Ends of Python Regular Expressions
In the first two parts of this series, we looked at some fairly advanced usage of regular expressions. In this part, we take a step back and look at some of the other functions Python offers in the re module, then we talk about some common mistakes people regularly (ha!) make. Also Read: Python Program to Find Sum of Odd Numbers Using Recursion in a List/Array Useful Python Regular Expression Functions Python offers several functions that make it easy to manipulate strings using regular expressions. With a single statement, Python can return a list of all the sub-strings that match a regular expression. For example: >>> s = ‘Hello world, this is a test!’ >>> print(re.findall(r’\S+’, s)) [‘Hello’, ‘world,’, ‘this’, ‘is’, ‘a’, ‘test!’] \S means any non-whitespace character, so the regular expression \S+ means match one or more non-whitespace characters (eg. a word). We can replace each matching sub-string with another string. For example: >>> print(re.sub( r’\S+’ , ‘WORD’, s)) WORD WORD WORD WORD WORD WORD The call to re.sub replaces all matches for the regular expression (eg. words) with the string “WORD”. Or if you want to iterate over each matching sub-string and process it yourself, re.finditer will loop over each match, returning a MatchObject on each iteration. For example: >>>…
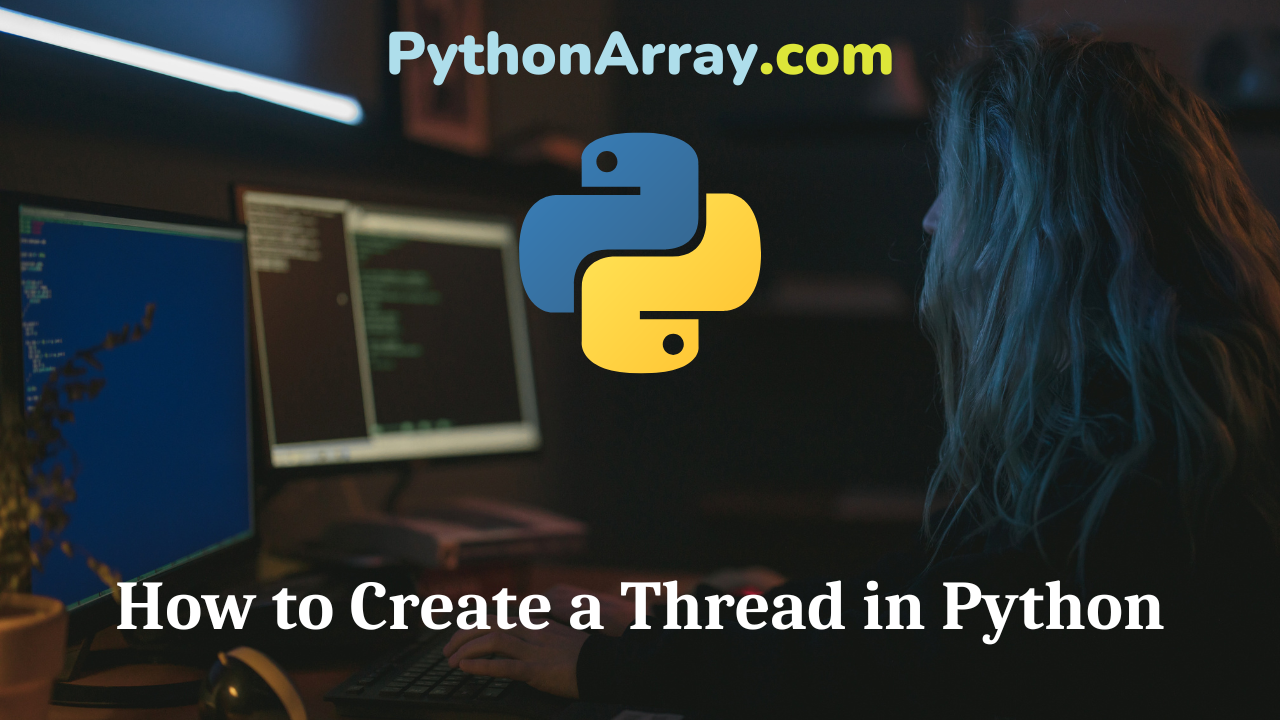
How to Create a Thread in Python
Introduction to Python threads What are threads? Simply put, try to imagine them as running several programs concurrently, in a single process. When you create one or more threads in your program, they get executed simultaneously, independent of each other, and most importantly, they can share information among them without any extra difficulty. Also Read: Start thread by member function with arguments These features make threads lightweight and handy in situations like network programming, when you try to ping (send network packets or requests) hundreds of workstations and you don’t want to ping them one after another! Since network replies may come after a significant amount of delay, the program will be extremely slow if you don’t ping many workstations concurrently. This article will show you how to create a thread in Python, and how to use them in general. How to Pickle Unpickle Tutorial | Understanding Python Pickling & Unpickling with Example Python Programming – Scope and Module Python for Android: Android’s Native Dialogs (SL4A) Create a Thread in Python Threading in Python is easy. First thing you need to do is to import Thread using the following code: from threading import Thread To create a thread in Python you’ll want…
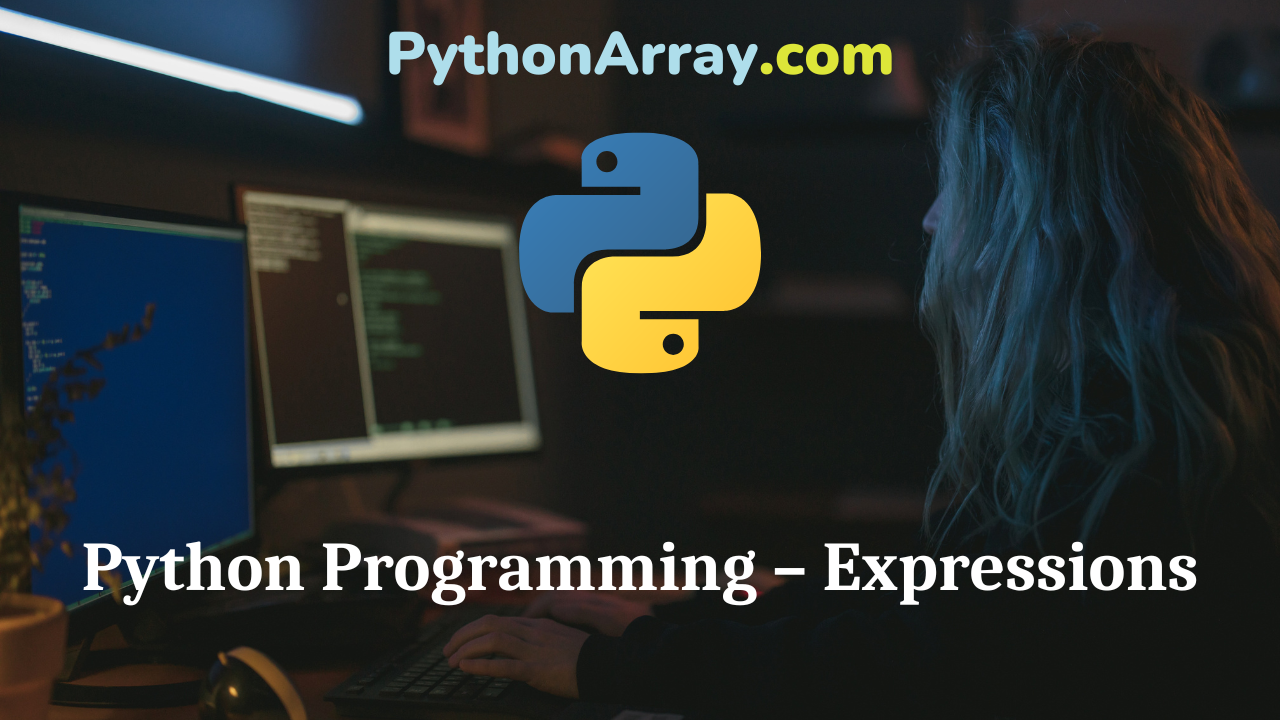
Python Programming – Expressions
You can learn about Operators in Python Programs with Outputs helped you to understand the language better. Also Read: Java Program to Convert Kilogram to Pound and Pound to Kilogram Python Programming – Expressions The expression consists of a combination of values, which can be constant values, such as a string (” Shiva ” ) or a number (12), and operators, which are symbols that act on the values in some way. The following are the examples of expression: 10 – 4 11 * ( 4 + 5 ) X – 5 a / b In other words, the expression consists of one or more operands and one or more operators linked together to compter a value. for example: a = 5 # assume a = 5 a + 2 is a larger expression that results in the sum of the value in the variable a is also an expression as is the constant 2 since they both represent the value. expression value, in turn, can act as Operands for Operators. For example, 9+6and 8+5+2 are two expressions that will result in a value of 15. taking another example, 6.0/5+(8-5.0) is an expression in which values of different data types are used.…
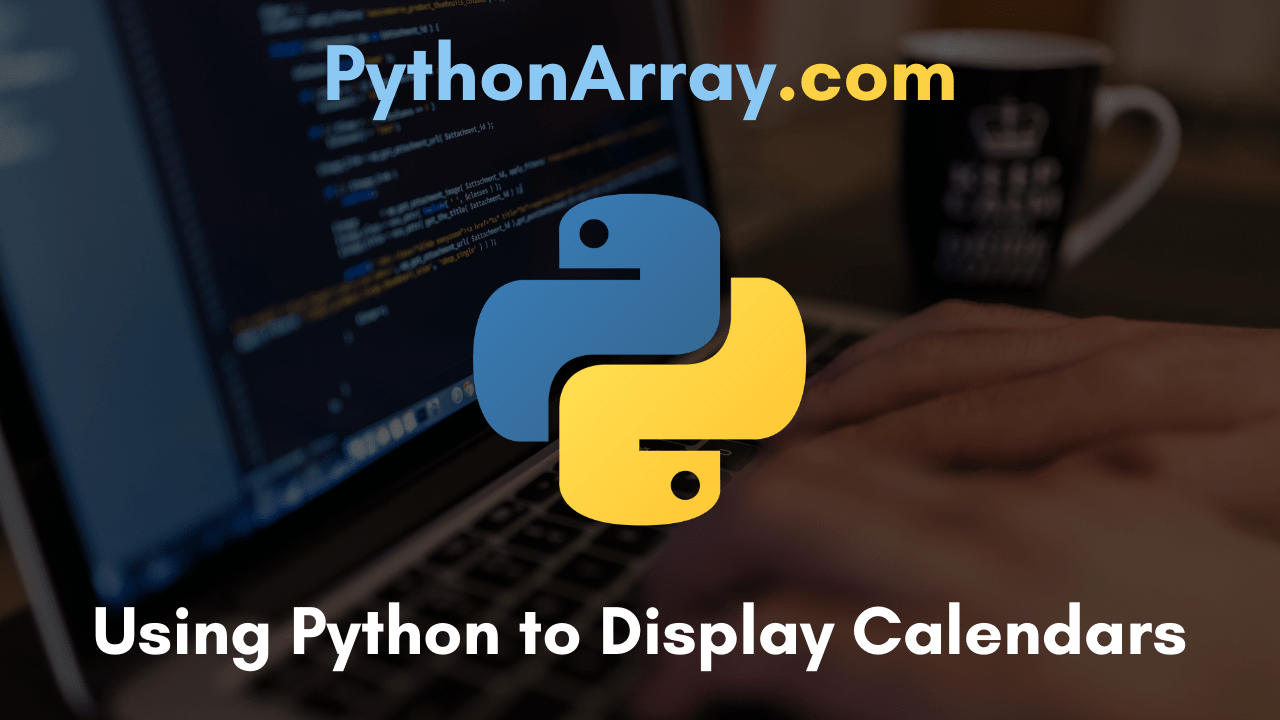
Using Python to Display Calendars | Learn How to Print a Calendar in Python with Example
Are you excited to learn how to display calendars using python programming? If yes, then here’s a fun thing about Python that you can be commonly known with it ie., there is a simple and interesting built-in function in python to display a calendar. If you want to get a good hold on this particular concept please go with this Using Python Display Calendars Tutorial. By using this tutorial of Python program to display calendars, you can easily and effectively print a calendar of your selected year and month. This Tutorial of Using Python to Print Calendars covers below helpful modules: Using Python Display Calendars Prerequisites for Learning Display Calendar using Python Python Program to Display Calendar Print a Calendar in Python Python calendar.weekday() method Calendar in Python Example Using Python Display Calendars In python, you can find plenty of bult-in functions for functioning many codes. Like that, it has a built-in calendar function you can use to display as many calendars as you like. When you import the function, you can use it to display a month of any year in standard calendar format (a month at the top, followed by 7 columns of days). Because it’s a built-in function,…
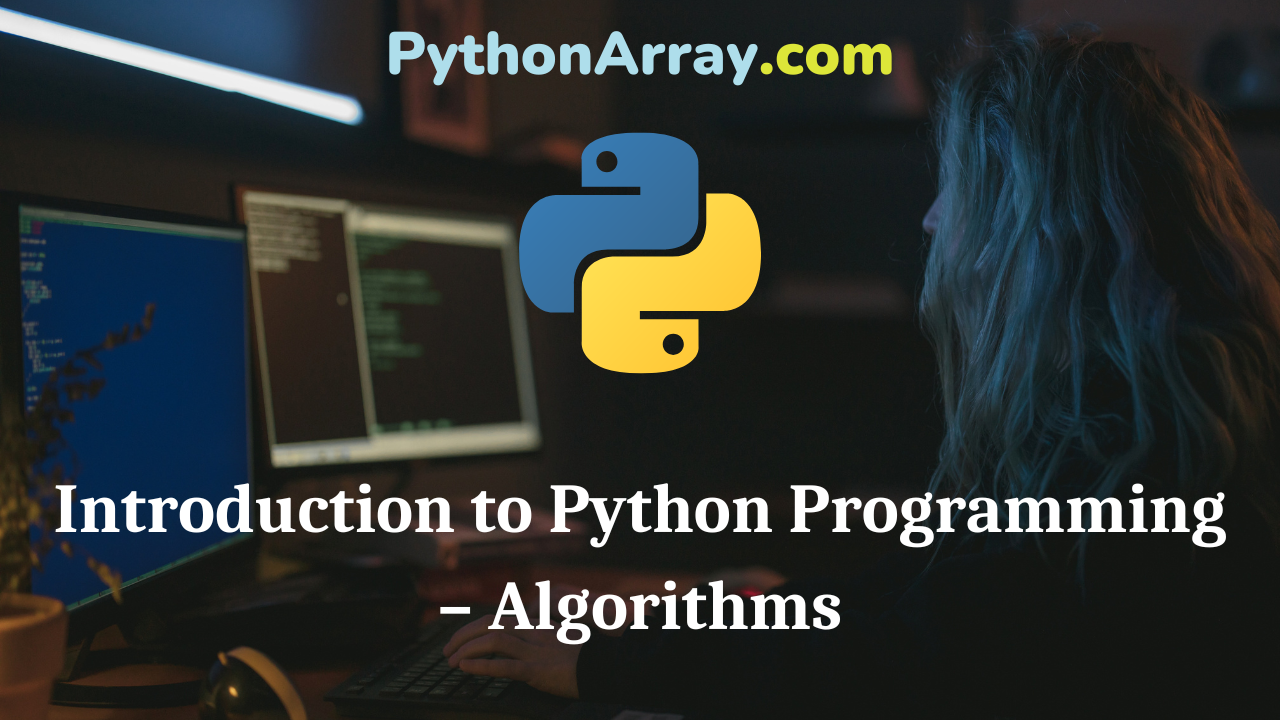
Introduction to Python Programming – Algorithms
You can learn about Introduction to Programming Using Python Programs with Outputs helped you to understand the language better. Also Read: Python Programming Examples with Output Introduction to Python Programming – Algorithms Algorithms A set of instructions that describes the steps to be followed to carry out an activity is called an algorithm or procedure for solving a problem. If the algorithm is written in the computer programming language, then such a set of instructions is called a program. The task of writing the computer program involves going through several stages. So, programming requires more than just writing programs. That means it requires writing algorithms, as well as testing the program for all types of errors. The algorithm is a stepwise presentation of the program in simple English. Characteristics of Algorithm (a) Language independent. (b) Simple, complete, unambiguous, step-by-step program flow. (c) No standard format or syntax required. (d) Helpful to understand problems and plan out solutions. Python Programming – Introduction to Python Interpreter and Program Execution Introduction to Python Programming – Programming Languages Introduction to Python Programming – Testing and Debugging Developing or Writing the Algorithms The process of developing an algorithm (to solve a specific problem) is a trial-and-error process…
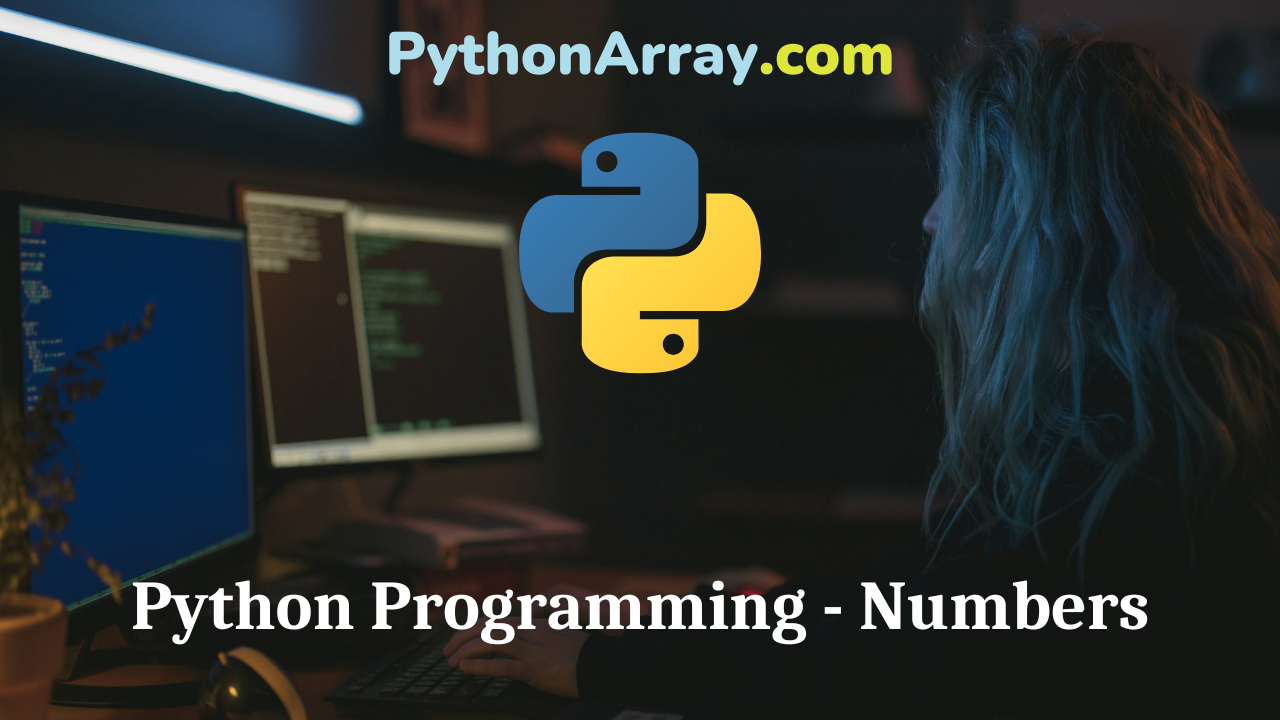
Python Programming – Numbers
Python Programming – Numbers A number is an object in Python, and it is created when some value is assigned to it. The number data type is used to hold numerical data. Python language supports four different numeric data types as described below: • int (signed integers): Alike C/C++/Java, Python supports integer numbers, which are whole numbers positive as well as negative having no decimal point. • long (long integers): Similar to integers but with limitless size. In Python long integers are followed by a lowercase or uppercase L. • float (floating point real values): Alike C/C++/Java, Python supports real numbers, called floats. These numbers are written with-a decimal point or sometimes in a scientific notation, with exponent e such as 5.9e7 i.e. 5.9xl07, where e represents the power of 10. • complex (complex numbers) : Unlike C/C++/Java, Python supports complex data type. It holds complex numbers of the form x+iy, where x and y are floating-point numbers and i is iota representing the square root of -1 (imaginary number). Complex numbers have two parts where x is known as the real part and y is called the imaginary, part as it is with iota. Unlike C/C++/Java, in Python, the…