Count Occurrences of a string This small script will use Pythons count method to count the numberof occurrences of a string. Also Read: Python Program to Count Non Palindrome words in a Sentence s = “Count, the number of spaces.” print s.count(” “) x = “I like to program in Python” print x.count(“i”) Python Programming – String Functions and Methods Python Programming – String Functions Extract a specific word from a string in Python
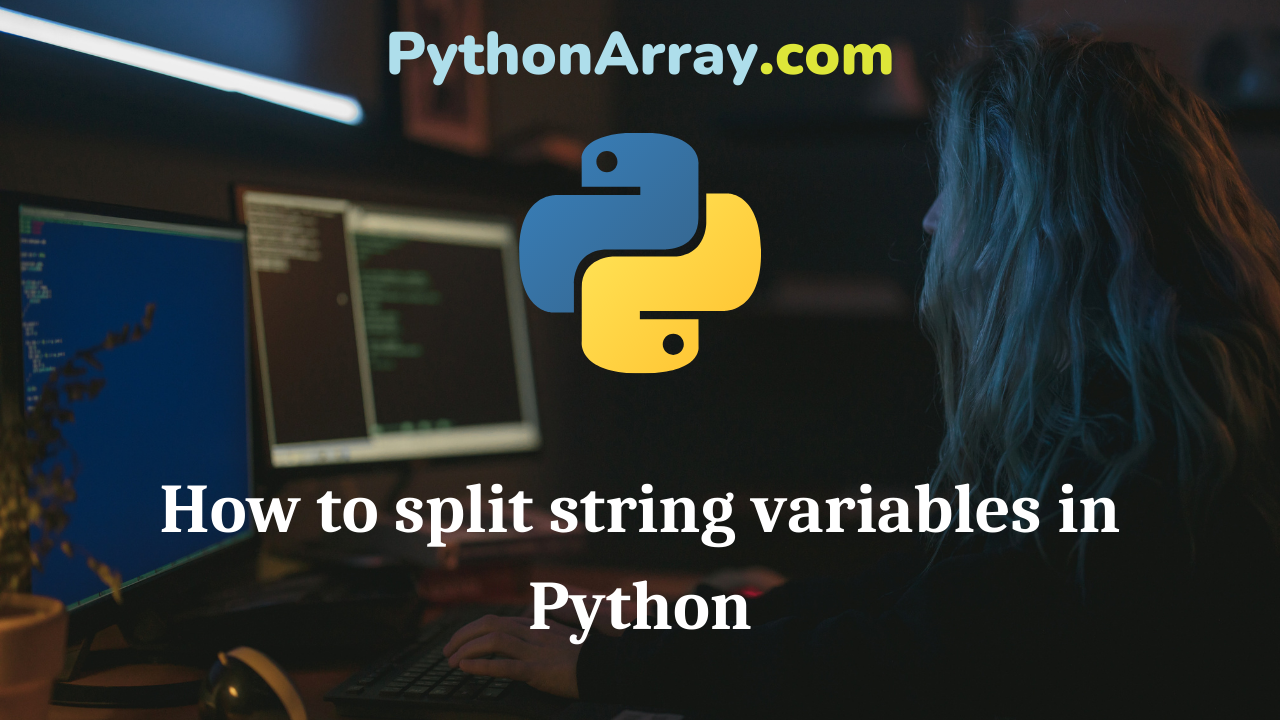
How to split string variables in Python
There may be many situations where we may need to split string variables. To split string variables in python we can use split() method, rsplit() method and splitlines() method with different arguments to the functions to accomplish different tasks. In this article, we will be looking at the several ways in which string variables can be split in python. Also Read: Textiles Engineering Notes Split Strings at Whitespaces To split string variables at each whitespace, we can use the split() function with no arguments. The syntax for split() function is split(separator, maxsplit) where the separator specifies the character at which the string should be split. maxsplit specifies the number of times the string has to be split. By default, separator has a value of whitespace which means that if no arguments are specified, the string will be split at whitespaces. The parameter maxsplit has a default value of -1 which denotes that string should be split at all the occurrences of the separator. When we invoke the split() method at any string without any argument, it will perform the python string split operation at every place where whitespace is present and returns a list of the substrings. Example myString=”Python For Beginners” print(“The string is:”) print(myString) myList=myString.split() print(“Output List is:”) print(myList) Output: The string is: Python For Beginners Output List is: [‘Python’, ‘For’, ‘Beginners’] Python Programming – String Functions Python…
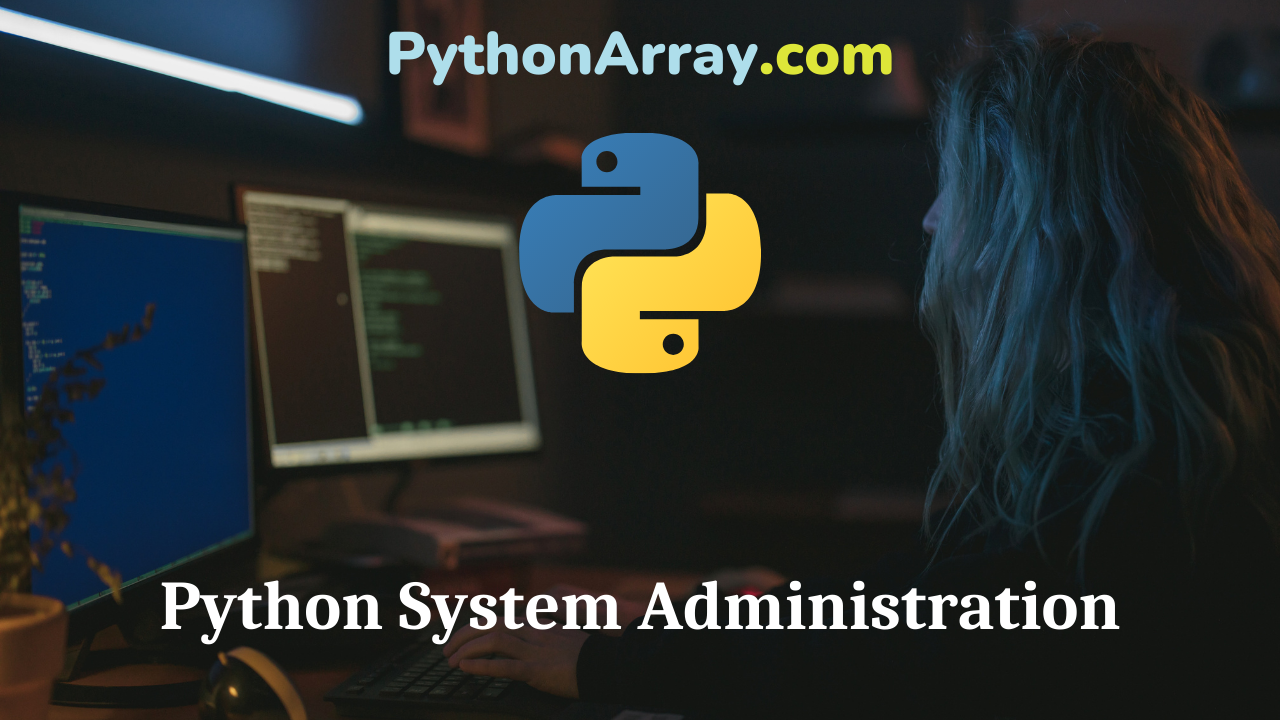
Python System Administration
Overview The OS module in Python provides a way of using operating system dependent functionality. The functions that the OS module provides allows you to interface with the underlying operating system that Python is running on. (Windows, Mac or Linux. You can find important information about your location or about the process. Read Also: Operating System Notes Before we start, make sure that you have imported the OS module “import os” Python Programming – Python Directory Methods OS.Walk and Fnmatch in Python How to Traverse a Directory Tree in Python – Guide to os.walk OS Functions explained os.system() # Executing a shell command os.stat() # Get the status of a file os.environ() # Get the users environment os.chdir() # Move focus to a different directory os.getcwd() # Returns the current working directory os.getgid() # Return the real group id of the current process os.getuid() # Return the current process’s user id os.getpid() # Returns the real process ID of the current process os.getlogin() # Return the name of the user logged os.access() # Check read permissions os.chmod() # Change the mode of path to the numeric mode os.chown() # Change the owner and group id os.umask(mask) # Set the current…
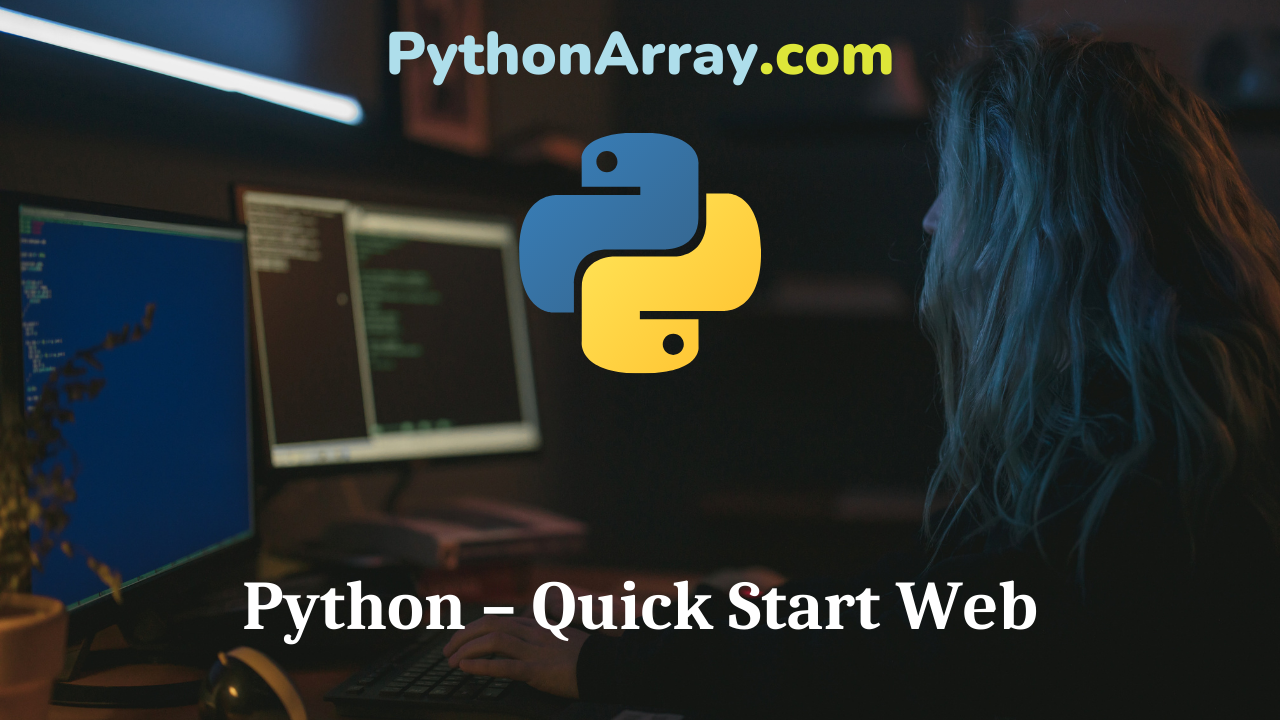
Python – Quick Start Web
Python Quick Start Web This post will be a collection of the posts we have written about Python for the web. What is Python for the Web? Basically, python programs that interface/scrape websites and/or utilize web based APIs. If you like what you read, please take a moment to share it using the buttons above. How to use Reddit API in Python In the API documentation, you can see there are tons of things to do. How to access various Web Services in Python A very good way of learning Python is trying to work with various Web Services API’s. In order to answer that, we will first have to get some knowledge about API’s, JSON, Data structures etc. Also Read: Python Program to Print the Equilateral Triangle Pattern of Star Web Scraping with BeautifulSoup “Web scraping (web harvesting or web data extraction) is a computer software technique of extracting information from websites.” HTML parsing is easy in Python, especially with help of the BeautifulSoup library. In this post we will scrape a website (our own) to extract all URL’s. Scraping websites with Python List of Python API’s How to access various Web Services in Python Tweet Search with Python Twitter’s API is REST-based and will return results…
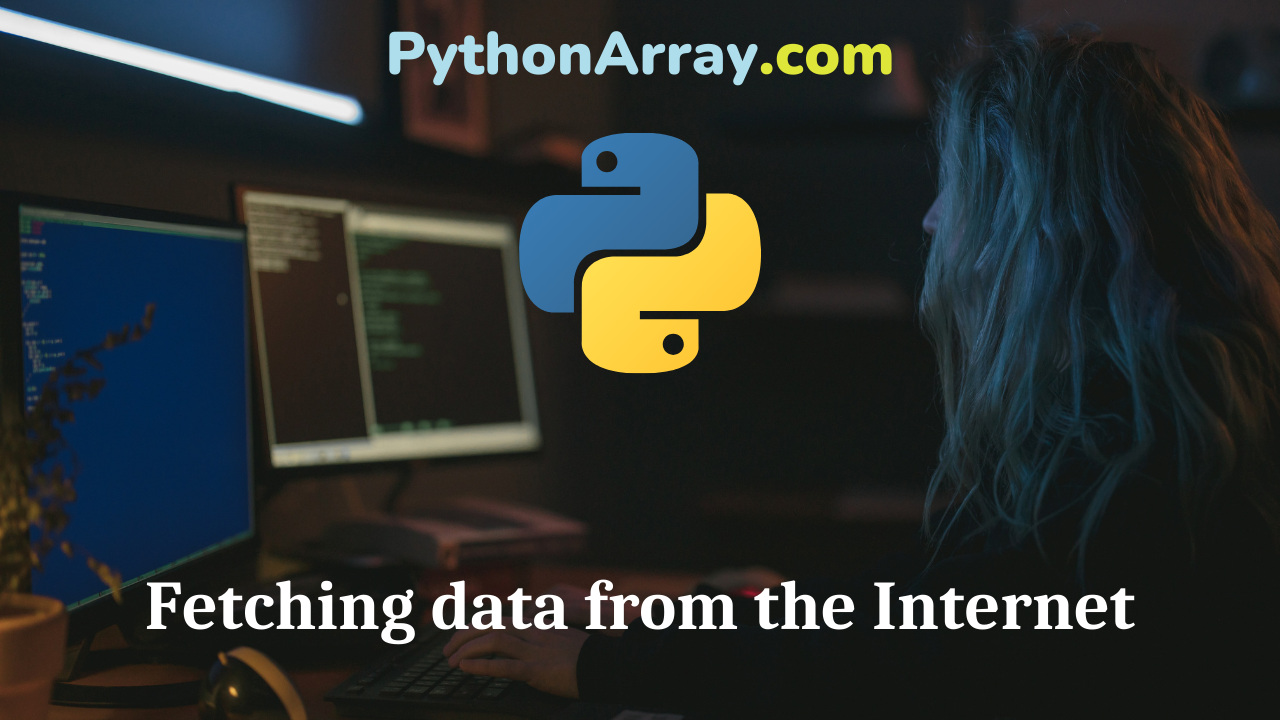
Fetching data from the Internet
What is Urllib2? urllib2 is a Python module for fetching URLs. What can it do? It offers a very simple interface, in the form of the urlopen function. Urlopen is capable of fetching URLs using a variety of different protocols like (http, ftp, file). It also offers an interface for handling basic authentication, cookies, proxies and so on. These are provided by objects called handlers and openers. Python Modules Urllib2 User Agent CommandLineFu with Python Scraping websites with Python HTTP Requests HTTP is based on requests and responses, in that the client makes requests and the servers send responses. This response is a file-like object, which means you can for example call .read() on the response. How can I use it? import urllib2 response = urllib2.urlopen(‘http://python.org/’) html = response.read() User Agents You can also add your own headers with urllib2. Some websites dislike being browsed by programs. By default urllib2 identifies itself as Python-urllib/x.y (where x and y are the major and minor version numbers of the Python release, which may confuse the site, or just plain not work. The way a browser identifies itself is through the User-Agent header. See our Urllib2 User Agent post that describes how to use that…
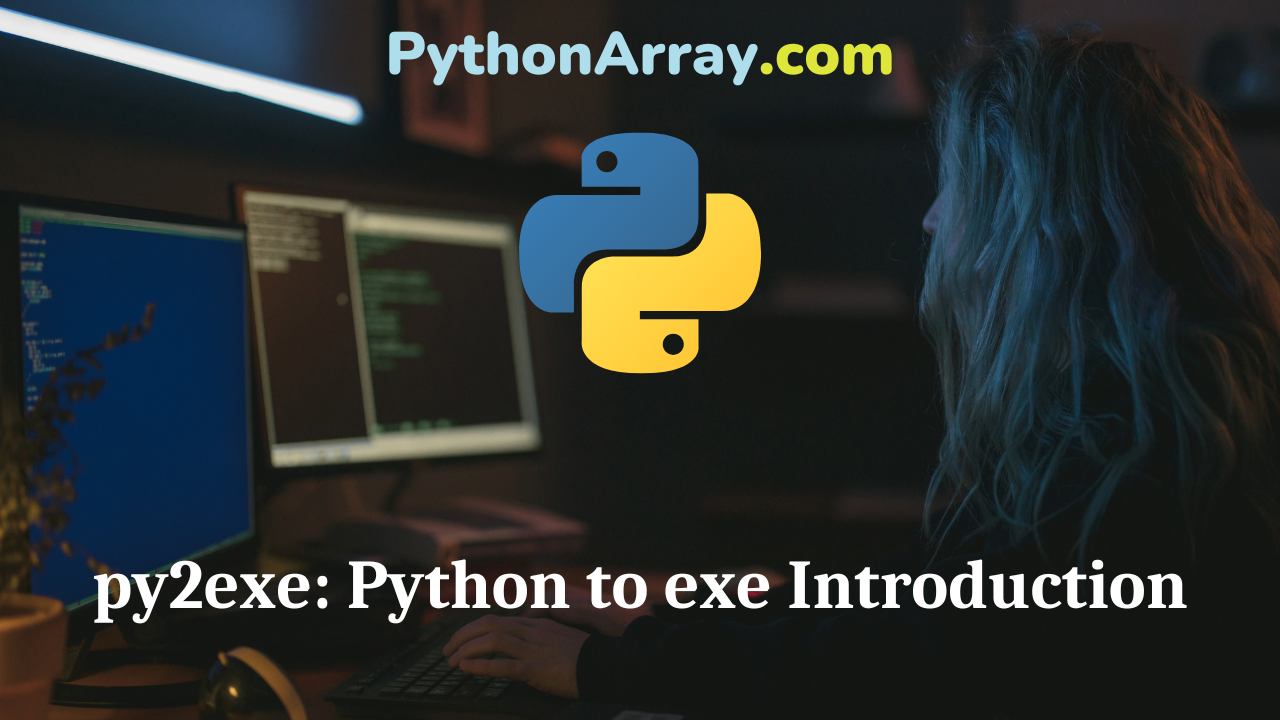
py2exe: Python to exe Introduction
py2exe is a simple way to convert Python scripts into Windows .exe applications. It is an utility based in Distutils that allows you to run applications written in Python on a Windows computer without requiring the user to install Python. It is an excellent option when you need to distribute a program to the end user as a standalone application. py2exe currently only works in Python 2.x. Also Read: Estimation and Costing Notes PDF First you need to download and install py2exe from the official sourceforge site Now, in order to be able to create the executable we need to create a file called setup.py in the same folder where the script you want to be executable is located: # setup.py from distutils.core import setup import py2exe setup(console=[‘myscript.py’]) In the code above, we are going to create an executable for myscript.py. The setup function receives a parameter console=[‘myscript.py’] telling py2exe that we have a console application called myscript.py. Then in order to create the executable just run python setup.py py2exe from the Windows command prompt (cmd). You will see a lot of output and then two folders will be created: dist and build. The build folder is used by py2exe as a temporary folder to create the files needed for the executable. The dist folder stores the executable and…
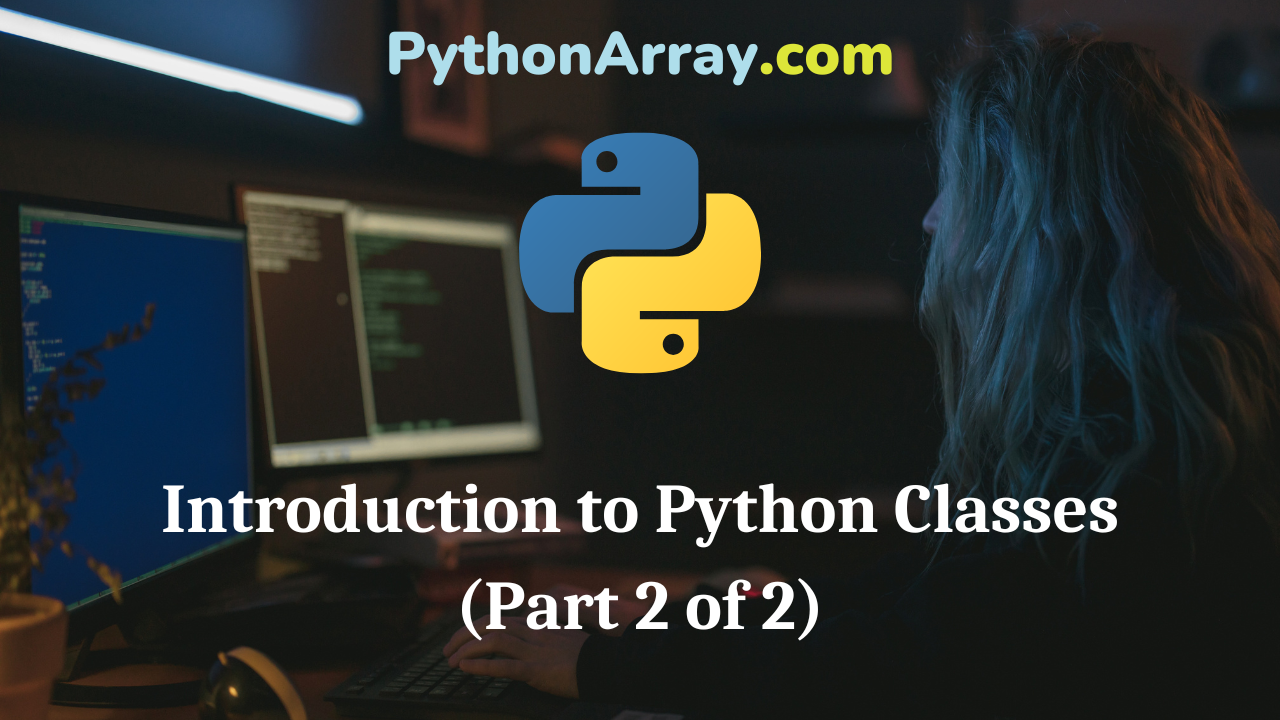
Introduction to Python Classes (Part 2 of 2)
In the first part of this series, we looked at the basics of using classes in Python. Now we’ll take a look at some more advanced topics. Also Read: Java Program to Find the Sum of Series x – x^3 + x^5 – x^7 + …… + N Python Class Inheritance Python classes support inheritance, which lets us take a class definition and extend it. Let’s create a new class that inherits (or derives) from the example in part 1: class Foo: def __init__(self, val): self.val = val def printVal(self): print(self.val) class DerivedFoo(Foo): def negateVal(self): self.val = -self.val This defines a class called DerivedFoo that has everything the Foo class has, and also adds a new method called negateVal. Here it is in action: >>> obj = DerivedFoo(42) >>> obj.printVal() 42 >>> obj.negateVal() >>> obj.printVal() -42 Python Programming – Method Overriding in Python Python Programming – Python Multilevel Inheritance Python Programming – Python Single Inheritance Inheritance becomes really useful when we re-define (or override) a method that is already defined in the base class: class DerivedFoo2(Foo): def printVal(self): print(‘My value is %s’ % self.val) We can test the class as follows: >>> obj2 = DerivedFoo2(42) >>> obj2.printVal() My value is 42 The derived class re-defines the printVal method to do something different,…
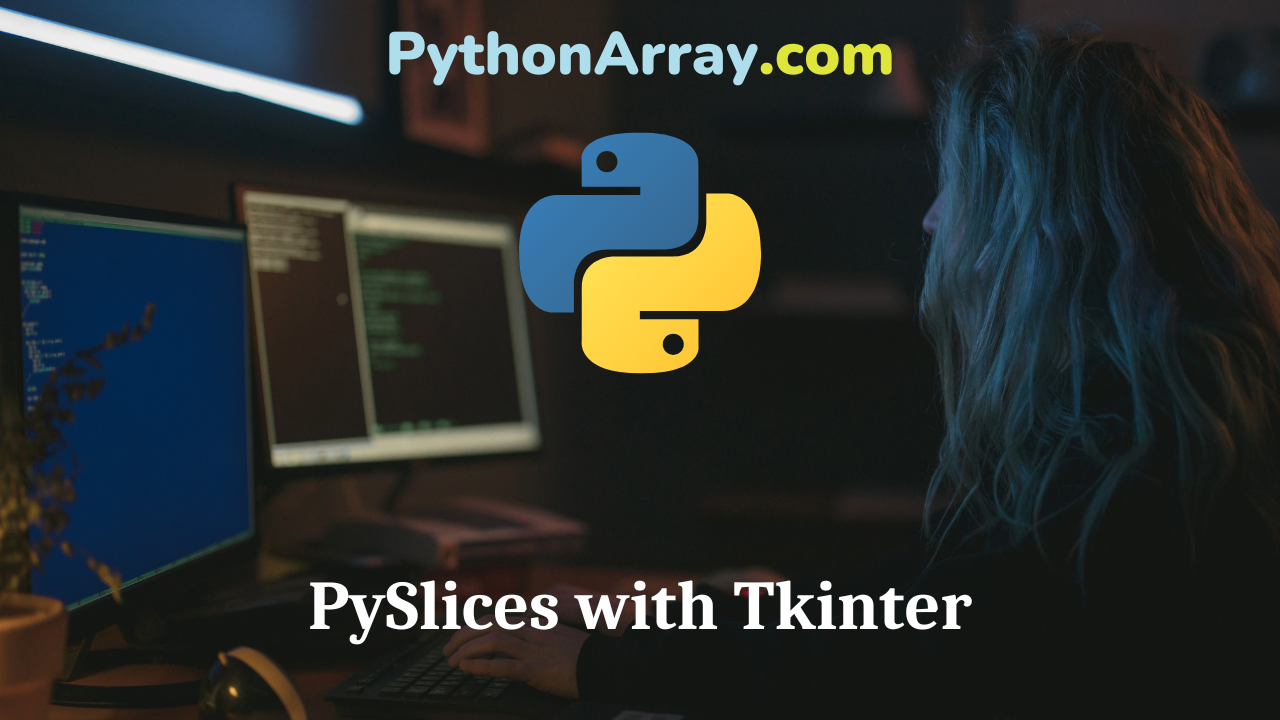
PySlices with Tkinter
Among the numerous libraries and software suites Python developers have created with and for Python is the Py Suite, a group of tools written in wxPython to assist Python enthusiasts with their programming endeavors. Also Read: Cloud Computing Notes Included in this suite is a Python shell worthy of mention called PySlices. Perhaps this shell’s most distinguishing feature is the capability to execute Python scripts written in notebook-style chunks of code without interfering with other chunks written in the same shell window (in addition to having a “normal” shell mode that can be activated in the Options > Settings menu). One way this can be extremely useful is when working with scripts that utilize the Tkinter library. PyInstaller: Package Python Applications (Windows, Mac and Linux) py2exe: Python to exe Introduction Overview of Python GUI development (Hello World) Using PySlices with Tkinter To illustrate the usefulness of PySlices, let’s examine the case where we were trying out some of the code in the Introduction to Tkinter article. In the standard Python IDLE, if we wanted to see the difference in output when incorporating the select() method for designating default RadioButtons, we’d have to type all of the code to initialize our widgets every time we wanted to change even something as small as selecting button “B”…
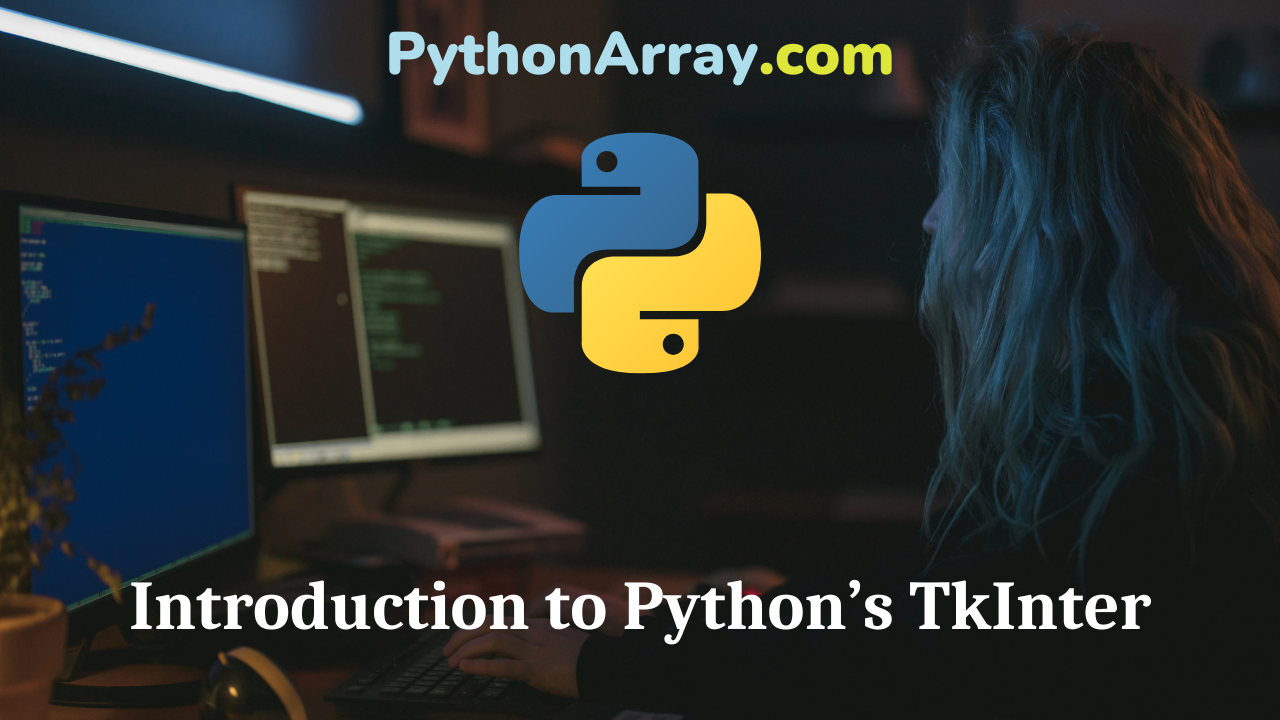
Introduction to Python’s TkInter
At some point in your Python experience, you might want to create a program with a graphical user interface or GUI (pronounced “gooey” by most). Many toolkits for Python exist to accomplish this, including Python’s own native GUI library, TkInter. Setting up GUIs in Tkinter isn’t complicated, though in the end it depends on the complexity of your own design. Here we will look at of the commonly used parts of the Tkinter module through some simple GUI examples. Before getting into the examples, let’s briefly cover the commonly used widgets (building blocks of the GUI) the Tkinter library has to offer. Also Read: Python: Add a Column to an Existing CSV File In a nutshell all of Tkinter’s widgets provide either simple input (user-input) to the GUI, output (graphical display of information) from the GUI, or a combination of the two. The following are two tables of the most commonly used widgets for input and output with brief descriptions. PySlices with Tkinter Overview of Python GUI development (Hello World) PyInstaller: Package Python Applications (Windows, Mac and Linux) Tkinter widgets commonly used for input: Widget Description Entry the Entry widget is the most basic text box, usually a one line-high…
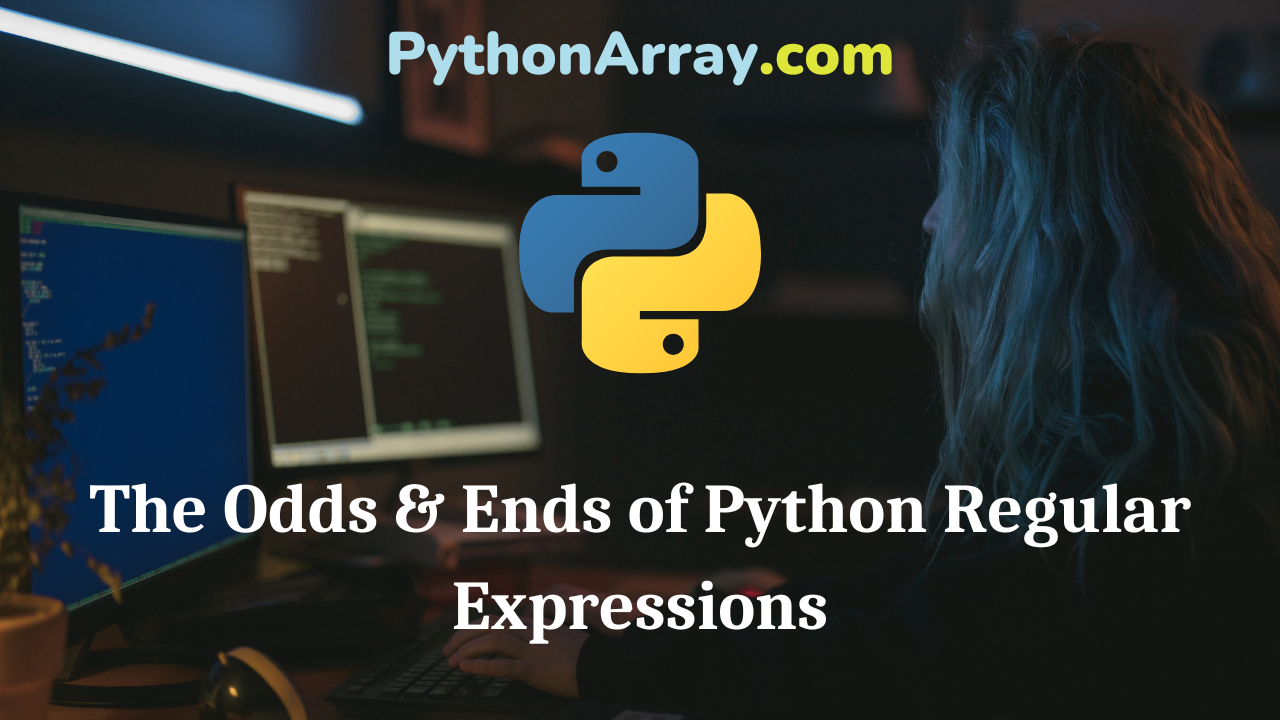
The Odds & Ends of Python Regular Expressions
In the first two parts of this series, we looked at some fairly advanced usage of regular expressions. In this part, we take a step back and look at some of the other functions Python offers in the re module, then we talk about some common mistakes people regularly (ha!) make. Also Read: Python Program to Find Sum of Odd Numbers Using Recursion in a List/Array Useful Python Regular Expression Functions Python offers several functions that make it easy to manipulate strings using regular expressions. With a single statement, Python can return a list of all the sub-strings that match a regular expression. For example: >>> s = ‘Hello world, this is a test!’ >>> print(re.findall(r’\S+’, s)) [‘Hello’, ‘world,’, ‘this’, ‘is’, ‘a’, ‘test!’] \S means any non-whitespace character, so the regular expression \S+ means match one or more non-whitespace characters (eg. a word). We can replace each matching sub-string with another string. For example: >>> print(re.sub( r’\S+’ , ‘WORD’, s)) WORD WORD WORD WORD WORD WORD The call to re.sub replaces all matches for the regular expression (eg. words) with the string “WORD”. Or if you want to iterate over each matching sub-string and process it yourself, re.finditer will loop over each match, returning a MatchObject on each iteration. For example: >>>…