Mechanize A very useful python module for navigating through web forms is Mechanize. In a previous post I wrote about “Browsing in Python with Mechanize”. Today I found this excellent cheat sheet on scraperwiki that I would like to share. Also Read: Iterate over string c++ – C++ iterate over set – Different Ways to Iterate Over a Set in C++ Create a browser object Create a browser object and give it some optional settings. import mechanize br = mechanize.Browser() br.set_all_readonly(False) # allow everything to be written to br.set_handle_robots(False) # ignore robots br.set_handle_refresh(False) # can sometimes hang without this br.addheaders = # [(‘User-agent’, ‘Firefox’)] Python Cheat Sheets Loops in Python Scraping websites with Python Open a webpage Open a webpage and inspect its contents response = br.open(url) print response.read() # the text of the page response1 = br.response() # get the response again print response1.read() # can apply lxml.html.fromstring() Using forms List the forms that are in the page for form in br.forms(): print “Form name:”, form.name print form To go on the mechanize browser object must have a form selected br.select_form(“form1”) # works when form has a name br.form = list(br.forms())[0] # use when form is unnamed Using Controls Iterate through the…
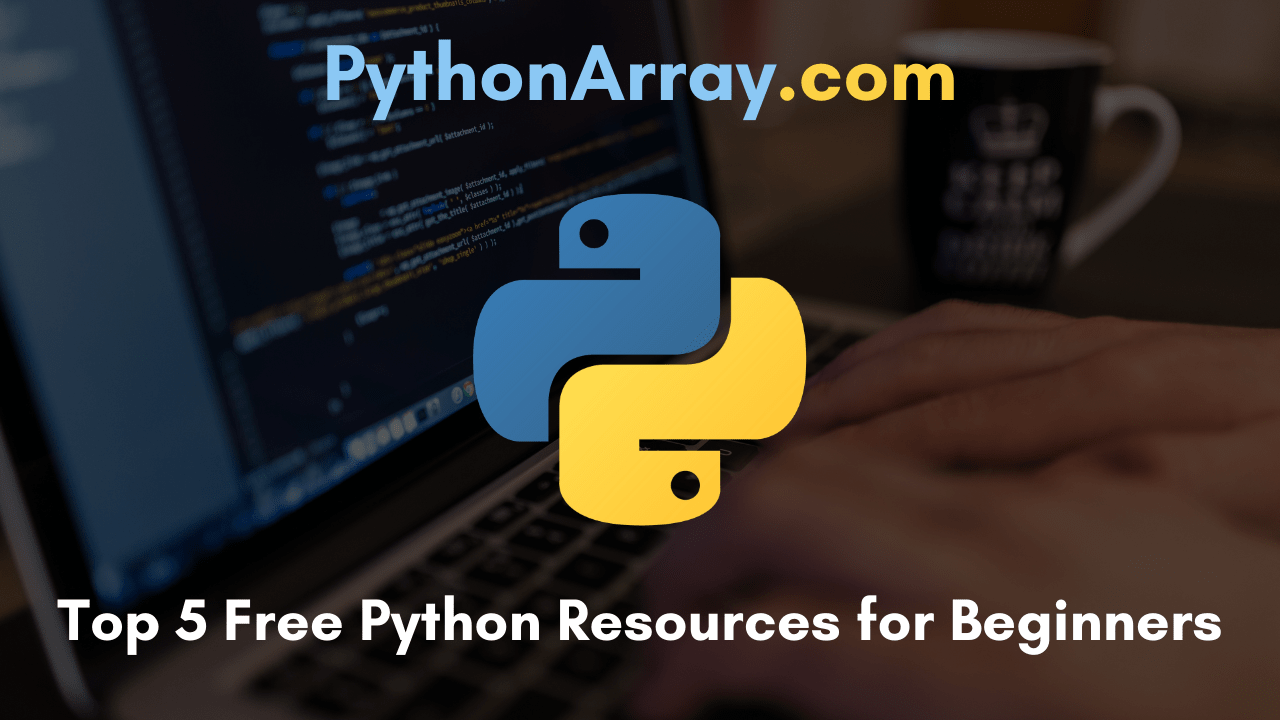
Top 5 Free Python Resources for Beginners | Best Free Python Learning Resources
Do you have N number of questions raised in your head after deciding to learn the Python programming language? Wondering how to get solutions for your queries as a beginner in python? Then, you’ve come to the right place. This Best Free Python Learning Resources for Beginners Tutorial is the perfect solution. From here, you will find the answers to all your queries like Where do you start? How do you know you’re getting the best possible information? What do you do once you’ve gotten down to the basics? Also, you will discover the list of top 5 free Python learning resources for newbies and skilled coders just starting out with Python. Stay Tuned to it and continue your read! Build Games for Python Using Pygame | What is PyGame? | Basic PyGame Program Quick Tip: Using Python’s Comparison Operators | Chaining Comparison Operators in Python How to Pickle Unpickle Tutorial | Understanding Python Pickling & Unpickling with Example What is Python? A high-level, interpreted, and open-source language is called Python. Python helps both object-oriented and procedural programming paradigms. The collection of python’s built-in functions are very huge and supports a lot while coding basic to complex programs. Also Read: …
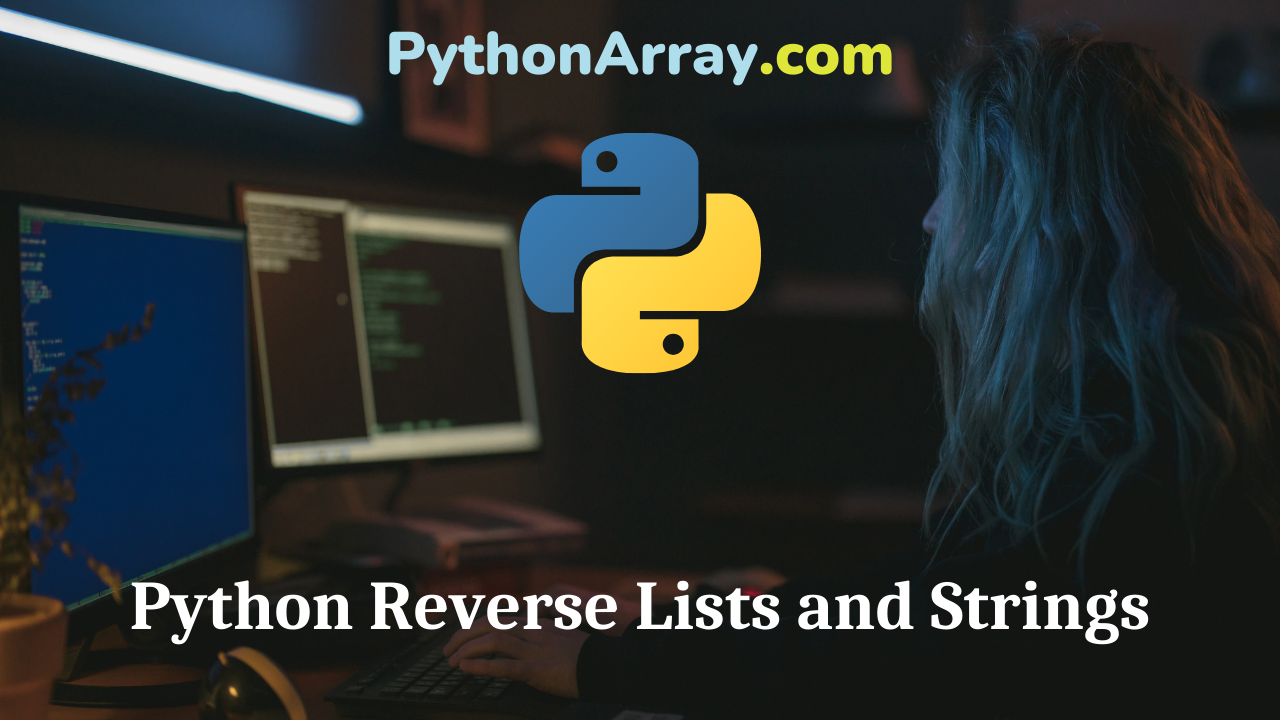
Python Reverse Lists and Strings
This short post will show how to do a reverse loop in Python. The first example will show how to do that in a list a and the other example how to reverse a string. Also Read: Python Program to Check if a String is a Pangram or Not Just open up a Python interpreter and test it out. Python Lists Cheat Sheet Python Programming – List Functions And Methods String Manipulation in Python Create a list with some values in it, like this: L1 = [“One”, “two”, “three”, “four”, “five”] #To print the list as it is, simply do: print L1 #To print a reverse list, do: for i in L1[::-1]: print i >>Output: five four three two One We can also reverse strings, like this: string = “Hello World” print ‘ ‘.join(reversed(string)) >>Output: d l r o W o l l e H
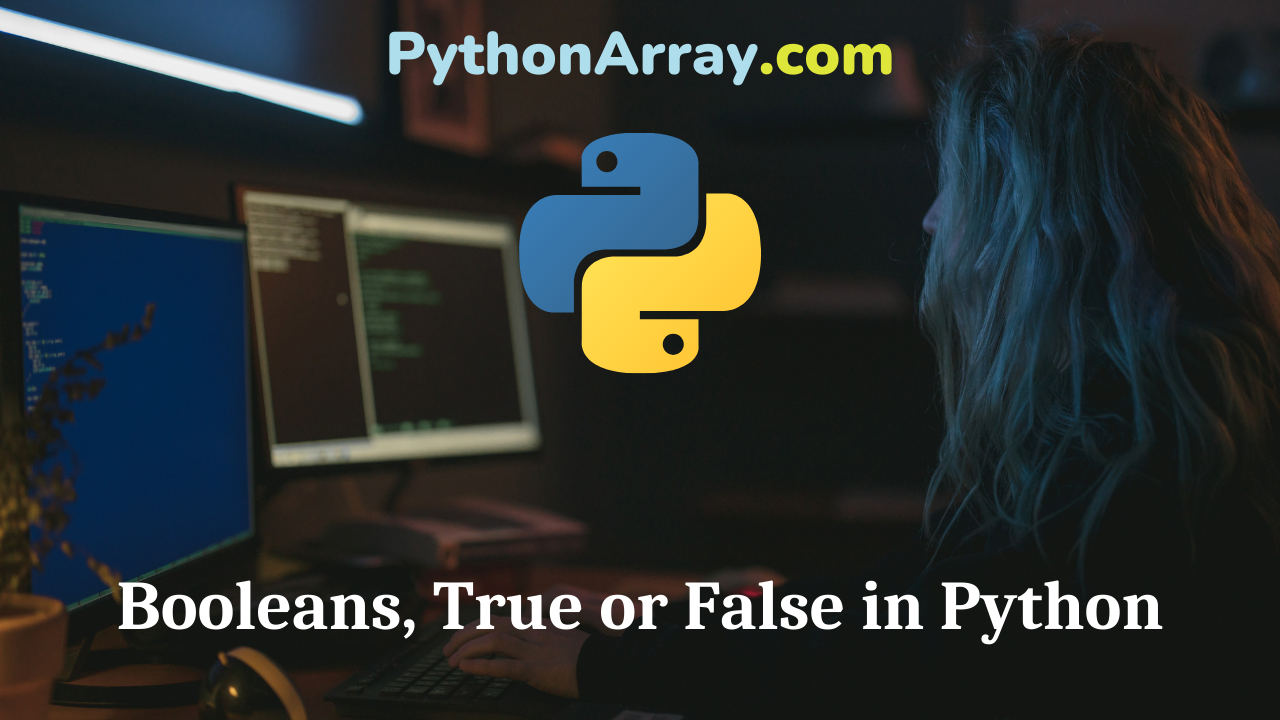
Booleans, True or False in Python
What are Boolean? Boolean values are the two constant objects False and True. They are used to represent truth values (other values can also be considered false or true). In numeric contexts (for example, when used as the argument to an arithmetic operator), they behave like the integers 0 and 1, respectively. The built-in function bool() can be used to cast any value to a Boolean if the value can be interpreted as a truth value They are written as False and True, respectively. Quick Tip: Using Python’s in Operator Quick Tip: Using Python’s Comparison Operators | Chaining Comparison Operators in Python How to Check if a List, Tuple or Dictionary is Empty in Python Boolean Strings A string in Python can be tested for truth value. The return type will be in Boolean value (True or False) Let’s make an example, by first create a new variable and give it a value. my_string = “Hello World” my_string.isalnum() #check if all char are numbers my_string.isalpha() #check if all char in the string are alphabetic my_string.isdigit() #test if string contains digits my_string.istitle() #test if string contains title words my_string.isupper() #test if string contains upper case my_string.islower() #test if string contains lower…
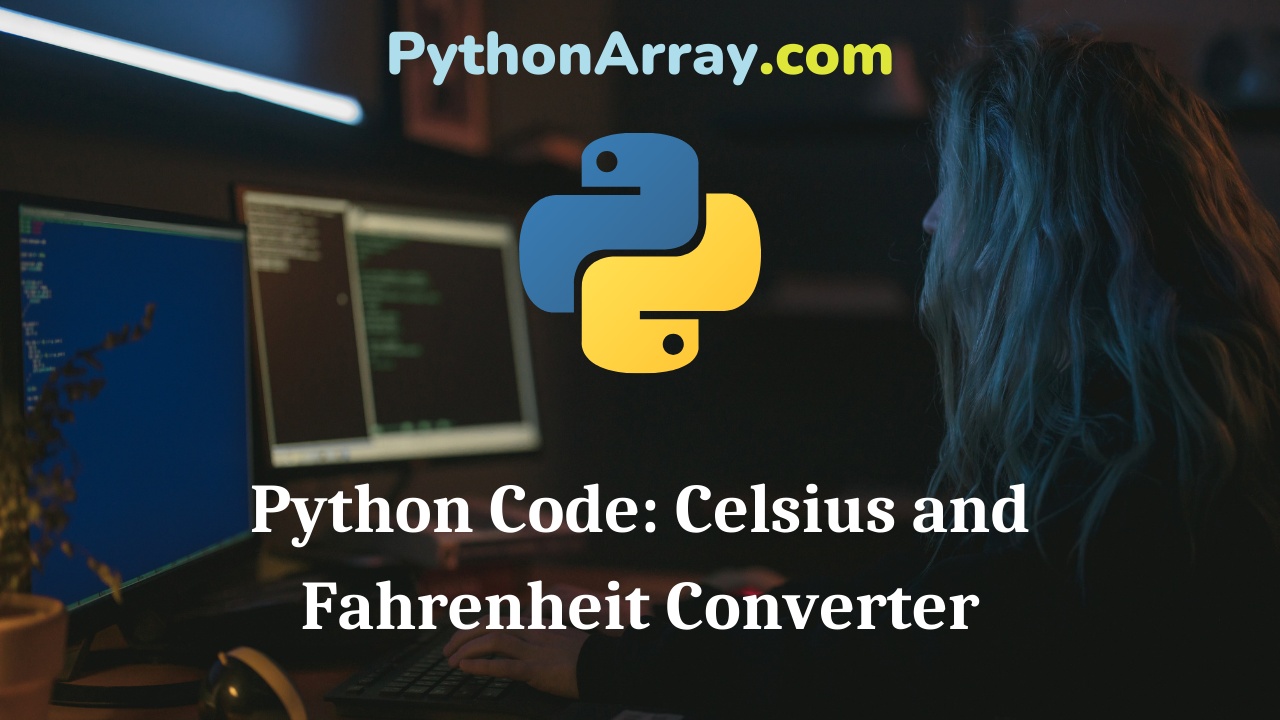
Python Code: Celsius and Fahrenheit Converter
Overview This script converts temperature between Fahrenheit to Celsius. To create a python converter for celsius and Fahrenheit, you first have to find out which formula to use. How to Use Python to Convert Fahrenheit to Celsius | Python Program to Convert Fahrenheit to Celsius & Vice Versa Python Functions Cheat Sheet How to Use Python to Convert Miles to Kilometers | Python Program to Convert Miles to KM Unit Measurement Fahrenheit to Celsius formula: (°F – 32) x 5/9 = °C or in plain English, First subtract 32, then multiply by 5, then divide by 9. Celsius to Fahrenheit formula: (°C × 9/5) + 32 = °F or in plain English, Multiple by 9, then divide by 5, then add 32. Convert Fahrenheit to Celsius Read Also: Java Program to Convert Inch to Kilometer and Kilometer to Inch #!/usr/bin/env python Fahrenheit = int(raw_input(“Enter a temperature in Fahrenheit: “)) Celsius = (Fahrenheit – 32) * 5.0/9.0 print “Temperature:”, Fahrenheit, “Fahrenheit = “, Celsius, ” C” Convert Celsius to Fahrenheit #!/usr/bin/env python Celsius = int(raw_input(“Enter a temperature in Celsius: “)) Fahrenheit = 9.0/5.0 * Celsius + 32 print “Temperature:”, Celsius, “Celsius = “, Fahrenheit, ” F” Read and understand the script.…
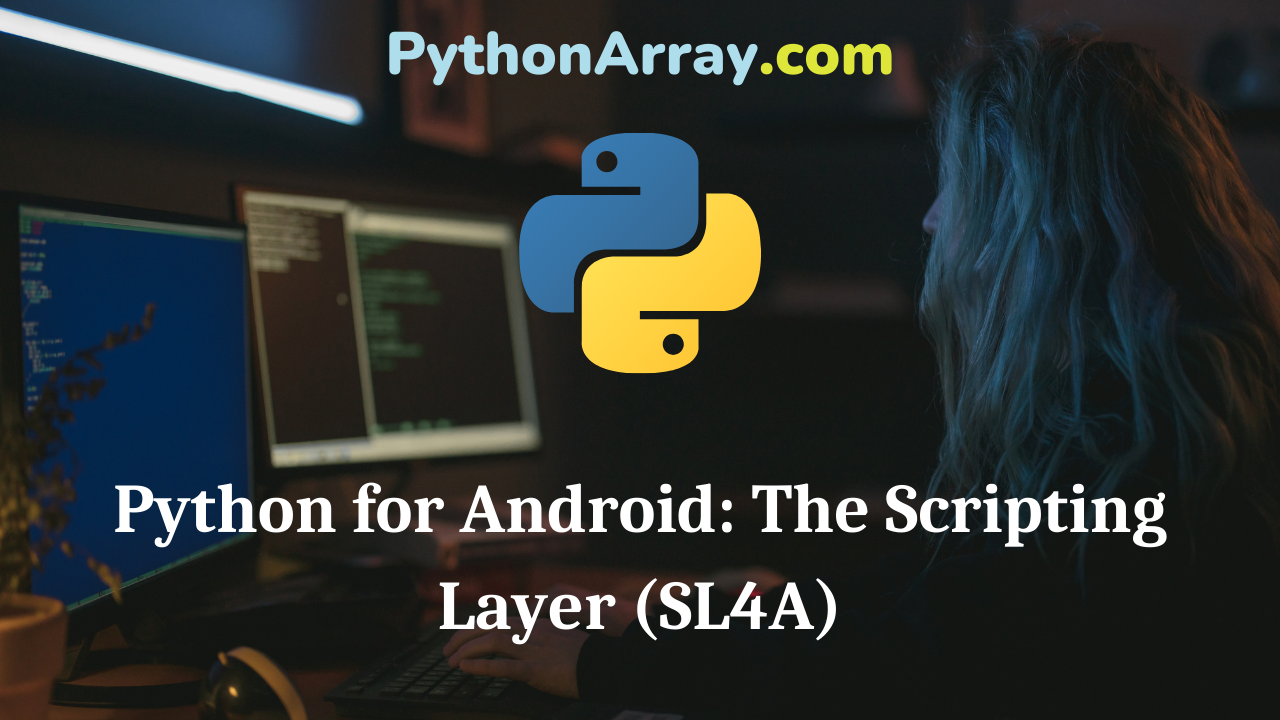
Python for Android: The Scripting Layer (SL4A)
The Scripting Layer for Android, SL4A, is an open source application that allows programs written in a range of interpreted languages to run on Android. It also provides a high level API that allows these programs to interact with the Android device, making it easy to do stuff like accessing sensor data, sending an SMS, rendering user interfaces and so on. It’s is really easy to install and it works on any stock Android device, so you don’t need to be root or anything like that. Currently, the Scripting Layer supports Python, Perl, Ruby, Lua, BeanShell, JavaScript and Tcl. It also provides access to the Android system shell, which is actually just a minimal Linux shell. Also Read: Java Program to Print Series 6 12 18 24 28 …N You can find out more about the SL4A project from their website. Python for Android: Using Webviews (SL4A) Python for Android: Android’s Native Dialogs (SL4A) Quick Tip: How to Print a File Path of a Module Why Android’s SL4A is Different There are a couple of other options for running Python on Android, and some are very good, but none offer the flexibility and features of the Scripting Layer. The alternatives really…
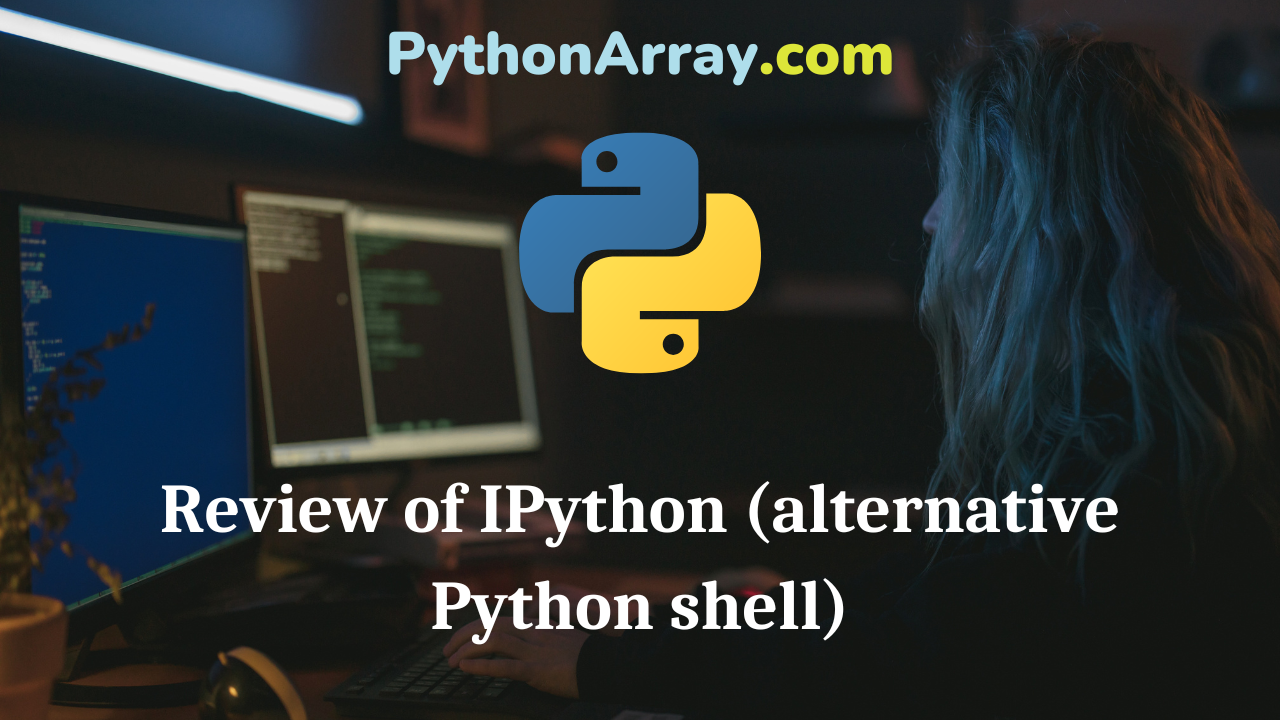
Review of IPython (alternative Python shell)
For an introduction to IPython, be sure to check out the article Introduction to IPython: An Enhanced Python Interpreter. One of the nice things about working in an interpreted language like Python is exploratory programming using the interactive Python shell. It lets you try things out quickly and easily without writing a script and then executing it, and you can easily inspect values as you go along. The shell has some conveniences, as well, including line-by-line history and a built-in pager for reading help. There are some limitations to the standard Python shell, though: you’ll end up using dir(x) frequently to inspect objects and find the method name you’re looking for. It’s also challenging to get the code you wrote from the shell into a convenient file with good formatting. Finally, if you’re used to the conveniences of an IDE, you’ll miss things like syntax highlighting, code completion, function signature assistance, and the like. Also, when testing GUI development using a toolkit other than TkInter, the GUI toolkit’s main loop blocks the interactive interpreter. There are some alternative shells for Python, however, that provide some of these extensions to the behavior of the basic interface. IPython, probably the oldest of these, offers…
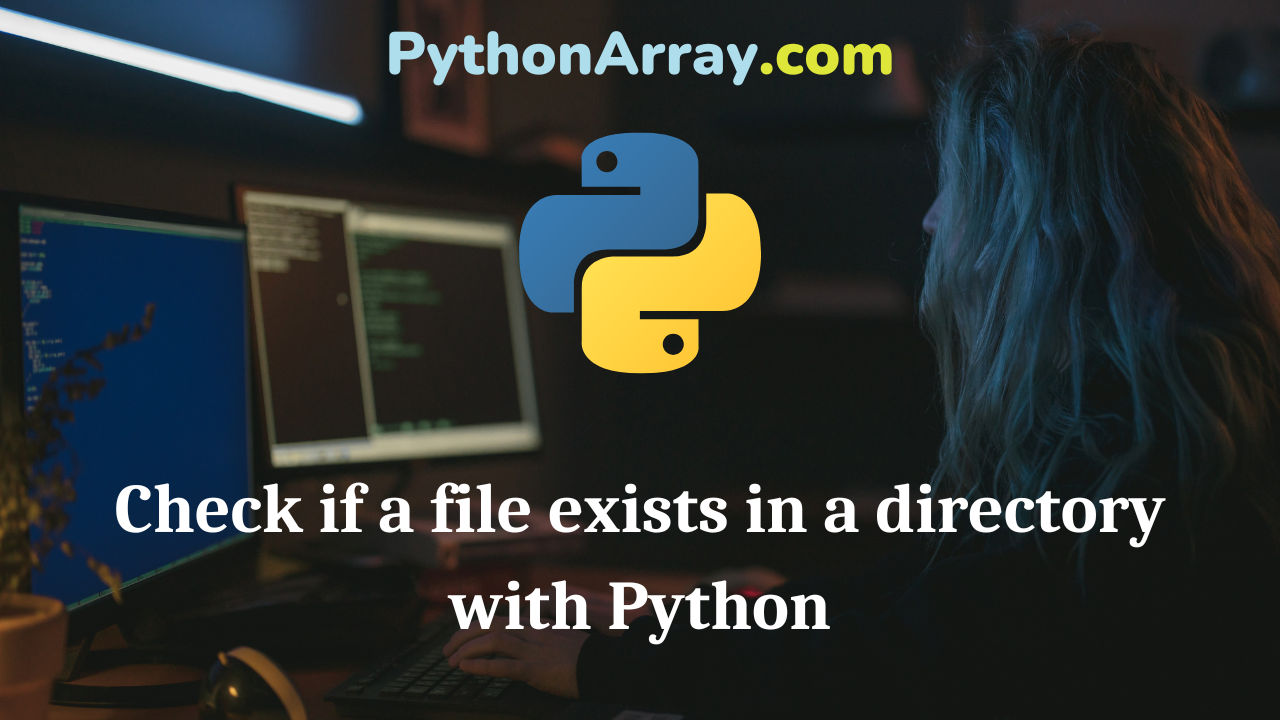
Check if a file exists in a directory with Python
With Python there are several methods which can be used to check if a file exists, in a certain directory. When checking if a file exists, often it is performed right before accessing (reading and/or writing) a file. Below we will go through each method of checking if a file exists (and whether it is accessible), and discuss some of the potential issues with each one. Also Read: Java Program to Check Sphenic Number 1. os.path.isfile(path) This function returns true if the given path is an existing, regular file. It follows symbolic links, therefore it’s possible for os.path.islink(path) to be true while os.path.isfile(path) is also true. This is a handy function to check if a file exists because it’s a simple one-liner. Unfortunately, the function only checks whether the specified path is a file, but does not guarantee that the user has access to it. It also only tells you that the file existed at the point in time you called the function. It is possible (although highly unlikely), that between the time you called this function, and when you access the file, it has been deleted or moved/renamed. For example, it may fail in the following scenario: >>> os.path.isfile(‘foo.txt’) True >> f…
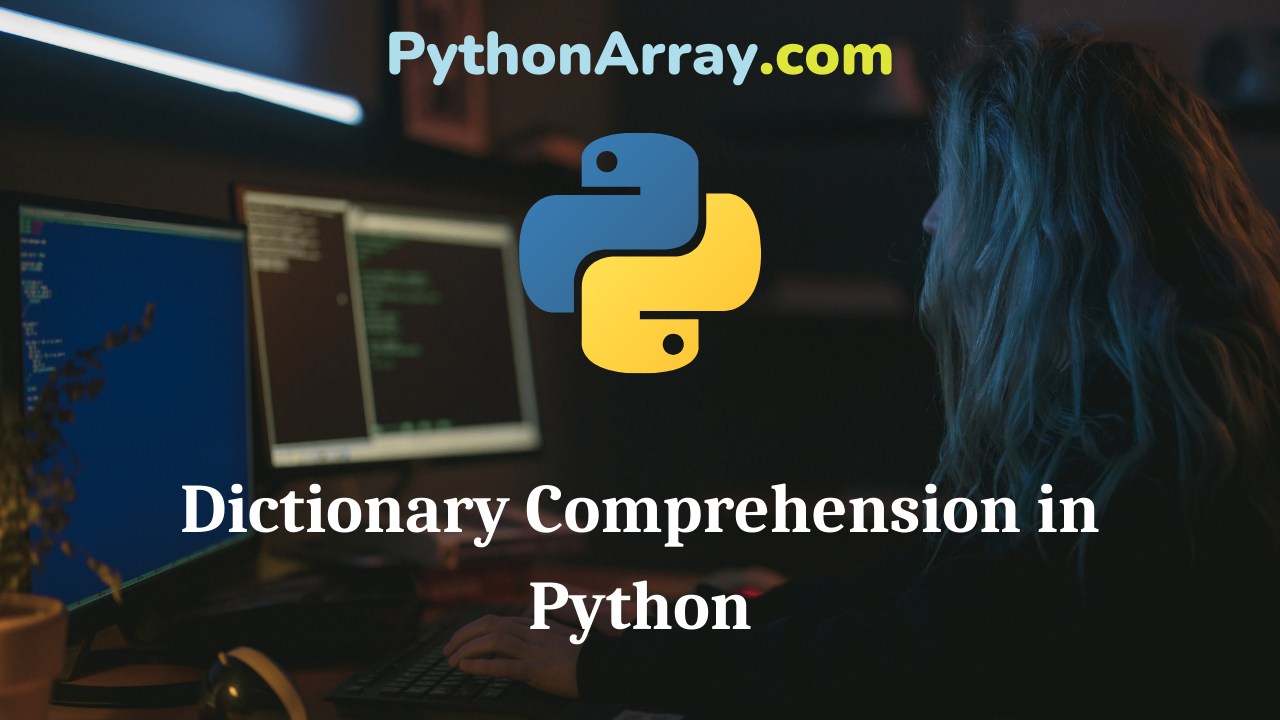
Dictionary Comprehension in Python
There may be situations while working with dictionaries in python where we need to create a new dictionary from another dictionary by including some items from the dictionary based on a condition or we want to create a new dictionary having keys and values following a specific condition. We may do it by manually checking the dictionary items one by one using a for loop and adding them to the new dictionary. In this tutorial, we will see how we can achieve the same result using a concise construct called dictionary comprehension in python. Also Read: Java Program to Print Stair Case Number Pattern What is dictionary comprehension? Just like list comprehension is a way to create lists from other lists, dictionary comprehension is a way to create a python dictionary from another dictionary or from any other iterable. We can create new dictionaries with the same elements as the original dictionary based on conditional statements or we can transform the items in the dictionary according to our needs. We can either modify the keys or the values or both key and value of items in the dictionary while creating a new dictionary using dictionary comprehension. Convert Dictionary Values List…
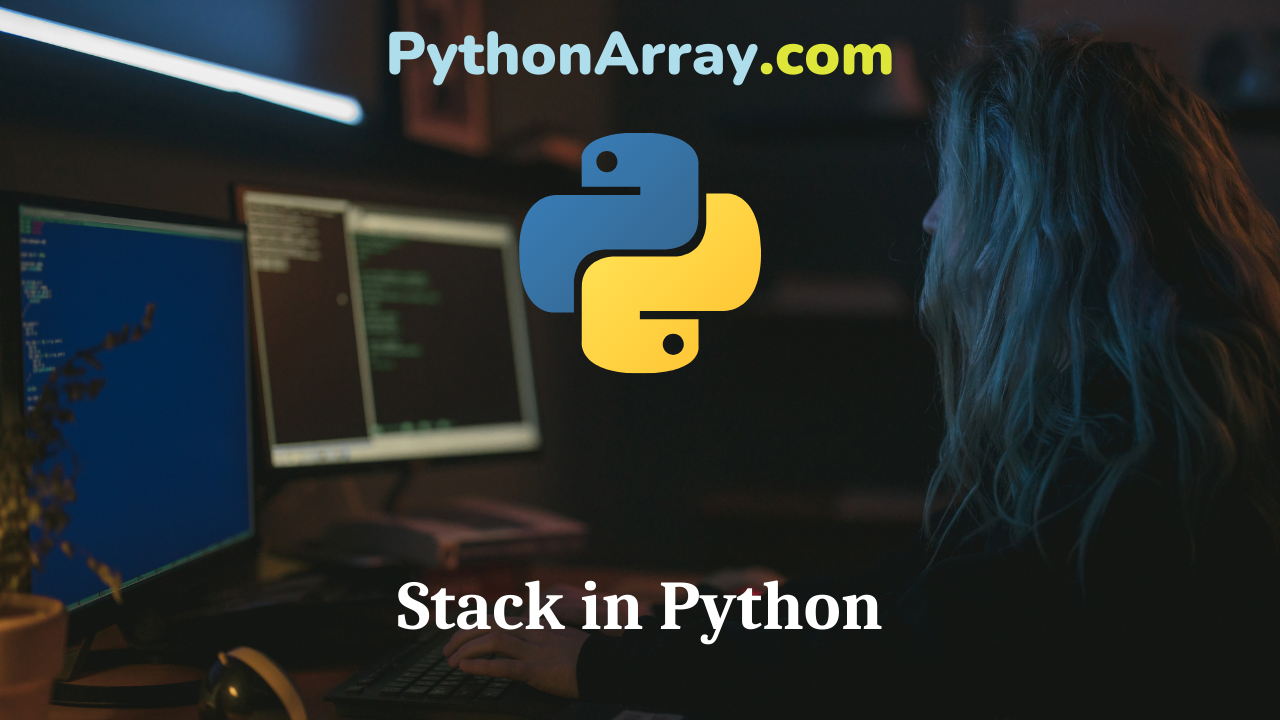
Stack in Python
In python, there are inbuilt data structures like list,tuple, set and dictionary but we may need some additional functionalities in python programs. For example, If we need a last in first out (LIFO) functionality in our program then none of the inbuilt data structures provide that functionality. We can use stack to implement last in first out functionality in python. In this article, we will study the concept behind stack data structure and implement it in python. Read Also: Python Program to Evaluate a Postfix Expression Using Stack What is a stack? A stack is a linear data structure in which we can only access or remove the element which was added last to it. In real life, we can take the example of a stack of plates where we can only take out the uppermost plate because it was added last. We can perform the following operations on a stack data structure in python. Insert an element(Push an element) Remove an element (Pop an element) Check if the stack is empty Check the topmost element in the stack Check the size of the stack In the following subsections, we will implement stack and all its and will implement them…