Python Programming – Special Functions in Python The Python language provides two special functions, which can be used while using inheritance. These functions and their description is given in Table 11.1. The programming example for these functions is also given in Code 11.7., the output of which apparently makes clear the use of these functions. Function Description issubclass(child, parent) Returns a boolean result; either true or false, true if the child is indeed a subclass of the superclass parent isinstance(obj, Class) Returns a boolean result; either true or false, true if obj is indeed an instance of Class or a subclass of Class Python Programming – Python Multiple Inheritance Python Programming – Python Multilevel Inheritance Python Programming – Editing Class Attributes Code: 11.7. Illustration of ininstance( ) and issubclass( ) functions. #Illustration of ininstance( ) and issubclass( ) functions class student: #base class ‘A student class’ class marks(student): #derived class ‘A marks class’ class result(marks): # new derived class A result class’ r1=result() r2=student() print(issubclass(result, marks)) print(issubclass(student, marks)) print(isinstance(rl, result)) print(isinstance(r2, result)) Output True False True False Python Tutorial
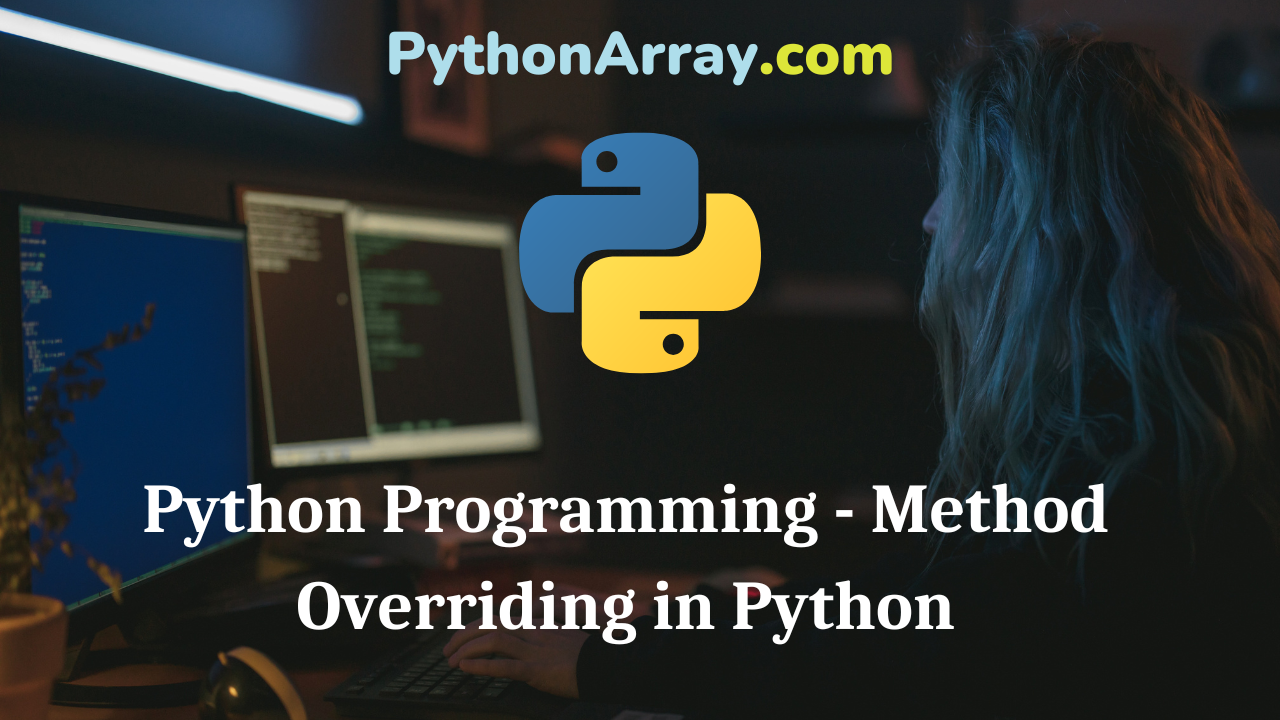
Python Programming – Method Overriding in Python
Python Programming – Method Overriding in Python Method overriding is one of the important concepts to consider while using inheritance in Python. As we see in the previous programming Code 11.1. for single inheritance that the __init__() method was defined both in the base class as well as derived class. When an object of a derived class is created then the __init_() method of derived class overrides that of the base class. That means, the __init__( ) of the derived class rectangle takes preference over the __init__( ) method of shape class. In order to overcome this issue, the __init__() method of the derived class is extended further to make a call to the __init__( ) method of base class. This is done by using shape, __init__() as shown in code 11.1. By invoking this way, a call to the__init__() method of shape class is made from the __init__() method of the derived class rectangle. Method overriding can also be resolved by using a built-in Python function super(). The super() function invokes the parent constructor method by itself without referring to the class name as mentioned in the call shape.__init__(). The super() function is used as follows: super().__init__() The programming…
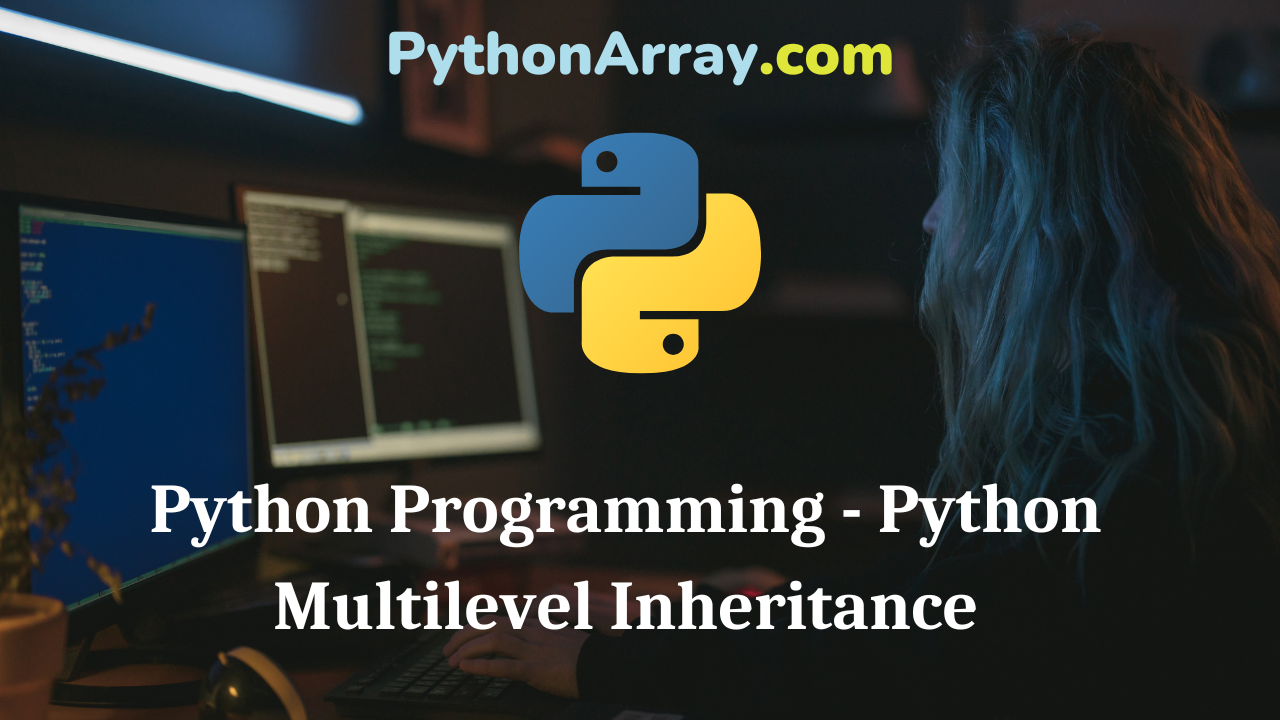
Python Programming – Python Multilevel Inheritance
Python Programming – Python Multilevel Inheritance In the concept of multilevel inheritance, we see that a derived class can be further inherited by a new derived class. In other words, the features of the base class and a derived class can be inherited by a new derived class. The programming illustration of multilevel inheritance is given in Code 11.3. It is almost similar to the program code given for multiple inheritances, where there were two base classes and one derived class. Herein, with multilevel inheritance, we create a base class student with similar data members and functions as mentioned in the previous example. Then, we derive marks class from the student class that means the marks class inherits the traits of the student class. Further, we create a new derived class result, which is inherited from the marks class. Eventually, we have three classes with two levels student->marks->result. Thus, the result class contains the traits of both the class’s student as well as marks. Alike, previous program the object of the result class is created, by which all the operations are performed like earlier, and the result is displayed. Python Programming – Python Multiple Inheritance Python Programming – Inheritance Python…
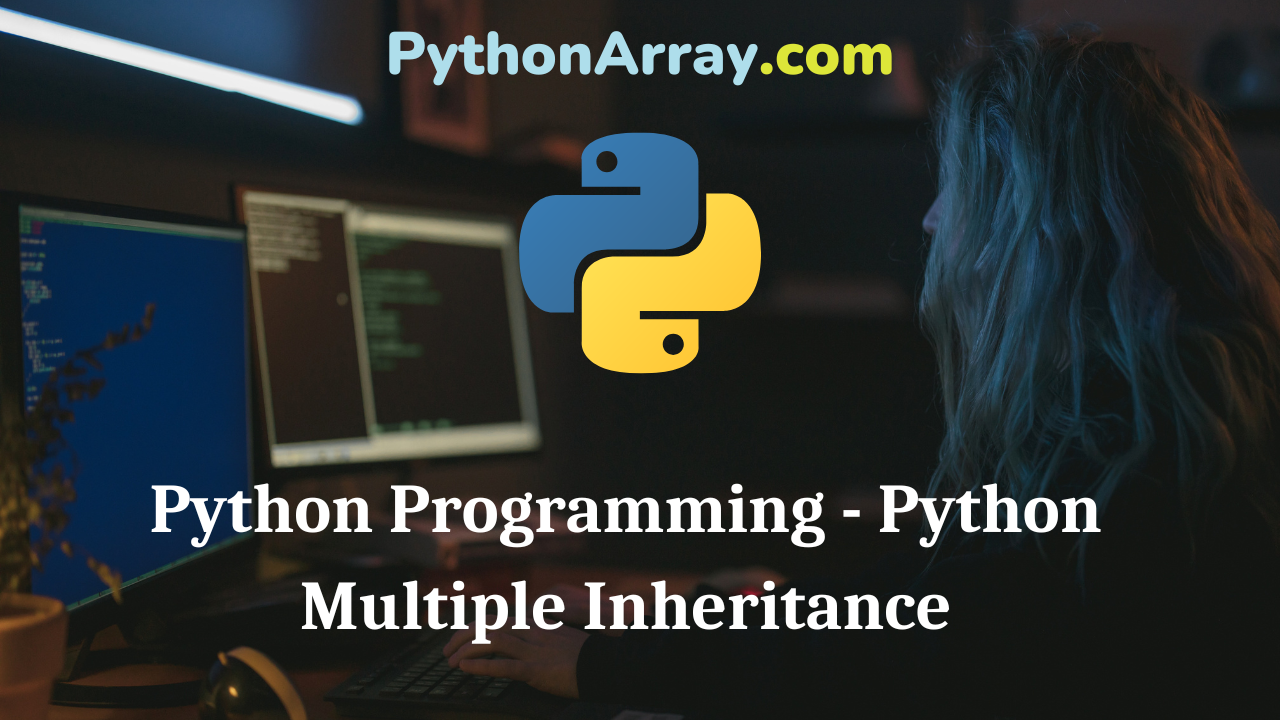
Python Programming – Python Multiple Inheritance
Python Programming – Python Multiple Inheritance Multiple inheritances refer to two or more base classes and one derived class. In this type of inheritance, the features of multiple classes can be inherited into the derived class. The programming example of multiple inheritances is given in Code 11.2. This program contains three classes, two base classes student and marks and one derived class result, which inherits the traits of both students and marks classes. The student class having two data members name and role of the student and a class function display, which displays the values of name and roll no. The other base class marks contain three data members ml, m2, and m3 representing marks of three subjects. It also contains a class function display_marks(), which displays the marks of all three subjects. Now, the third derived class result, which is derived from both the base classes student and marks contains two data members total_marks and perc representing percentage. It also contains a class function display_result() to display the total_marks and percentage of a student. In this program, the object of the derived class result is created as r1. It is to be noted here that by the creation of…
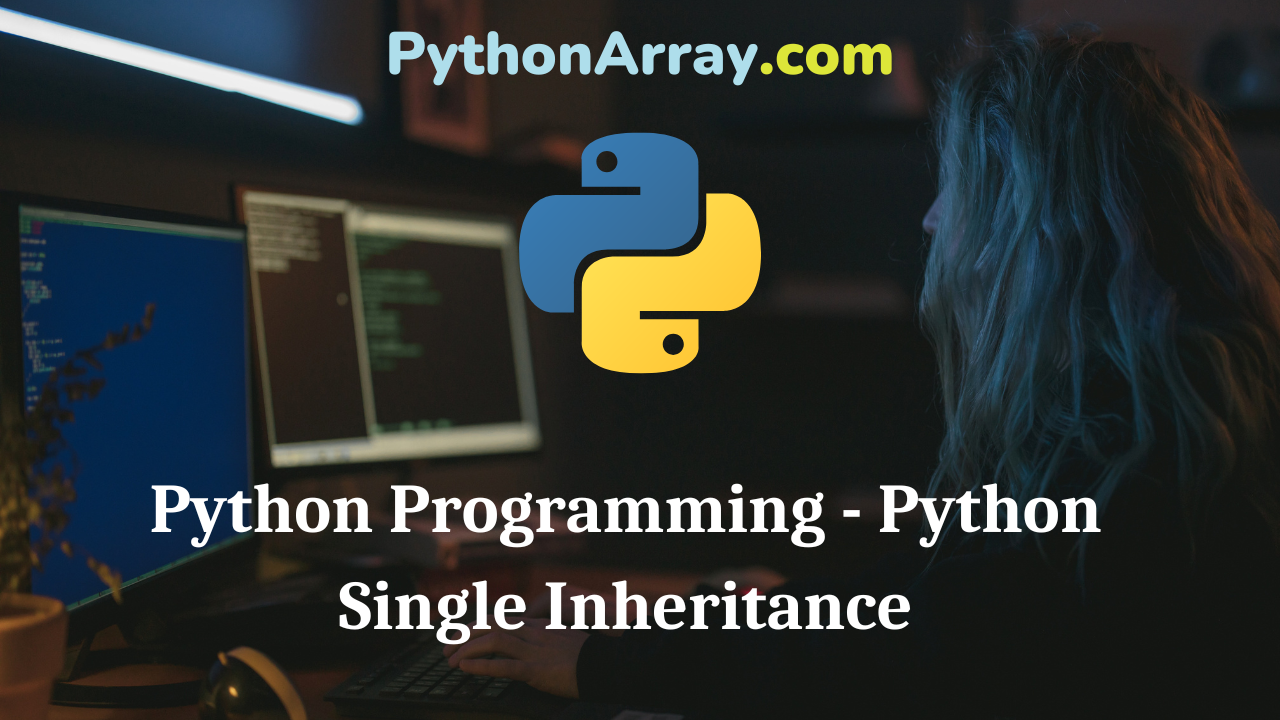
Python Programming – Python Single Inheritance
Python Programming – Python Single Inheritance A program to represent the concept of single inheritance is displayed in Code 11.1. In this program, we create a base class shape that contains two data members’ length and breadth as shown in the constructor method __int__( ) of the class shape. The base class shape contains one class function display, which displays the values of length and breadth. Then, we create a class rectangle which is inheriting the features of the base class shape. By deriving, the member’s length, breadth, and display() of base class shape will become the members of a derived class rectangle as well apart from its own members’ area and compute_area(). Now, we create the object r1 of the derived class rectangle and calls the functions display() and compute_area() with the object of the rectangle. It is to be noted here that, we have not created the object of base class shape and just by creating the object of the derived class rectangle, we can access the data members and functions of the base class. While the creation of object r1 of the derived class rectangle the constructor method __init__() of the derived class rectangle is invoked which…
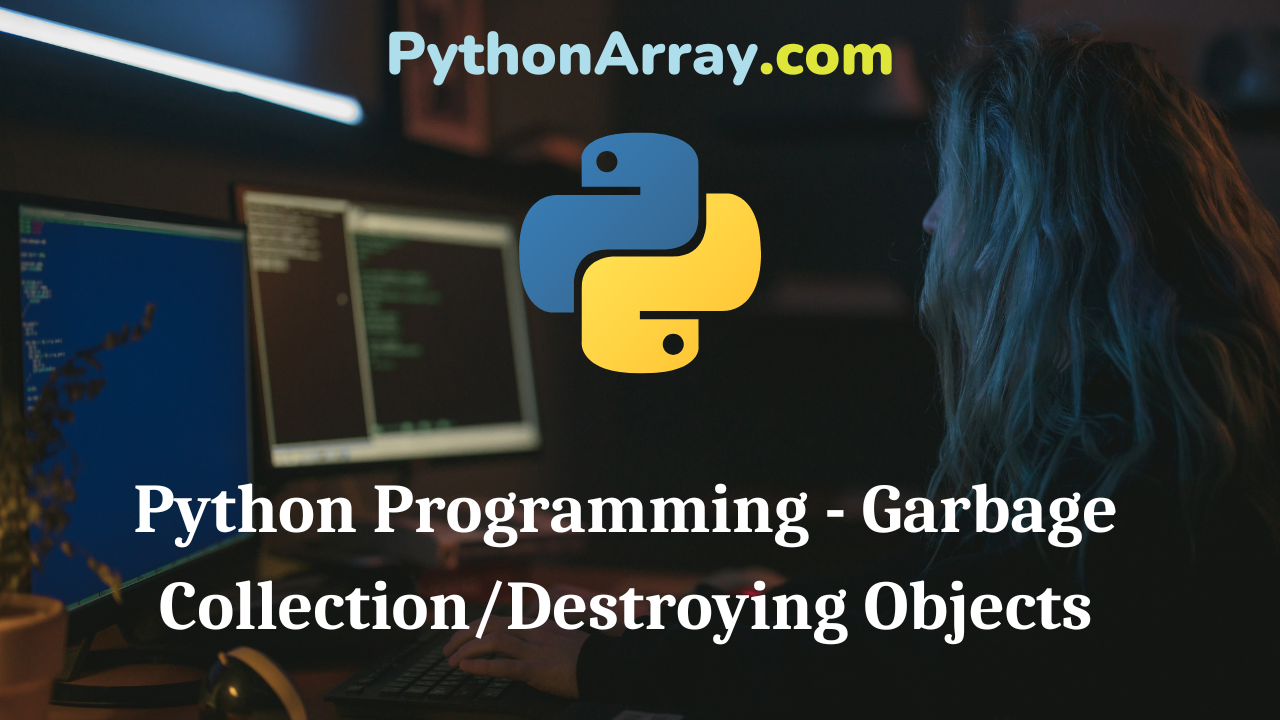
Python Programming – Garbage Collection/Destroying Objects
Python Programming – Garbage Collection/Destroying Objects Python facilitates the destruction of objects automatically when a certain object’s reference count reaches zero. That means Python deletes class instances automatically to free the memory space so that it can be utilized by some other program. The process of automatically and periodically cleaning the blocks of memory that is no longer required is called garbage collection. The garbage collector works automatically in the background to frees up any dereferenced space of memory. An object reference count increases when it is assigned a new name or place in a container and the object reference count decreases when it is deleted with the del statement as in the previous example Code 10.7. As the garbage collector works automatically, we do not know in the front end when an object has been destroyed by the garbage collector. However, Python provides a special method _del_(), called a destructor, that is called when the instance is about to be destroyed. The syntax of destructor method _del_() is given as follows: def del (self): Python Programming – Classes and Objects Python Programming – Exception Handling Python Programming – Array Creation We see from the syntax that it contains self…
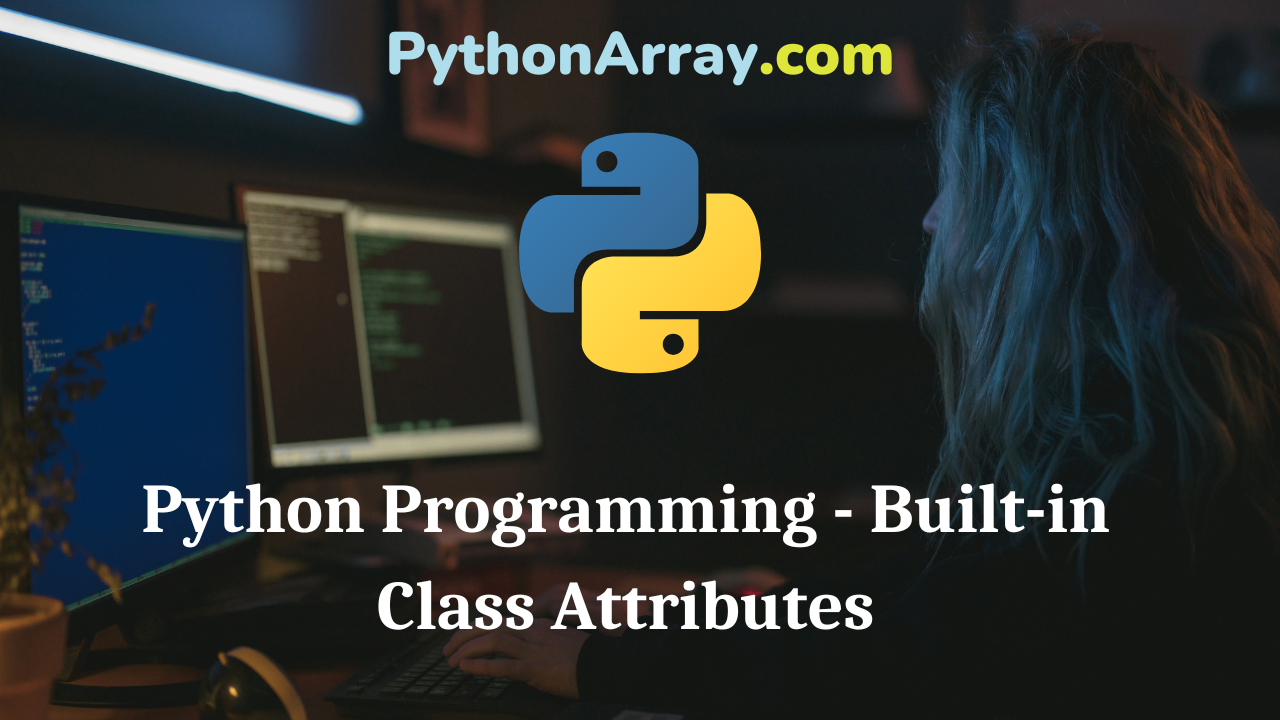
Python Programming – Built-in Class Attributes
Python Programming – Built-in Class Attributes We have seen in the previous sections that how to create a class and the attributes of a class. As we know that class is a user-defined data type. The programmer can create his own data type in the form of a class, which follows the concept of object-oriented programing structures. While creating a class, we specify our own attributes. However, certain attributes intrinsically associated with the class, when it is created. The list of built-in attributes is given in Table 10.2. with the description of each. The programming example for the same is given in Code 10.9. As we can see from the program that the first built-in attribute diet gives the information about the class variables itemCount and total_bill and class functions display them(), init () along with other details. The doc attribute gives the information about the document string which is “An inventory class” for this code. Subsequently, the named module provides the class name which is ‘inventory’. the module gives the main module. In the end, the bases attribute gives the “(<class ‘object’>,)” since there is no base for this class. It is to be noted that the class built-in…
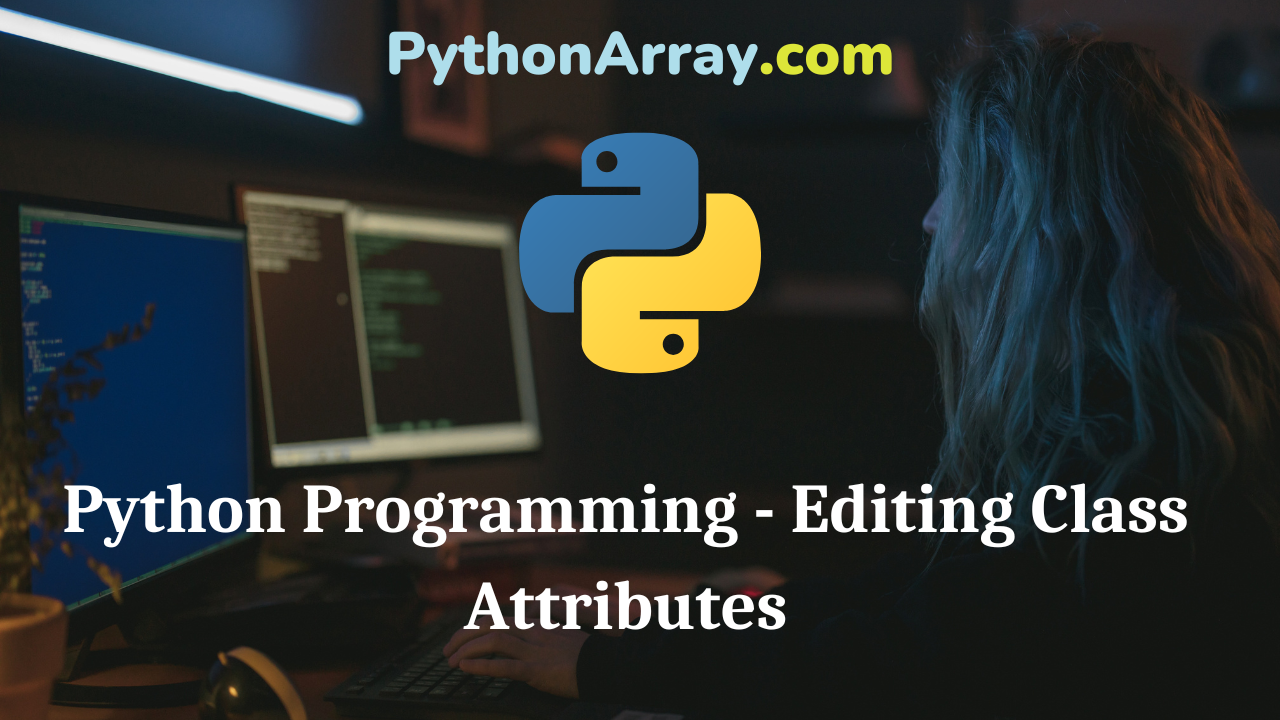
Python Programming – Editing Class Attributes
Python Programming – Editing Class Attributes Unlike C++ and Java in Python, the programmer can add, delete or modify class attributes. It is a very simple procedure, which can be performed by using the class object name with the class attribute by using the dot (.) operator. For example, in the above program given in Code 10.6, where inventory class is created with attributes item, price, quantity, and the total bill. Any of these attributes can be modified or deleted. Moreover, one can also add an additional attribute. The programming example for the same is given in Code 10.7. In this program, we add a new attribute code by using the class object II .code=l 11, then we modify the value of the “price” attribute by assigning the new value as Il.price=29 then we display the values of new attributes by calling the function display them(), which provides the output as shown in the output section of Code 10.7. Finally, the deletion of any of the attributes can be performed by del keyword. It is just a keyword to delete the reference of a certain attribute of a class. In the code given in 10.7., we delete the code attribute…
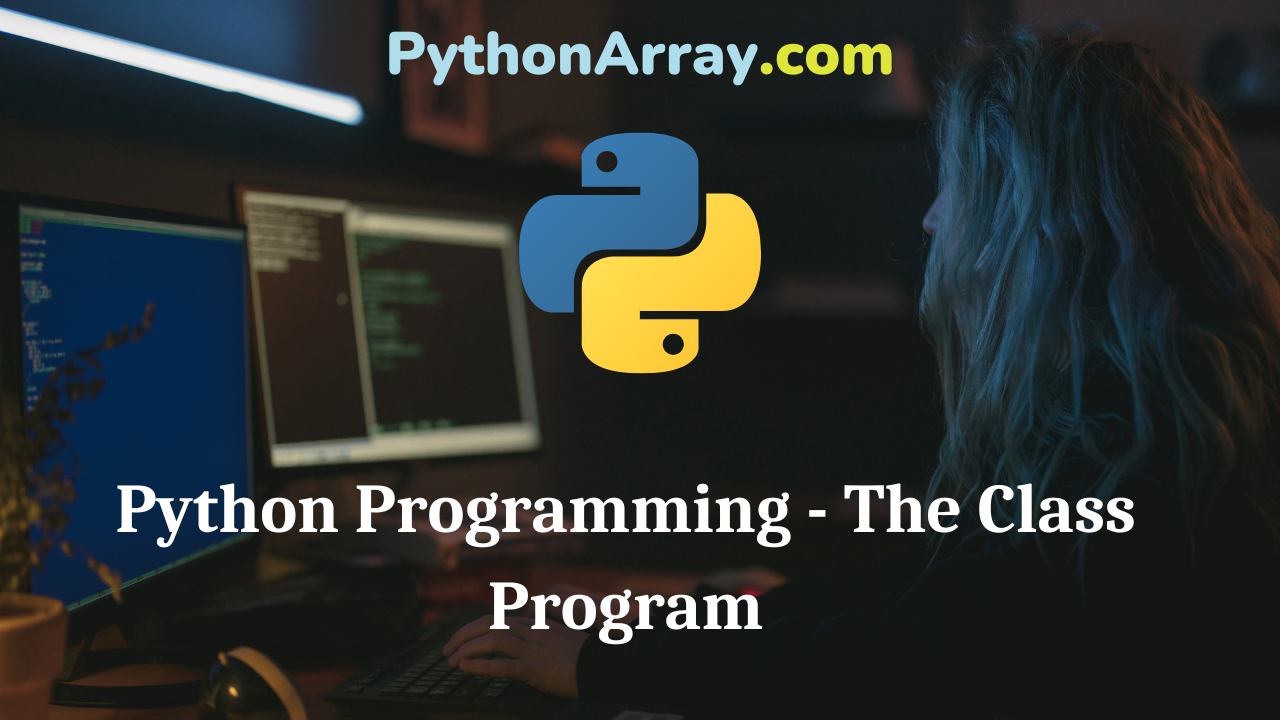
Python Programming – The Class Program
Python Programming – The Class Program The complete program representing the entire concept of creating classes and objects is given in Code 10.4. Here we see that a class variable student is created and two data members i.e., name and roll no are created. The values to name and roll are assigned through the constructor method __init__( ) in Python. It contains three arguments. The first is the self, which is mandatory in Python for every function (method) to be used within the class. The other two arguments are name and roll no. Two class methods display count() and display student() is also defined to display the total number of students and information of the students. Then, two objects soul and stu2 and created with the values of name and roll no, which are passed as arguments to the constructor function. Subsequently, the display student() method is called through stu1 and stu2 objects by using the dot(.) operator. The value of the common class variable stuCount is displayed or accessed by using the class name with the variable name student along with dot operator. The output of this code explains it all. Python Programming – Classes and Objects Python Programming…
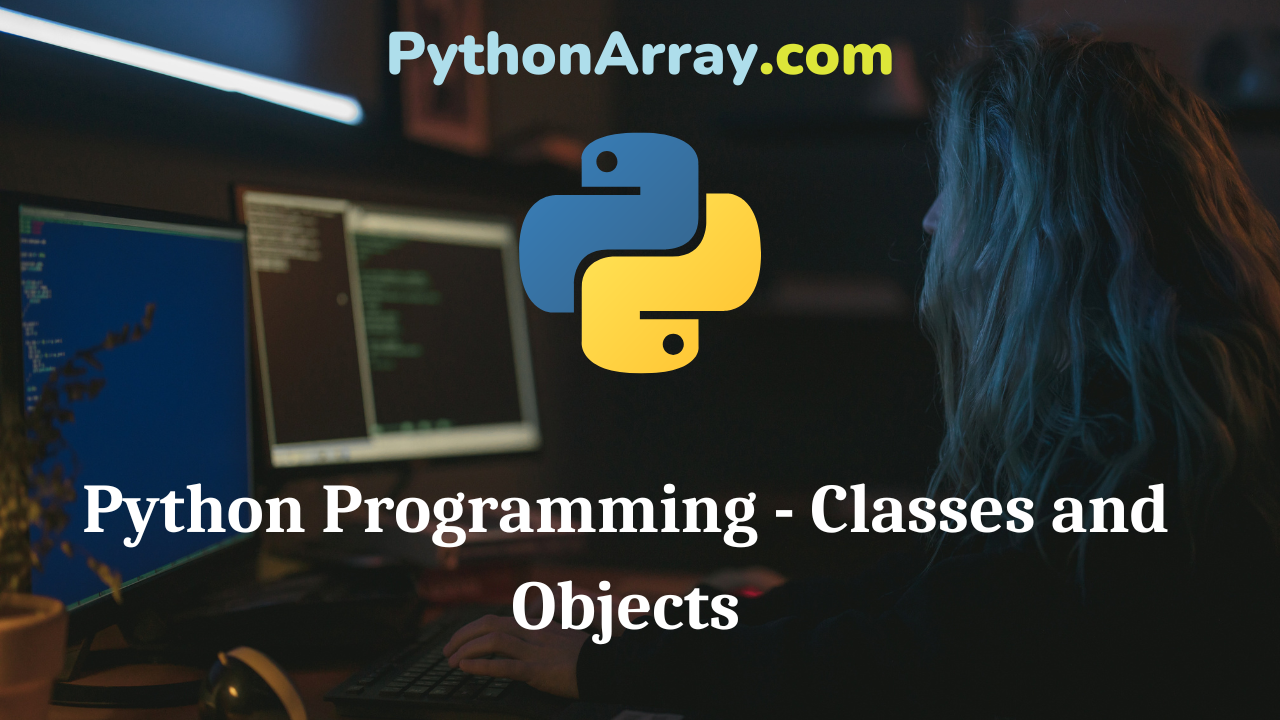
Python Programming – Classes and Objects
Python Programming – Classes and Objects Object-oriented is a term used to describe the object-oriented approach for building software. In an object-oriented approach, the data is treated as the most important element and it cannot flow freely around the system. This approach binds the data and the methods that will manipulate the data closely and prevents the data from being modified inadvertently. The object-oriented programming exhibits the following properties: Encapsulation Data hiding and abstraction Inheritance Polymorphism (overloading/overriding) Dynamic binding Here we will discuss each of these properties in brief. Encapsulation: The wrapping of data and functions into a single unit is known as encapsulation. Data hiding and abstraction: It refers to represent the necessary features without including the background particulars. Inheritance: It refers to the property by which objects of one class inherit the properties of objects of another class. Polymorphism: It refers to the ability to take more than one form. Here poly means many and morphism means forms. For example, the addition of two numbers will result in a sum however, the addition of two strings results in a concatenation of strings. That means to use single operator + for different purposes refers to operator overloading. Python Tutorial…