Python Programming – Python Modules In the previous chapter, we have learned about the creation of Python user-defined functions. We have seen that a large problem can be divided into small fragments to solve each of them separately using functions. In this chapter, we study modules, which are an extension to the user-defined functions. As in C/C++, and Java header files or packages are used to make use of built-in functions, in Python modules are used to utilize built-in Python functions. Apart from that, similar to C/C++/Java, where users can create header files or packages to use them similar to built-in ones, Python has the feature to create modules for further use. Need for Module In the interpreter-based Python programming language, all the definitions, variables, and functions written by the programmer get lost, when he quits the interpreter. However, another way to save the program code is to write the program in a script form in some editor such as notepad, which we have followed so far in this book. For large programs, it is a good practice to divide it into several files for better understanding and maintenance. Moreover, sometimes a similar function is used in multiple programs, so…
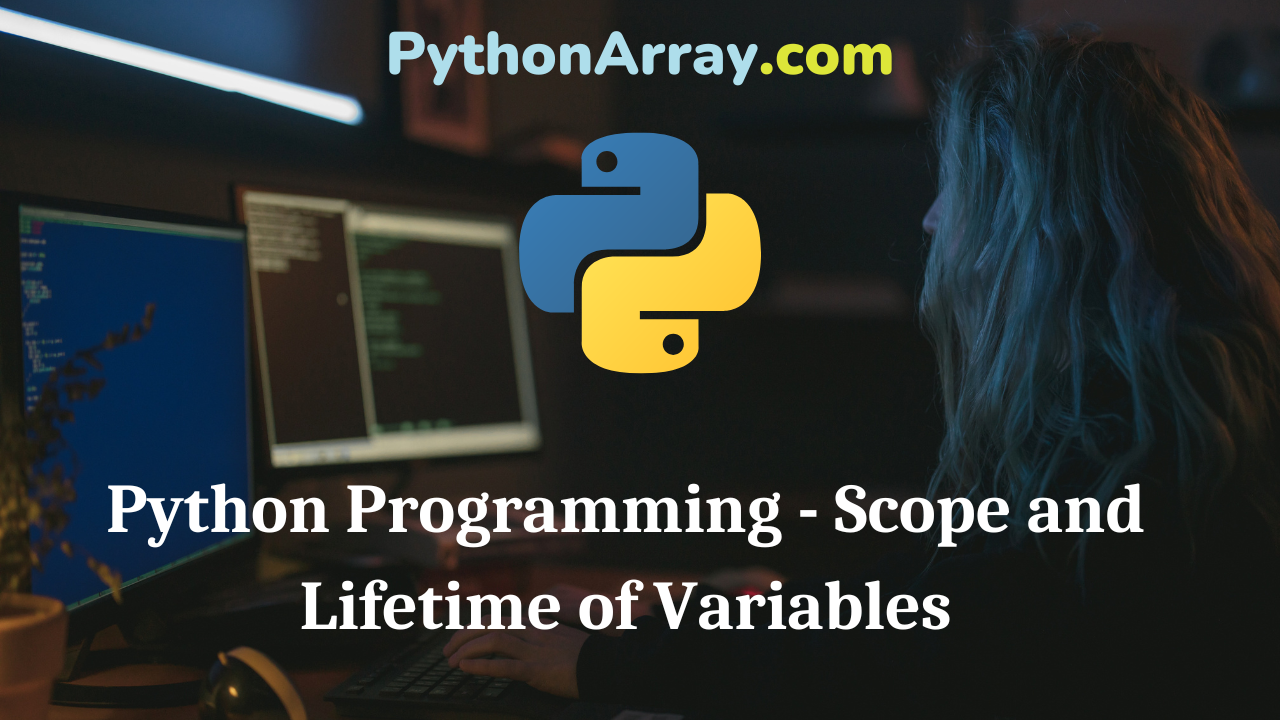
Python Programming – Scope and Lifetime of Variables
Python Programming – Scope and Lifetime of Variables The scope of a variable can either be local or global. It is a region of the program, where it is recognized. If a variable is defined outside all the functions of a program then its scope is considered to be global. As the value of that variable can be accessed by any of the functions. Such a variable is termed a global variable. On the other hand, the local variable exhibits a limited scope. Any variable defined inside a function is referred to as a local variable as it can be accessed only within that function. The lifetime of a variable is the duration or period for its existence in the memory. The lifetime of a local variable is as long as that function executes. They are destroyed or removed from the memory once the function is returned back to the function call. On the other hand, the lifetime of a global variable is as long as the whole program executes. The program is given in Code: 6.15. illustrates the concept of local and global variables. In this program, we see that a variable value is defined globally with a value…
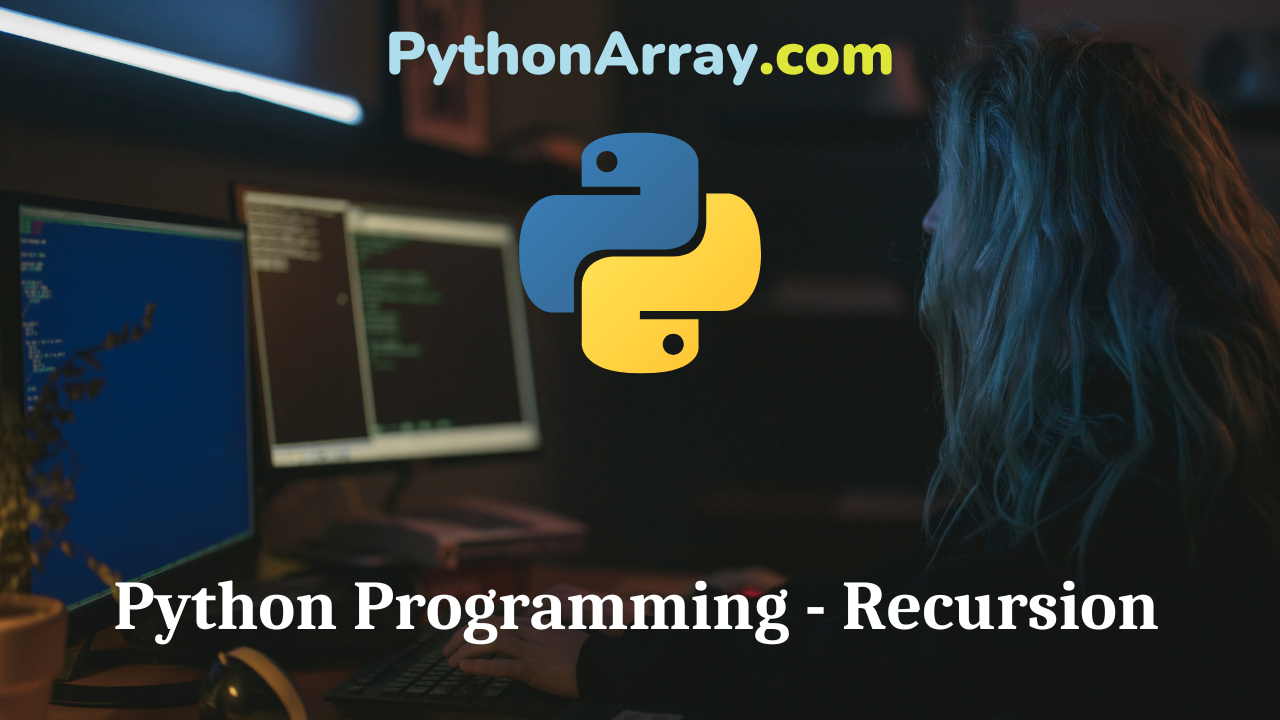
Python Programming – Recursion
Python Programming – Recursion Alike C/C++, a function can call itself, rather than from any other function. This concept is termed recursion. Recursive functions act like loops that they iterate within the function to perform some operation. The programmer must consider some points while using recursion, which are Recursion can be used for any function involving loops. While developing programs using recursion, the programmer must follow some guidelines, such as There must be a key variable, which will be responsible for the termination of recursion, To determine the base value, which the key variable has to meet to reach the termination, To make sure the key variable must approach the base value in every recursive call. To make the recursive function terminate when the key variable reaches the base value. The programming example to compute the factorial of a given number using recursion is presented in Code: 6.14. In this program, initially, the function call fact(num) is made with the number input by the user. In the function definition, recursive calls are made to function fact() with the value of n is decrementing by 1 in every recursive call. This process continues until the value of n becomes 1. Then,…
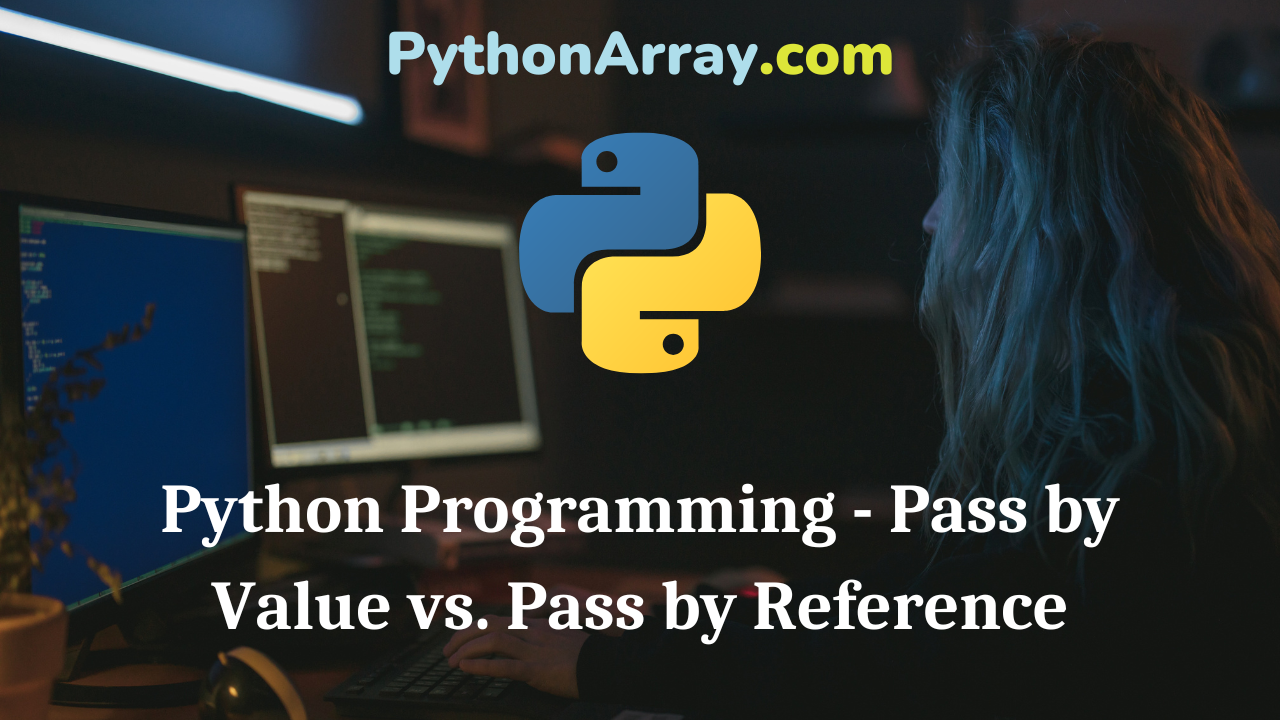
Python Programming – Pass by Value vs. Pass by Reference
Python Programming – Pass by Value vs. Pass by Reference As we know that the two-way communication between function caller and function definition is achieved through arguments, which we pass by the function call. In Python, language arguments can be passed by value and by object reference. Pass by Value This concept can be understood by considering the program for passing arguments by value in Code: 6.12. It is perceived from the output of the program that a list object is created with values 1, 2, 3 and it is passed as an argument to the function update( ). In the function definition of update, we see that the list values are modified to 100, 200, 300 and the result is printed, which displays the new values. However, when the function update() returns back and the value of the list is printed out to be the old ones, i.e., 1, 2, 3, and not the updated ones. This concept is known as a pass-by value, which signifies that if any modifications are made to the values in the function definition then it does not make any effect on the arguments of the caller function. Python Programming – Python User Defined…
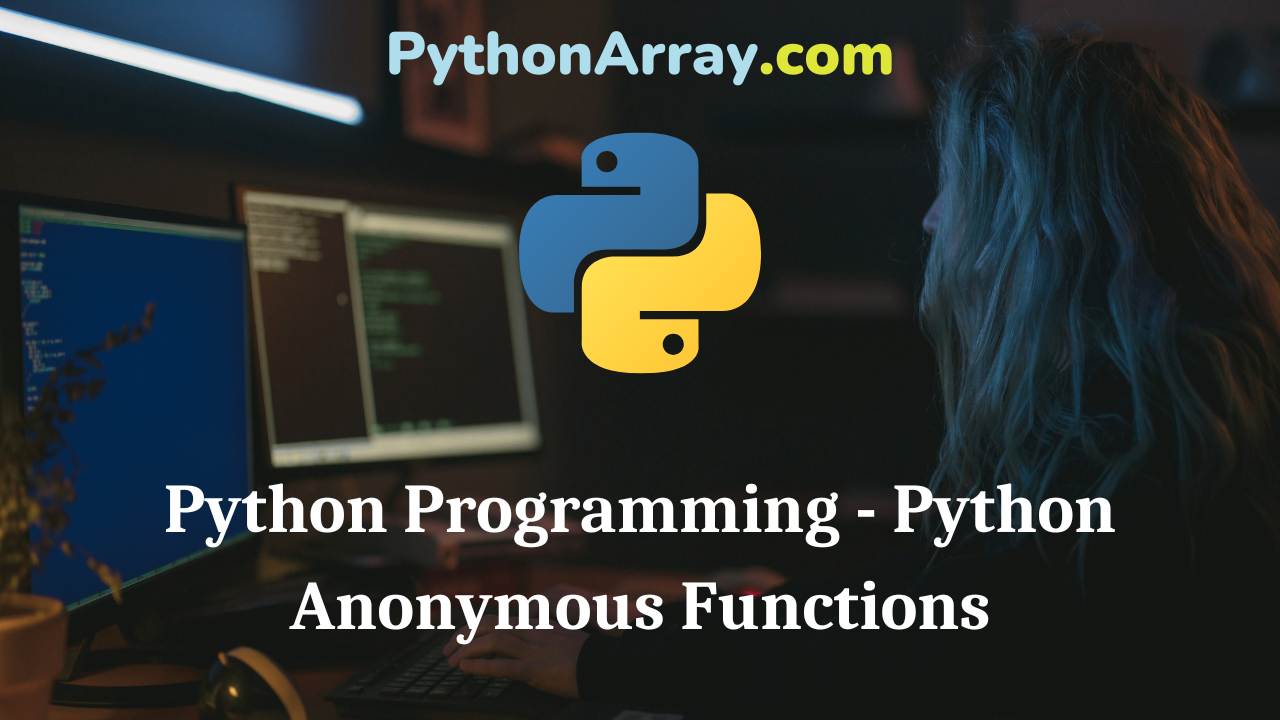
Python Programming – Python Anonymous Functions
Python Programming – Python Anonymous Functions Python supports the development of an additional type of function definition known as an anonymous function. These functions are termed anonymous because they do not follow the standard method by using the def keyword and anonymous functions are not bound to a name. These are created at runtime, using the construct known as lambda. The syntax of lambda is as follows: lambda arg1, arg2,…, argn: expression Characteristics of Lambda Form (Anonymous Function) • lambda form can take multiple arguments as shown in the syntax and returns only one value computed through the expression. • It does not contain multiple lines of statement blocks as in standard Python functions. • Since, an expression is required in lambda form, the direct call to print() function can not be made in lambda form of anonymous function. • As no additional statements can be written in lambda form, it has only a local namespace that means it can use only those variables that are passed as arguments to it or which are in the global scope. • The lambda form (anonymous functions) can not be considered as C/C++ inline functions, as they contain only a single line of…
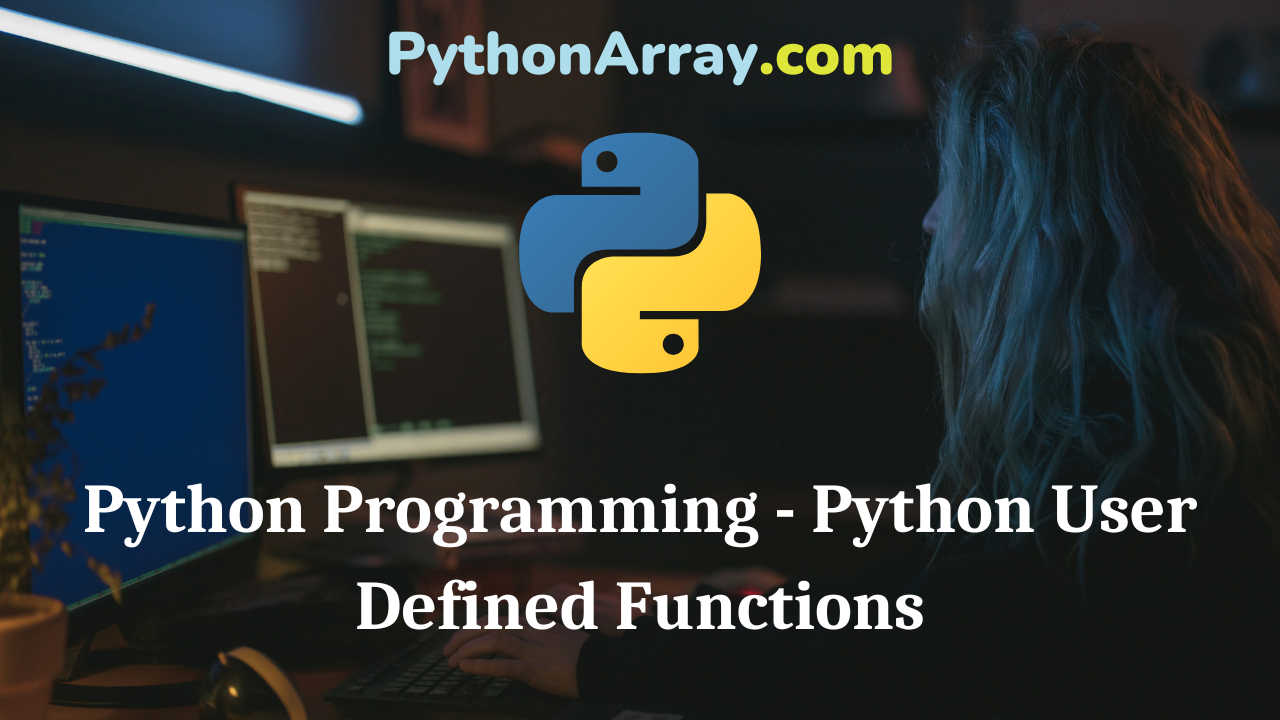
Python Programming – Python User Defined Functions
Python Programming – Python User Defined Functions As we have seen in the previous section that Python contains a rich set of built-in functions. However, the programmer can develop their own functions depending upon their requirement and such functions are termed user-defined functions. In formal language, functions that are defined by us to perform certain specific operations or tasks are termed as user-defined functions. Formally, the user-defined functions contain two parts: Function Definition: contains the actual code for the operation to be performed. Function Call: where the call to the function definition is made to perform a specific operation. Function Definition (Defining a Function in Python) Unlike C/C++/Java, the format of Python user-defined functions is different. The syntax of user-defined functions is given below: def function_name(list of parameters): “docstring” statement(s) return(parameter) Python Programming – Types Of Functions Python Programming – Python Functions Python Programming – Functions The above syntax of function definition has the following components: 1. The keyword def symbolizes the start of the function header. 2. A function name to uniquely identify it. Function naming follows similar rules that are used for writing identifiers as described in Chapter 2. 3. List of parameters also called a list of…
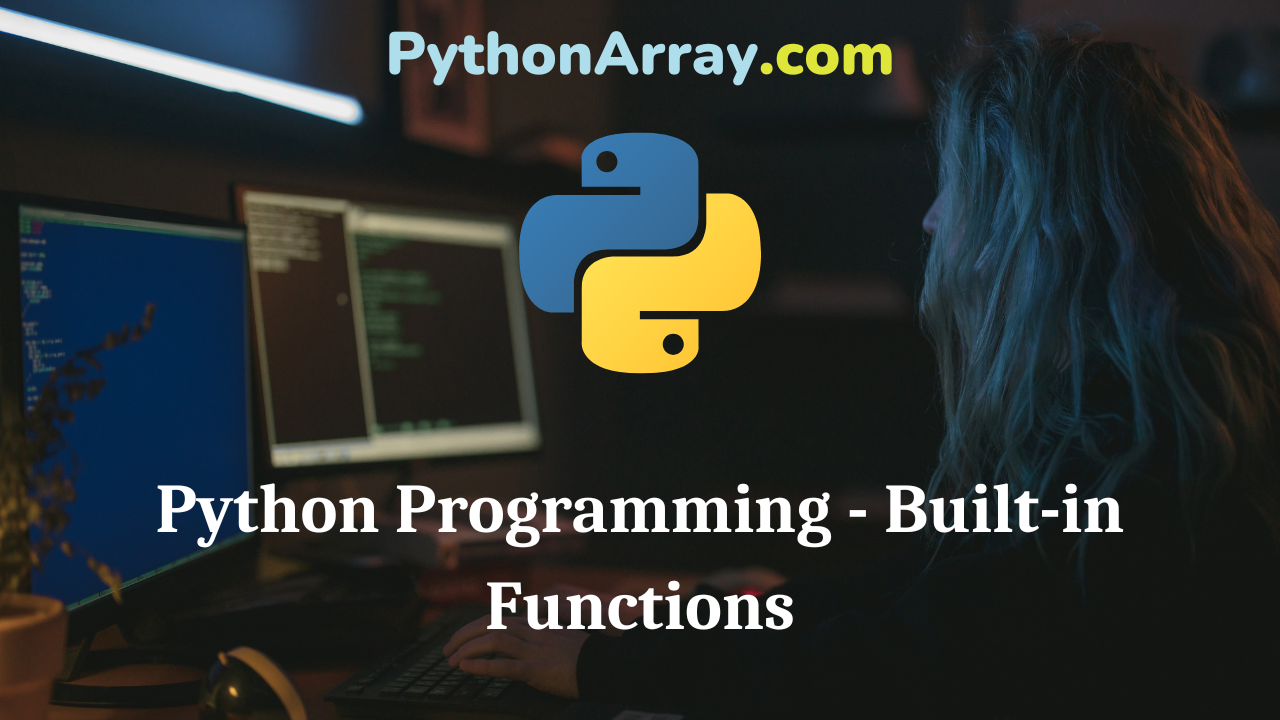
Python Programming – Built-in Functions
Python Programming – Built-in Functions The functions are already provided by Python are called built-in functions. The programmer can use built-in functions readily without any additional effort to code that function. The functions, which we have already used in previous chapters such as print( ), abs ( ), random ( ), range ( ), print ( ), input ( ), eval ( ), etc. In Python version 3.5.1. 68 built-in functions are available (which may vary depending on the different versions). The built-in functions are listed in Table 6.1., with the description of each. Python Programming – Python Sets Convert Dictionary Values List Python | How to Convert Dictionary Values to a List in Python Python Programming – String Functions Built-in Function Description abs() Returns the absolute value of a number. all() Returns True if all elements of the iterable are true (or if the iterable is empty). any() Returns True if any element of the iterable is true. If the iterable is empty, return False. ascii() Returns a string containing a printable representation of an object, but escape the nonASCII characters. bin() Converts an integer number to a binary string. bool() Converts a value to a Boolean. Bytearray( )…
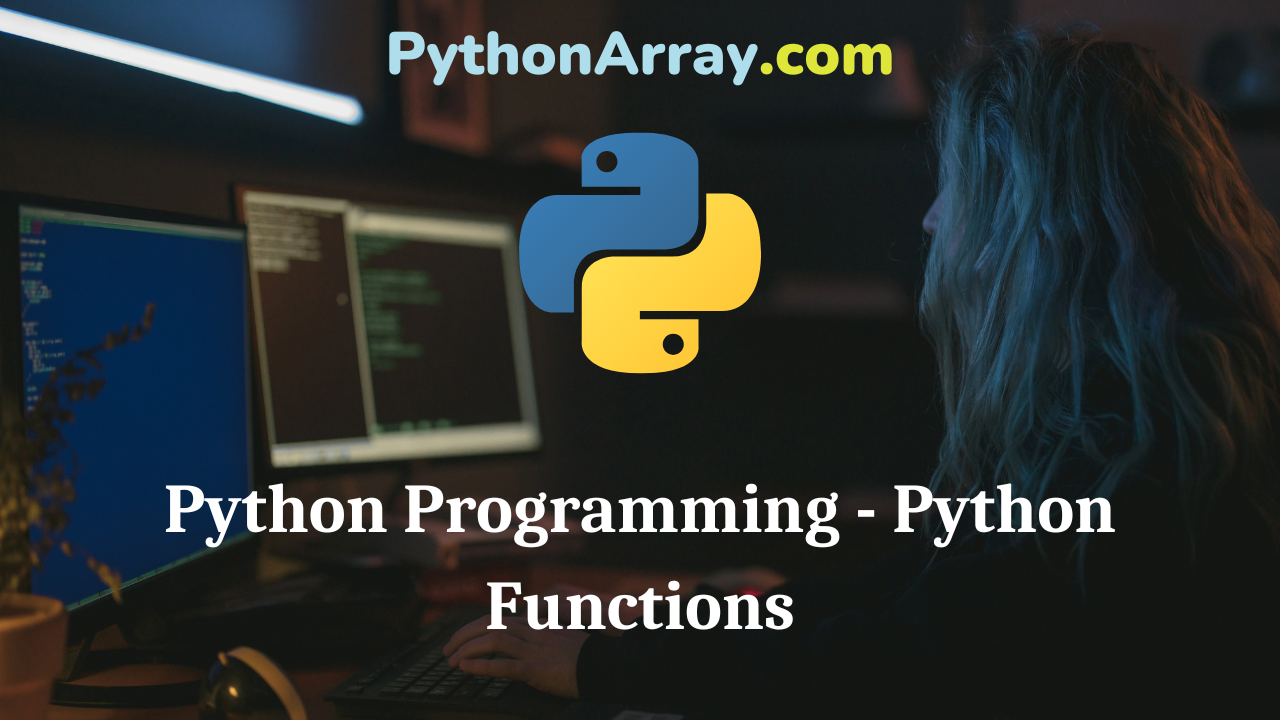
Python Programming – Python Functions
Python Programming – Python Functions In programming, sometimes a similar code has to be repeated at many places in a program. For which, the programmer needs to write the same set of instructions several times. For example, while computing a binomial coefficient, the factorial needs to be computed several times. It would waste the programmer’s time and effort to write the same code again and again. This activity not only increases the size of the program but also leads to typographical errors. To overcome this issue, the Python programming language is provided with a feature called subprograms or functions. A subprogram or function is a name given to a set of instructions that can be called by another program or a subprogram. This technique simplifies the programming process to a great extent because a single set of instructions in the form of a subprogram is being used for all instances of computational requirements with only changes in the supplied data. Python Functions In the Python programming language, a function is a self-contained group of statements, which are intended to perform a specific task. Functions break the program into smaller modules, which perform a particular operation and are easy to understand.…
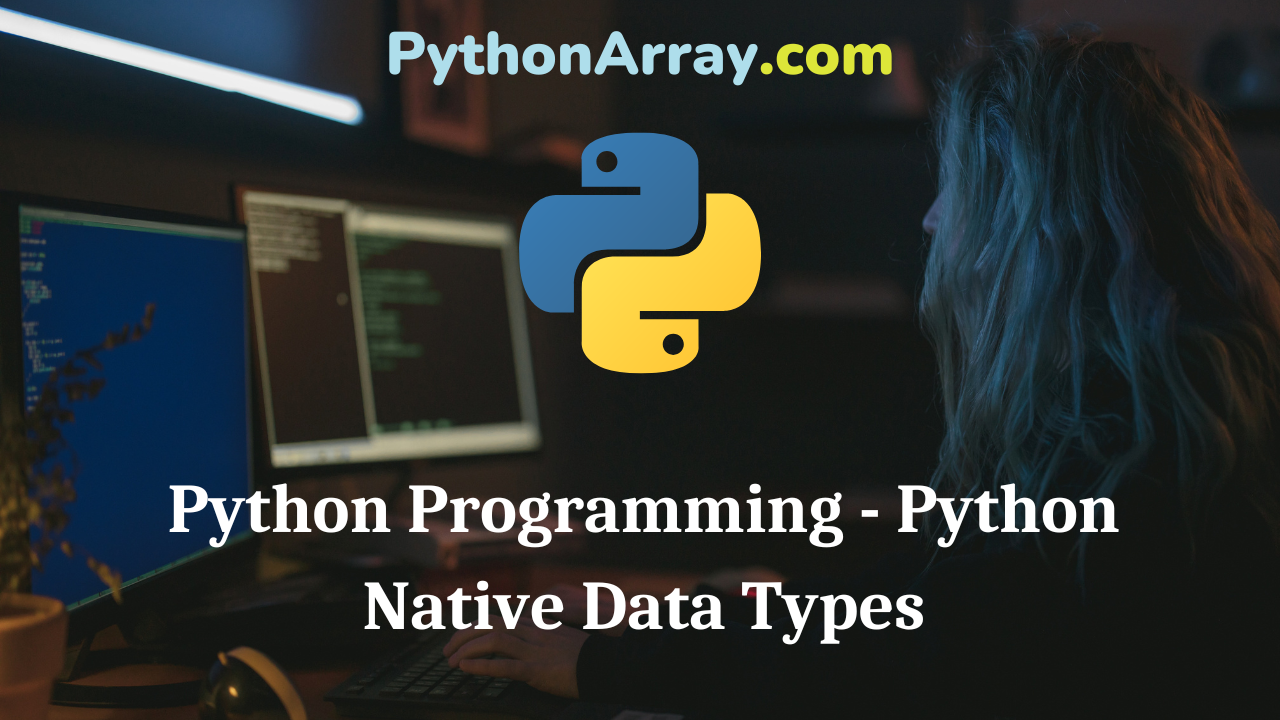
Python Programming – Python Native Data Types
Python Programming – Python Native Data Types As described in Chapter 2, Python language supports different data types to handle various sorts of data. Here in this chapter, we describe the following Python native data types in detail. Number: represents numeric data to perform mathematical operations. String: represents text characters, special symbols, or alphanumeric data. List: represents sequential data that the programmer wishes to sort, merge, etc. Tuple: represents sequential data with a little difference from the list. Set: is used for performing set operations such as intersection, difference, etc with multiple values. Dictionary: represents a collection of data that associate a unique key with each value. Python Programming – Standard Data Types Python Programming – Introduction To Numpy Python Programming – Dictionaries Summary In this chapter, we learned the Python native data types thoroughly. The Python language contains number, string, list, tuple, set, and dictionary, data types. These are the different ways to store data in Python. The number and string data types store numeric and character data respectively. Whereas, list, tuple, set, and dictionary store heterogeneous data sequentially. However, the set and dictionary represent an unordered representation of data. All the methods and functions associated with these data…
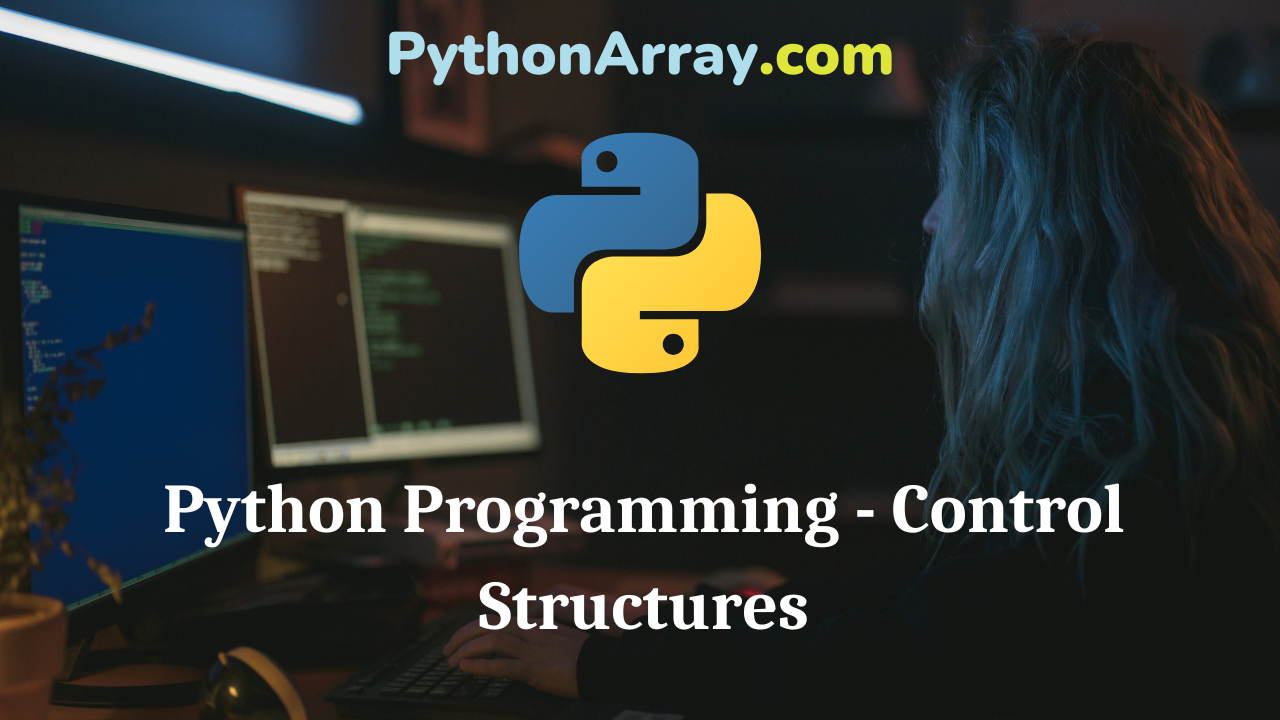
Python Programming – Control Structures
Python Programming – Control Structures Generally, a program is executed in a sequence normally from top to bottom. The statements get executed one after the other as the interpreter transfers the control from the current statement to the next statement as soon as the execution of the current statement gets over. However, in some situations, the sequential flow does not work. The control needs to move to some other location in the program depending upon certain conditions or there may be a requirement to execute a set of statements repetitively. Therefore, this chapter intends to emphasize control structures that are used to alter the order of execution of a program. In this chapter, we will learn three types of control structures decision making, looping, and controlling. Python Programming – Conditional and Iterative Statements Python Programming – Decision Making Python Programming – Conditional Statements Summary In this chapter, we have learned all the control structures provided by Python. language. The decision-making statement if, if-else, elif, nested if are elaborated thoroughly. The most likely used looping constructs while and for are discussed with appropriate programming examples of each. The new features of Python while loop with else, for loop with else, and…