Python Programming – Python Directory Methods In the previous section, we have described various Python directory handling methods with the programming illustration of each. However, Python contains a large number of methods to work with directories. All these methods are to be used with the os module. That means the os module needs to be imported for using any of the methods associated with directories. The list of methods to be used with directories is given in Table 9.6. with the description of each method. Python Programming – Directories in Python Python Tutorial for Beginners | Learn Python Programming Basics Python Programming – Python Modules Method Description os.access(path, mode) Uses the real uid/gid to test for access to path. os.chdir(path) Changes the current working directory to path os.chflags(path, flags) Sets the flags of path to the numeric flags. os.chmod(path, mode) Changes the mode of path to the numeric mode. os.chown(path, uid,gid) Changes the owner and group id of path to the numeric uid and gid. os.chroot(path) Changes the root directory of the current process to path. os.close(fd) Closes file descriptor fd os.closerange(fd_low, fd_high) Closes all file descriptors from fd_low (inclusive) to fd_high (exclusive), ignoring errors. os.dup(fd) Returns a duplicate of…
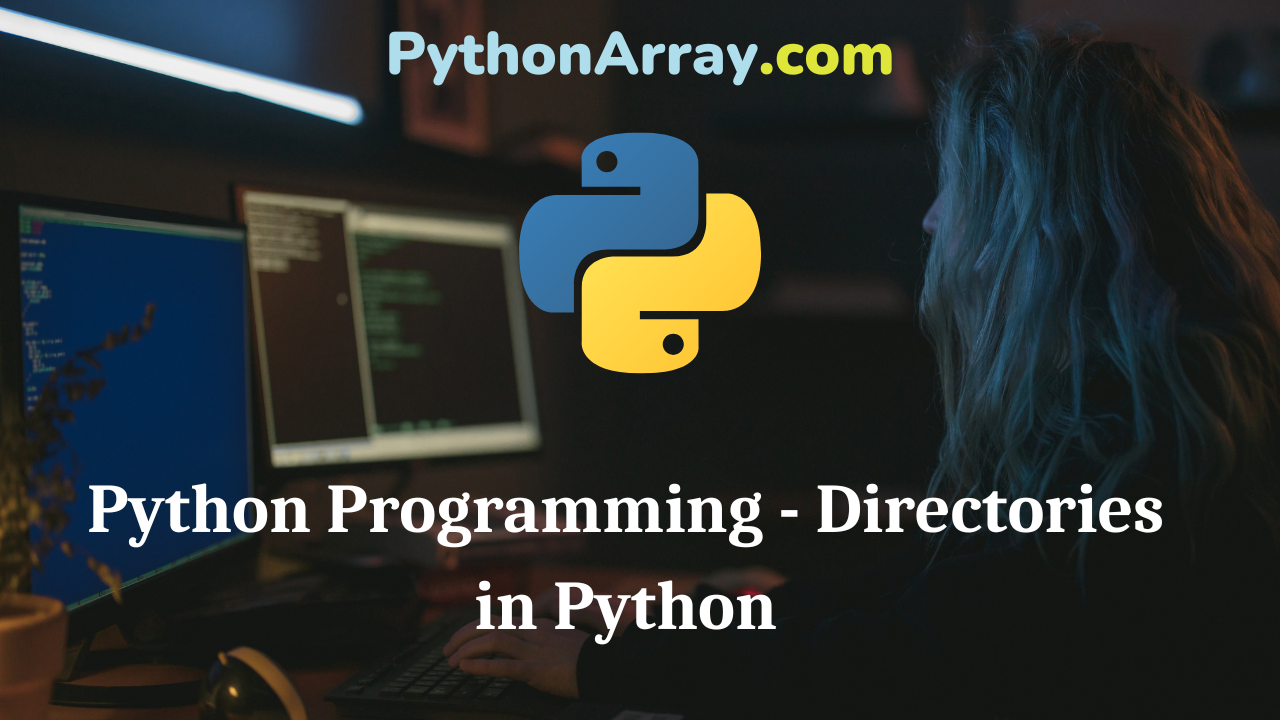
Python Programming – Directories in Python
Python Programming – Directories in Python So far, we have discussed the creation of files in Python and various „ operations upon them. However, we can also work on directories in Python. This is achieved by making use of the os module and various methods in it. Here, we discuss all the methods associated with the directories in Python. mkdir( ) method The mkdir() method is used to create directories in the current directory. This method contains only one argument that is the name of the directory to be created. The syntax for using the mkdir is given as follows os.mkdir(“new_directory_name”) The programming example of mkdir() is presented in Code 9.15. After the execution of this program, a new directory data file is created in the current folder. Code: 9.15. Illustration of mkdir( ) method. # Illustration of creating a directory Import os os.mkdir(‘datafiles’) Python Programming – Renaming and Deleting Files Python Programming – Python Packages Python Programming – Functions chdir( ) method The chdir() nlethod is used to change the current directory. It also takes one argument for moving from one directory to another and making it the current directory. The general syntax of chdir is given as follows:…
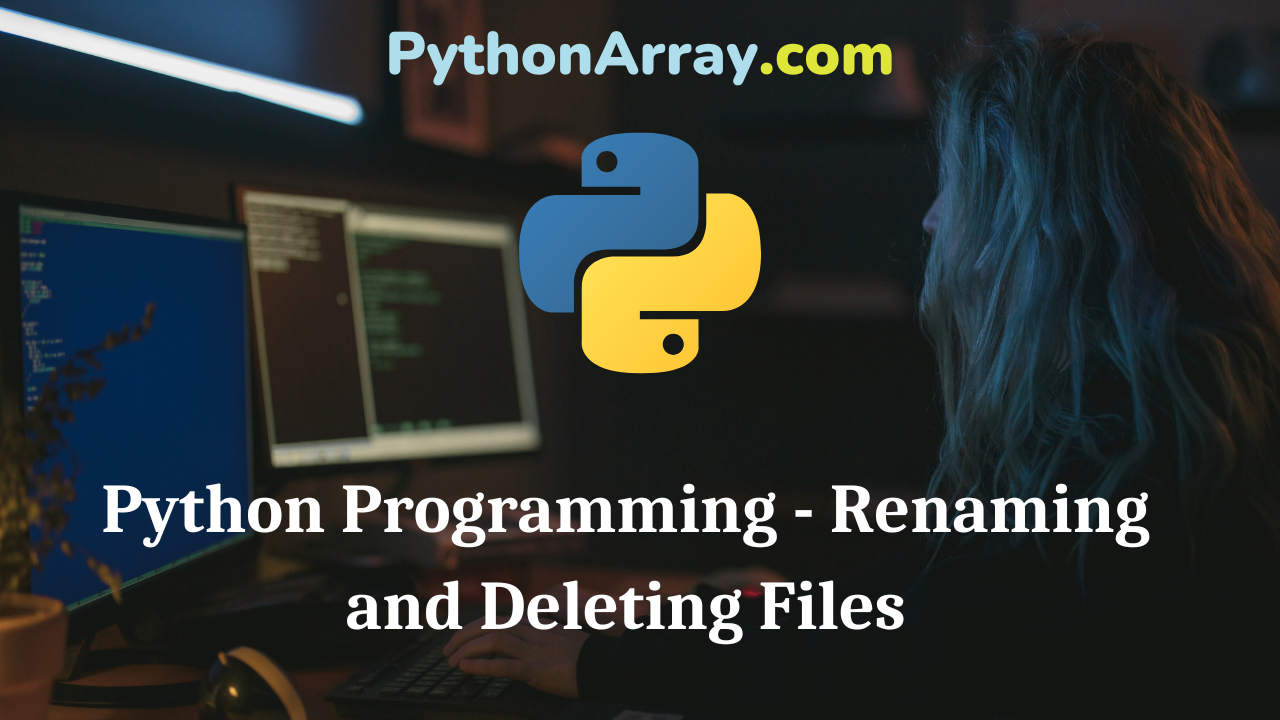
Python Programming – Renaming and Deleting Files
Python Programming – Renaming and Deleting Files Python language provides special methods for renaming and deleting a file. These methods are available through the Python os module. For using these methods, the programmer needs to import the os module. Both of these methods are very easy to use and described below: rename( ) method The rename( ) method takes two arguments. The syntax of rename( ) method is given as follows: os.rename(old_file_name, new_file_name) The programing example for using the rename( ) is given in Code 9.13. We see that the data.txt file already exists. By using the rename( ) method, it is renamed to info.txt. The programmer can see in the current directory that the file has been renamed. Code: 9.13. Illustration of renameO method. #Illustration of renaming a file Import os os.rename(‘data.txt’, ‘info.txt’) remove( ) method The remove( ) method is used to delete the file. It has only one argument, which is the file name to be deleted. The syntax of remove( ) is given as follows: os.remove(file_name) The programming example for using the remove( ) method is given in Code 9.14. This program removes the file from the current directory. After the execution of this program, the…
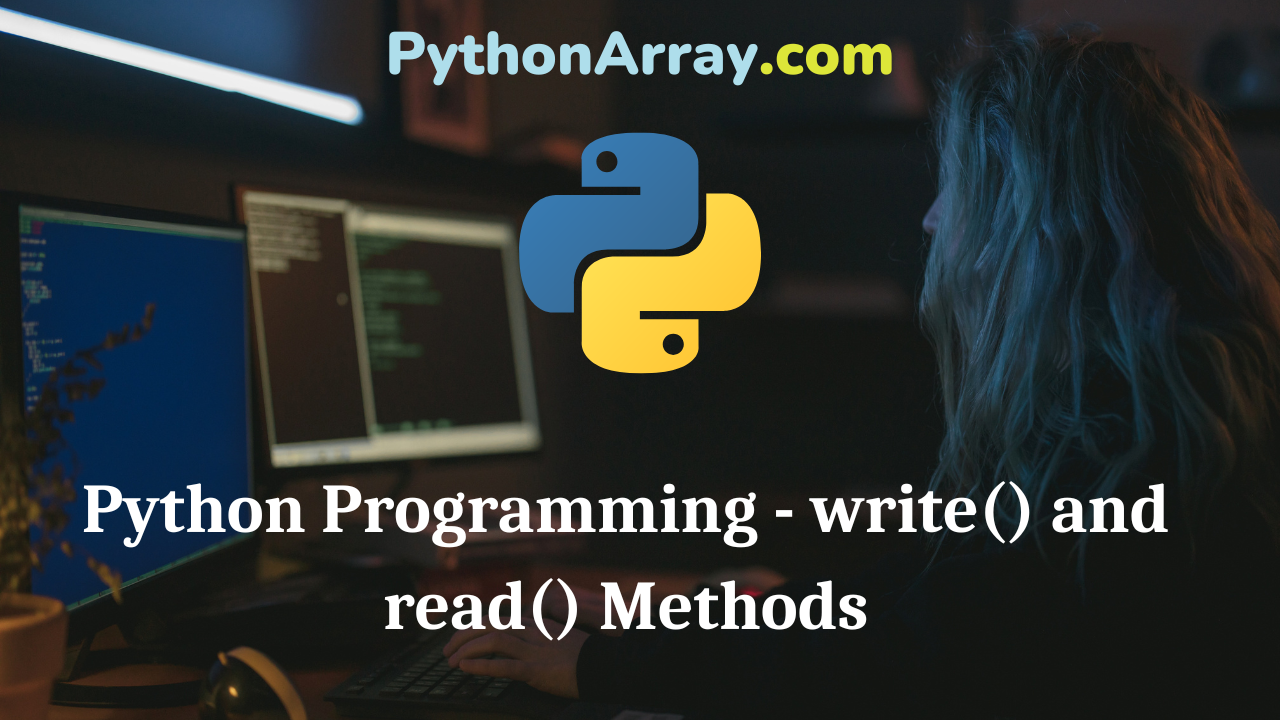
Python Programming – write() and read() Methods
Python Programming – write() and read() Methods Alike, C, C++, and Java, data can be written and read from the file by using some methods in Python. For writing data into the file write() method is used. However, for reading data from the file read() method is used. These methods are very easy to use in Python and described in the following sub-sections. Writing to a File In order to perform a write operation on a file, it must be opened first in either write mode ‘w’, append mode ‘a’, or exclusive mode ‘x\ While opening a file in ‘w’ mode, some caution is required. If file to be opened already exists then it will overwrite the contents of the file. That means, all the previous contents of the file will be lost or erased. Writing data to the file is accomplished by using write() method. This method returns the number of characters written to the file. The syntax of writeQ method is given as follows: fileObj ect. write(string) Code: 9.7. Illustration of writeQ method. # Illustration of write() method with open(“data.txt”,’w’,encoding = ‘cpl252’) as fp: fp.write(“This is an illustration \n”) fP.write(“of writing/n”) fp.write(“data to the file\n”) fp.close( ); The…
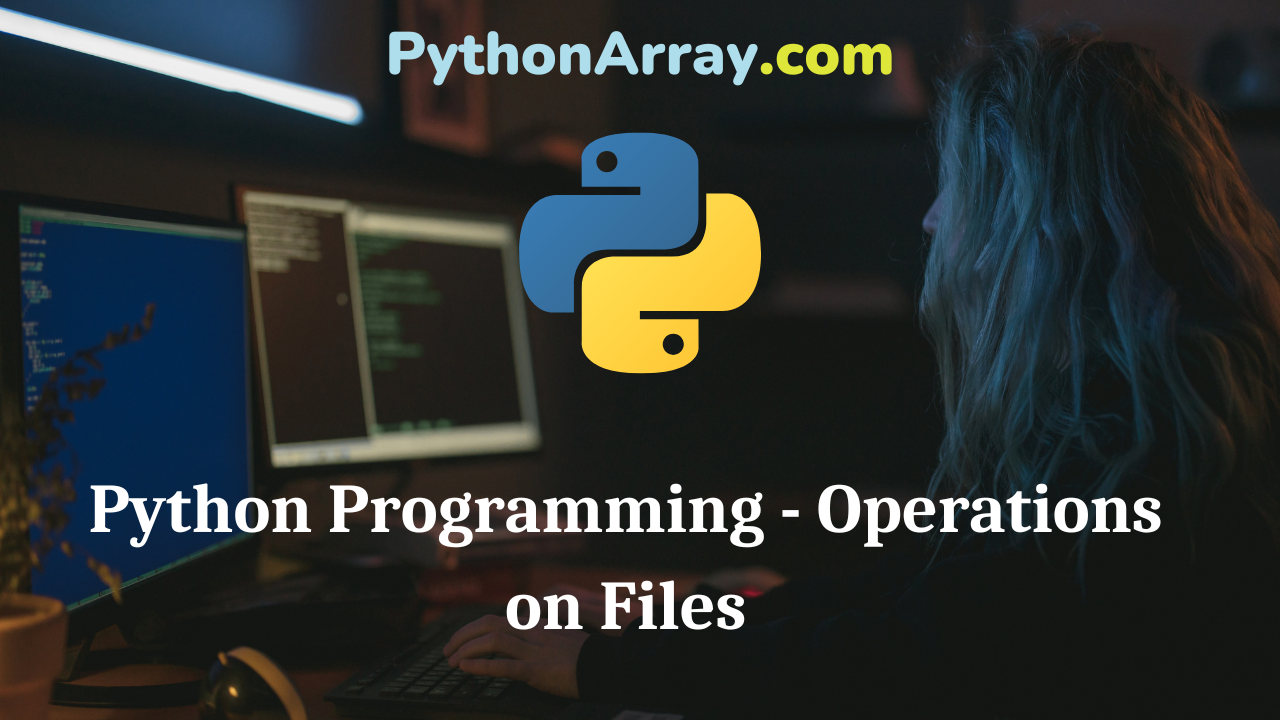
Python Programming – Operations on Files
Python Programming – Operations on Files Initially, we need to open the file first for reading or writing data into it. After performing operations on the file or saving data into it, it needs to be closed. It is necessary to close the file in order to release the resources acquired by the program. Hence, in Python, a file operation takes place in the following order. Open a file Read or write (perform the operation) Close the file Opening a File In Python, a file can be opened by the open() function. It is a very simple built-in function of Python to open a file. This function creates a file object, which would be utilized to call other support methods associated with it. The general syntax of the open method is given as follows: file object= open(file_name,access_mode, buffering) The open( ) function contains three parameters, which are elaborated as under: • file_name: The filename argument is a string value that contains the name of the file that the programmer wishes to access. • access_mode: The access_mode determines the mode in which the file has to be opened, i.e., read, write, append, etc. The detail of modes is given in the…
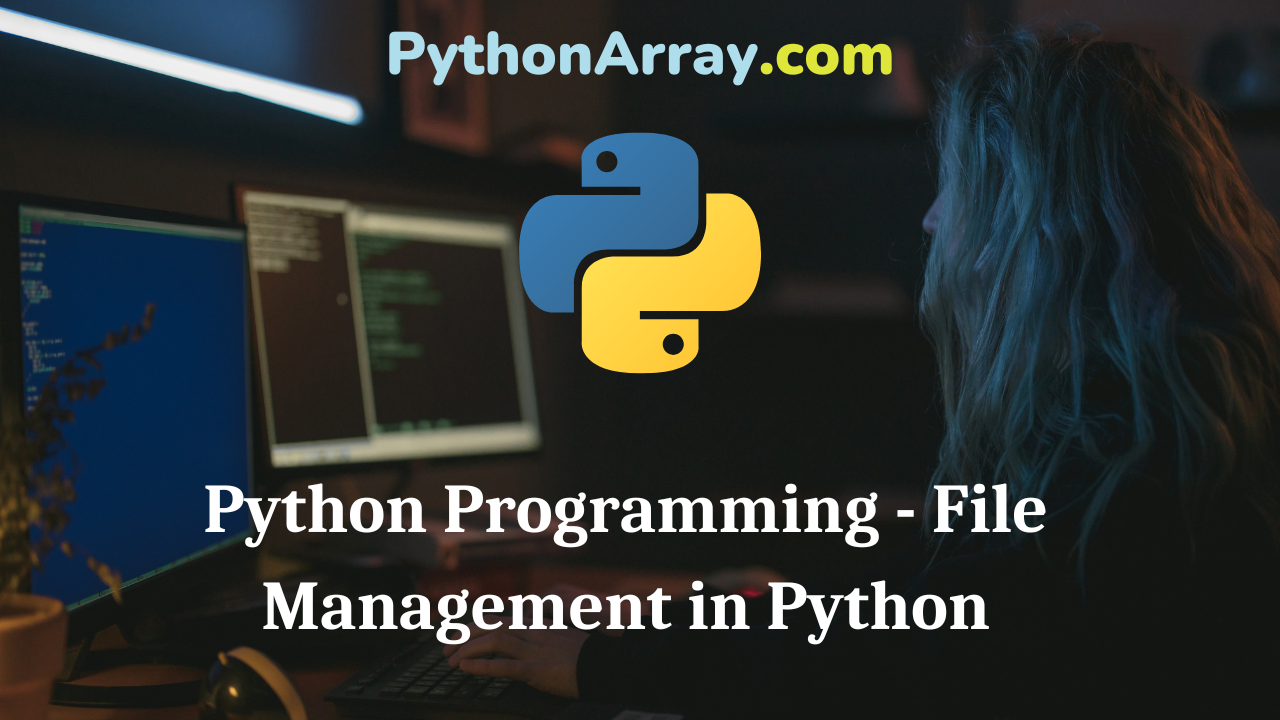
Python Programming – File Management in Python
Python Programming – File Management in Python In all the programs that we have developed so far using Python language, we see that the output is lost when we exit from the program. However, in certain situations, it is necessary to save the data for future use. This can be achieved by making use of files. A file is a place on the disk with a certain name to store related information. This information is stored on the secondary storage memory known as a hard disk, flash drive, etc. However, if we do not store the data on the hard disk in the form of a file, then it resides in the computer’s primary memory RAM (Random Access Memory). The RAM is a volatile memory that loses its data when the computer is turned off. However, a hard disk is permanent storage and also called non¬volatile memory. A computer programmer or data entry operator always prefers to enter the data in the files instead of storing the data in temporary storage. This is done for the following reasons: The complete data will be lost, if either the system is turned off or power failure occurs. Repetition of the same data…
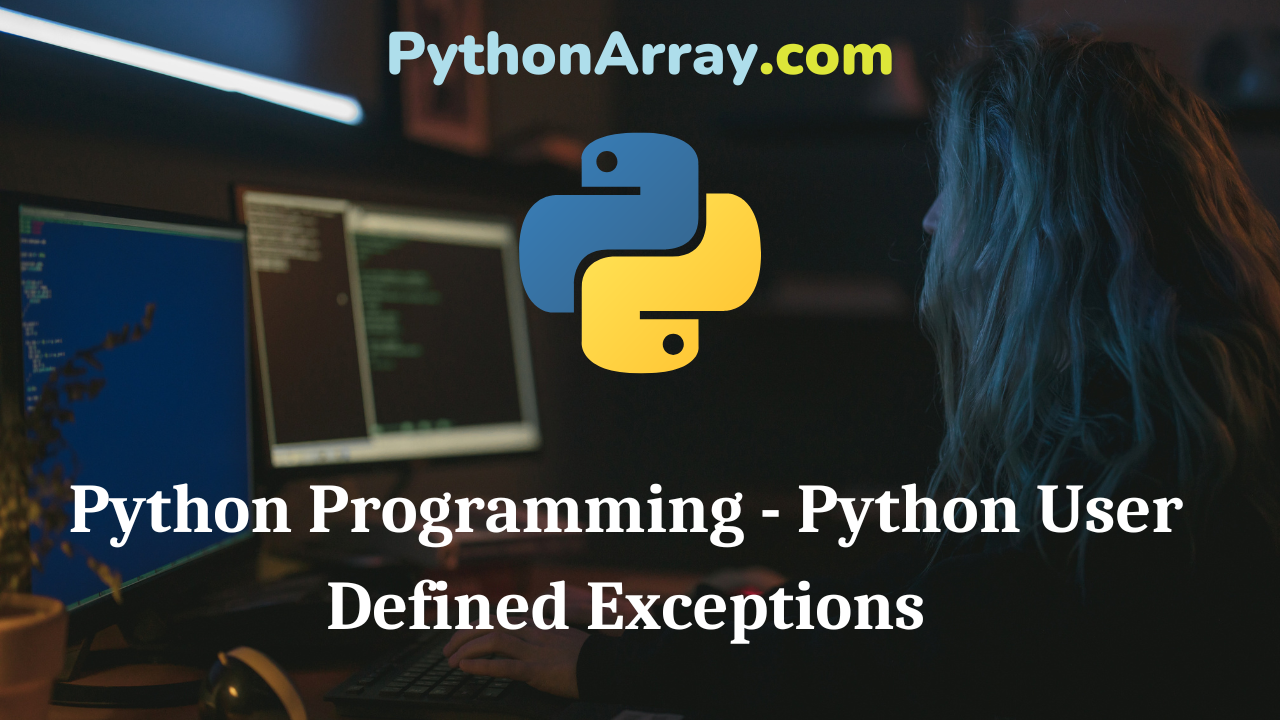
Python Programming – Python User Defined Exceptions
Python Programming – Python User Defined Exceptions In Python, users can create their own exceptions. This can be done by creating a new class that is derived from the Exception class. It is to be noted that most of the built-in exceptions are also derived from the Exception class. On the Python prompt, a user-defined exception can be created as follows as shown in Code 8.6. We see that a user-defined exception NewException is created which is derived from the Exception class. This exception can be raised just like other existing exceptions by using the raise statement with an optional error message. Code: 8.6. Illustration of user defined exception NewException. >>> class NewException(Exception): … pass …>>> raise NewException Traceback (most recent call last): … __main__.NewException>>> raise NewException(“An error has occurred”) Traceback (most recent call last):__main__,NewException:An error has occurred Python Programming – Exception Handling Python Programming – Exception Handling Python Tutorial for Beginners | Learn Python Programming Basics An illustration of user-defined exceptions is given in Code: 8.7. This program presents a number game, where the user has to guess a number. If the user enters a number greater than the saved number then the message is displayed as the number…
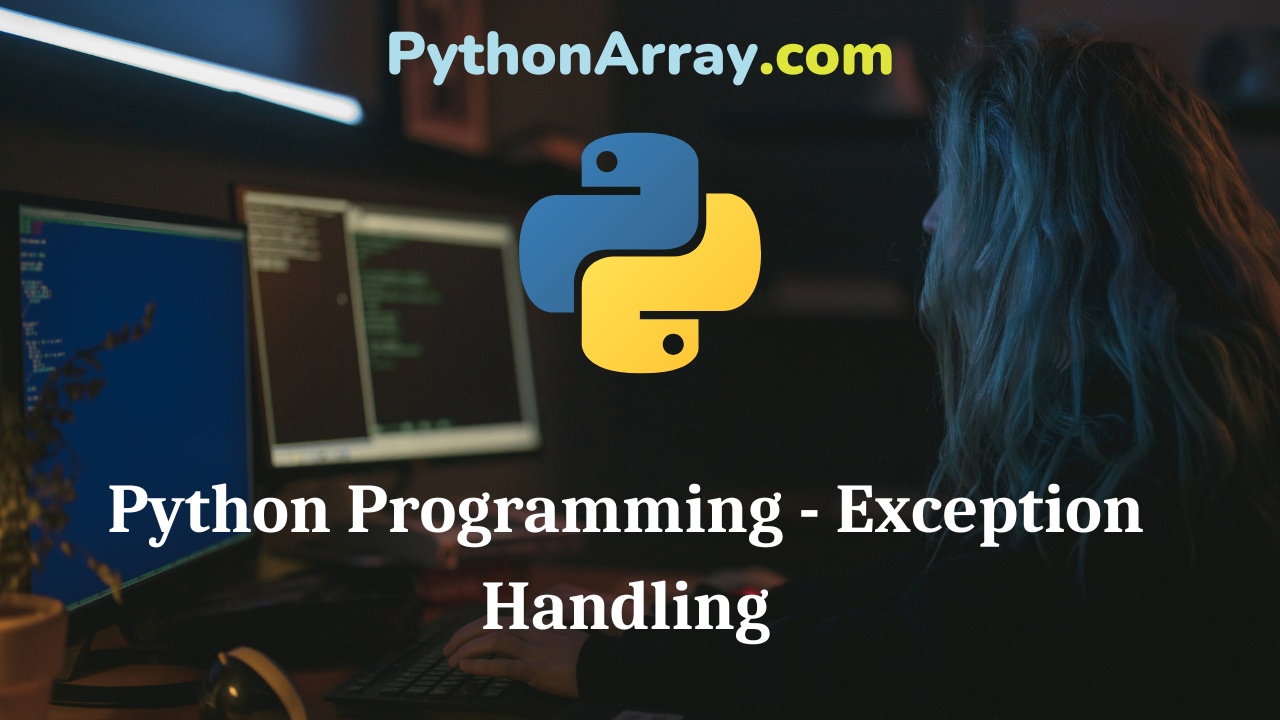
Python Programming – Exception Handling
Python Programming – Exception Handling While writing Python code, certain errors may occur. In the first place, these errors can prevent the program from being executed by the interpreter. These errors are called compile-time errors. For an instance, forgetting to follow the exact syntax of a particular construct such as if statement or making a spelling mistake, missing a semicolon or colon, may cause an interpreter/compile-time error. The program gets executed only after these errors are rectified. Let us consider a program given in Code 8.1. This program determines whether a number is even. In the if statement, we see that colon (:) is missing. The execution of this code raises a syntax error as presented in Fig. 8.1. Introduction to Python Programming – Testing and Debugging Python Programming – Python Loops Python Programming – Assert Statement Code: 8,1, Illustration of interpreter time error (syntax error). # This program illustrates syntax error number=input(‘Enter a number:’) number=int(number) if number%2==0 print(‘Number is even’) print(‘out of if block’) Exception There exist some errors which occur at run time in the program. For instance, attempting to divide by zero or accessing a list, which is not defined, opening a file that does not exist…
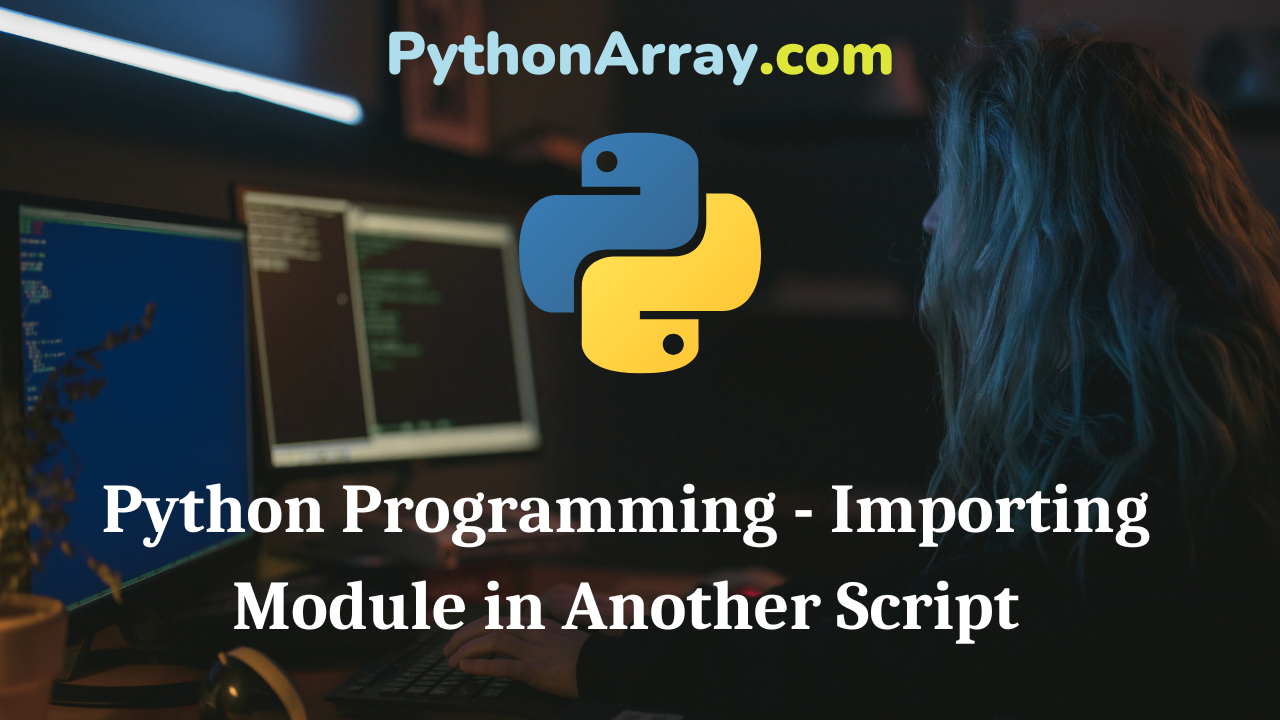
Python Programming – Importing Module in Another Script
Python Programming – Importing Module in Another Script Let us assume, we need to compute the sum of the following series +-+…+ In this series,-the programmer needs to compute the factorial n times. Thus, we will reuse the already created module fact.py and import it inside another script that computes the sum of the above series. The program of the module is given in Code 7.2. It slightly differs from Code 7.1., in that, it returns the value of the computed factorial to the script written in Code: 7.3., where it has been imported. Note that this module is given in Code. 7.3. must be saved in the same directory where module fact.py is saved. Code: 7.2. The illustration of module fact, which returns the value of computed factorial. Code: 7.2. The Illustration of module fact, which returns the value of computed factorial. #This program illustrates the designing/creating of a module def factorial (n): “This module computes factorial”| f=1; for i in range (1, n+1): f=f*i; return f Code: 7.3. The computation of given series by importing fact module. # To compute a series 1/1 !+2/2!+3/3!+…+n/n! import fact n=input(‘enter number:’) n=int(n) sum=0.0 for i in range (1, n+1): sum=sum+(i/fact. factorial(i))…
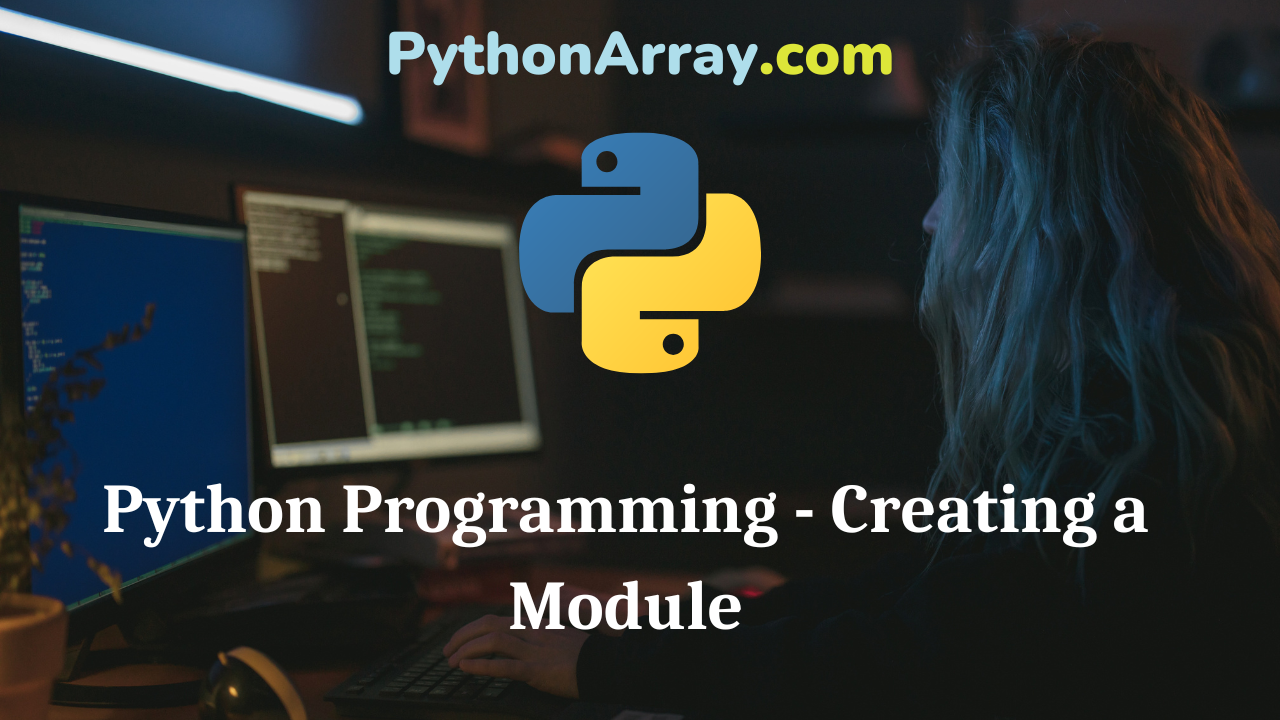
Python Programming – Creating a Module
Python Programming – Creating a Module In this section, we will demonstrate the creation of a module. A module is created as a script file, which contains function definitions that can be called in two ways From the interpreter From another script file or from another function Now, we shall discuss both the above ways of module importing in detail. As we know that factorial is the most frequently used operation and needs to be computed repeatedly in many programs. Here, we will create a module named fact.py, which computes the factorial of a number. Consider the program given in Code 7.1. This program contains only the definition of the function factorial( ), where n is passed as an argument. Code: 7.1. llustration of module fact containing definition of factorial() # This program illustrates the designing/creation of a module def factorial(n): “This module computes factorial” f = i for i in range (1, n+1): f=f*i; print(f) return Python Tutorial