There may be situations where we want to convert a list containing float numbers to string while working in python. In this article, we will look at different ways to convert the list containing float numbers to a string which contains the elements of the list as space separated sub strings. Python Programming – String Functions and Methods Python Programming – Built-in Functions Python String Methods for String Manipulation Important functions for the task to convert a list containing float numbers to string To convert a list of floating point numbers to string, we will need several string methods which we will discuss first and then perform the operations. str() function The str() function converts an integer literal,a float literal or any other given input to a string literal and returns the string literal of the input after conversion. This can be done as follows. float_num=10.0 str_num=str(float_num) join() method join() method is invoked on a separator and an iterable object of strings is passed to the method as input. It joins each string in the iterable object with the separator and returns a new string. The syntax for join() method is as separator.join(iterable) where separator can be any character and iterable can be a list or tuple etc.…
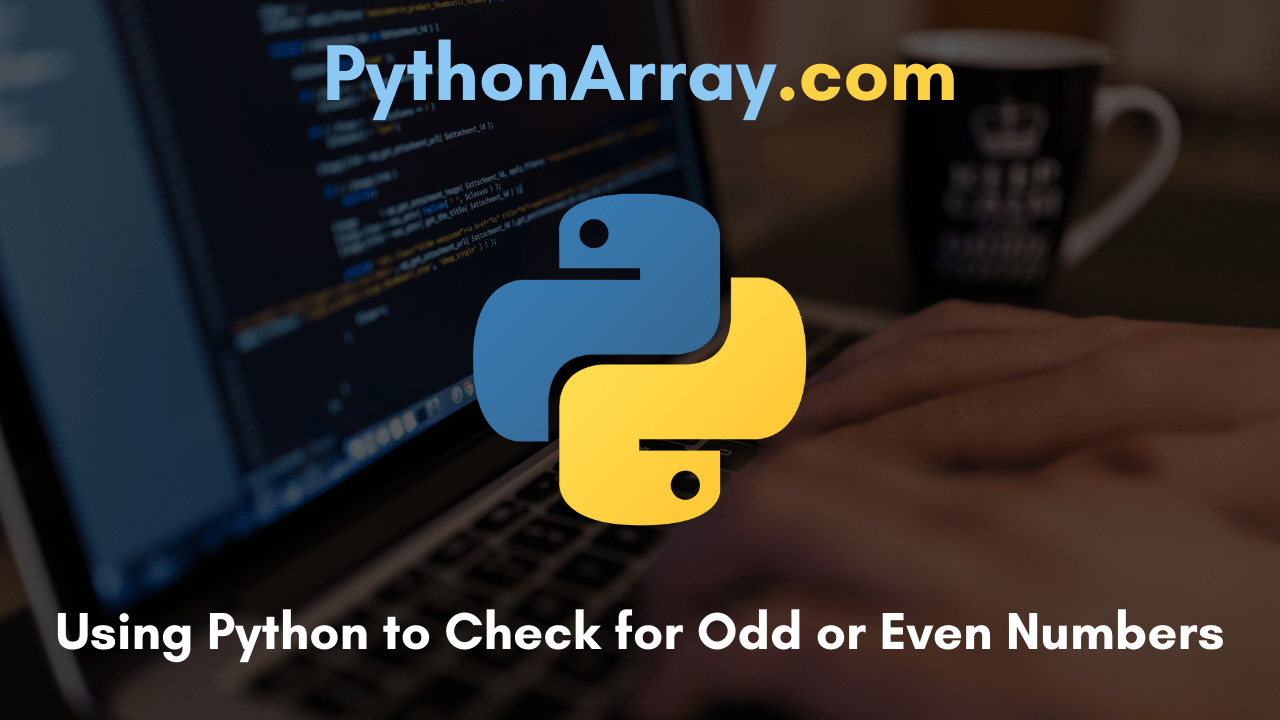
Using Python to Check for Odd or Even Numbers | Python Program to Check If number is Even or Odd
In this Python Tutorial, beginners will effortlessly learn how to check for odd or even numbers using python. Along with the detailed explanation, you will also see the Python program to check a number is even or odd using different methods. Let’s get into this Tutorial of Using Python to Check for Odd or Even Numbers and become proficient in python programming. The Tutorial on how to check a number is even or odd in python cover the following stuff: Python, how to check if a number is odd or even Python Program to check if a Number is Odd or Even using Modulor Operator Python Program to Verify if a Number is Odd or Even Python Program To Check A Number Is Even Or Odd Using Bitwise Operator Using the Division Operator to Check for Odd or Even Numbers Python, how to check if a number is odd or even It’s moderately straightforward to use Python to make calculations and arithmetic. One cool type of arithmetic that Python can do is to calculate the remainder of one number divided by another. To accomplish this, you have to use the modulo operator (otherwise known as the percentage sign: %). When…
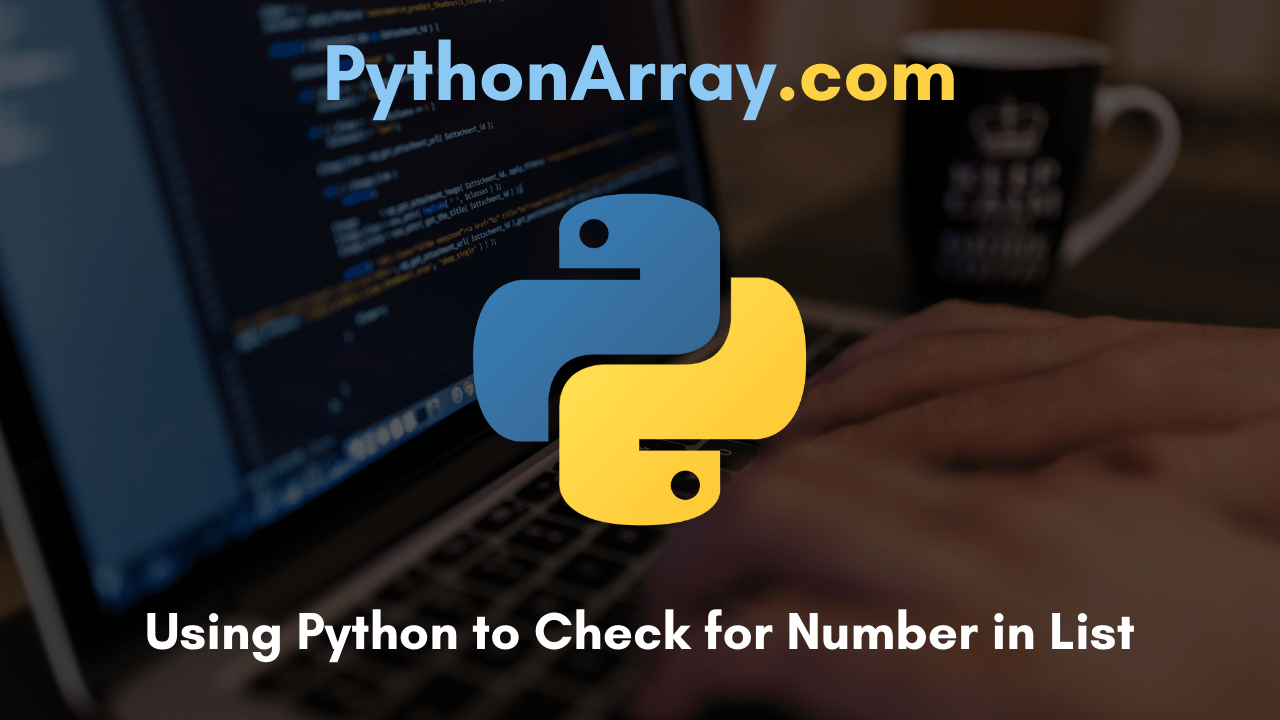
Using Python to Check for Number in List | Python List Contains | How to check if element exists in List in Python?
Today’s Python Tutorial brought to you one more essential topic that you should aware of ie., Checking the Number in a List using Python. You have an idea that List is an essential container in python as it stores elements of all the datatypes as a collection. There are different ways to check if a number or element is in a list or not. Explore more information about How to Check if element exists in list in Python by going through this tutorial. In this Using Python to Check for Number in List Tutorial, you’ll learn: Python List Contains Check if element exists in list using python “in” Operator Check if Number exists in list in python using “not in” Operator Check if element exist in list using list.count() function Check if element exist in list based on custom logic using any() function Python all() method to check if the list exists in another list Python List Contains In order to check if the list contains a specific item in python, make use of inbuilt operators ie., ‘in’ or ‘not in’, or many other functions. One of the most convenient ways to check if an item exists on the list…
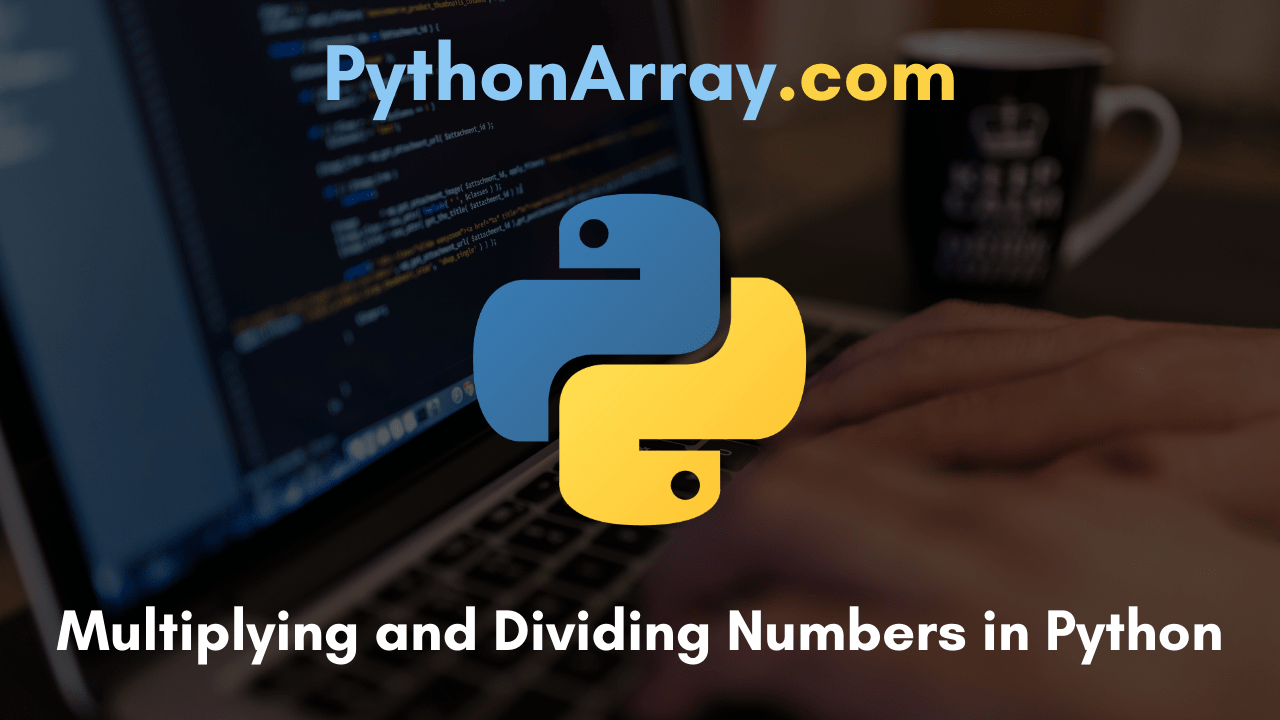
Multiplying and Dividing Numbers in Python | How to Multiply and Divide in Python?
Have you ever multiplied or divided numbers in other coding languages? If yes, then you’ll discover the process for Multiplying and Dividing Numbers in Python is very similar, if not pretty much exactly the same. Knowing how to multiply and divide numbers using Python is necessary not just to know the answer to something like 10 times 5, but because you can use certain operations in any of your complex code to manage other functionalities. In this Multiplying Dividing Numbers Python Tutorial, you’ll learn the following stuff: How to do Multiplying Numbers using Python? Python program to multiply floating-point number How to perform Dividing numbers in Python? Program to perform multiplication and Division on two numbers in Python Python program for multiplication and division of complex number How to do Multiplying Numbers using Python? To multiply two numbers by each other, you’ll use the “*” operator, like this: 2*4 5*10 3*7 That’s just about all it takes to perform some multiplication. Don’t forget that if you want to print the results of the numbers you’re multiplying, you’ll have to use the print command, like this: print(2*4) print(5*10) print(3*7) The output of the code above would be: 8 50 21 Do Check: Using…
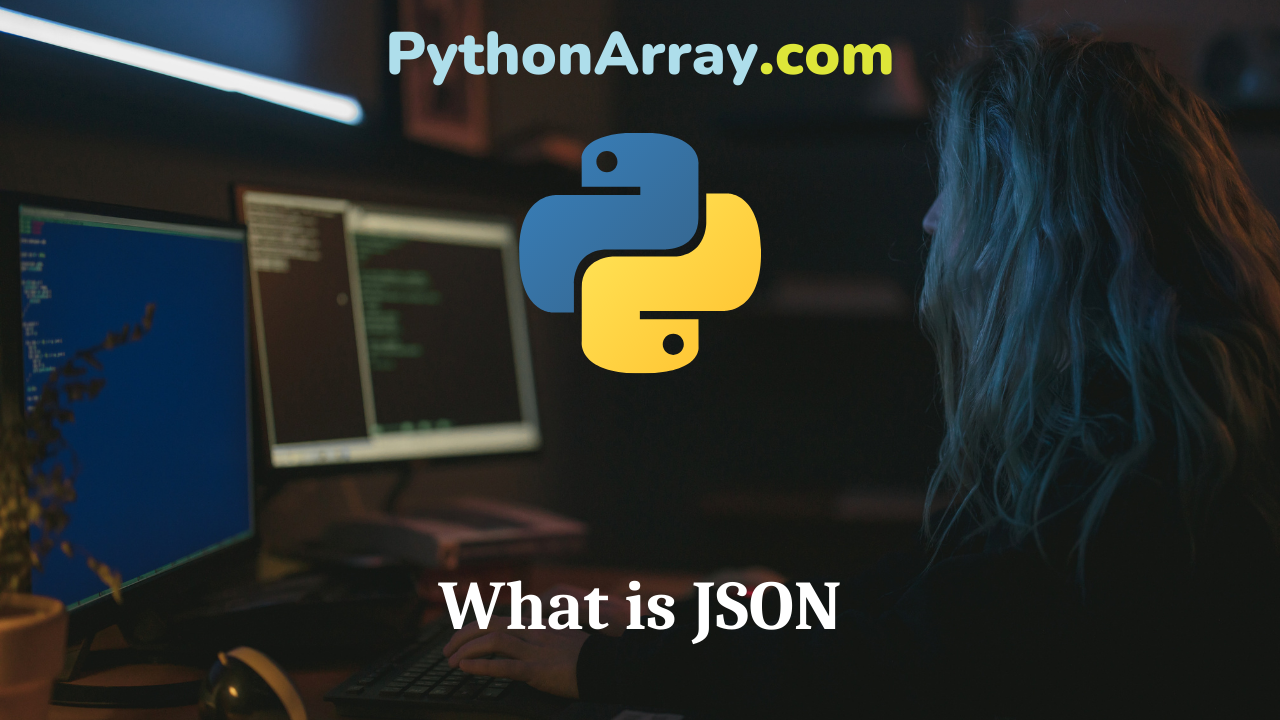
What is JSON
What is JSON? JSON (JavaScript Object Notation) is a compact, text based format for computers to exchange data. The official Internet media type for JSON is application/json, and the JSON filename extension is .json JSON is built on two structures: A collection of name/value pairs An ordered list of values. JSON take these forms: objects, array, value, string, number Parse JSON objects in Python Using the Requests Library in Python Google Command Line Script Object Unordered set of name/value pairs. Begins with { and ends with }. Each name is followed by : (colon) The name/value pairs are separated by , (comma). Array Ordered collection of values. Begins with [ and ends with ]. Values are separated by , (comma). Value Can be a string in double quotes, number, or true or false or null, or an object or an array. String A sequence of zero or more Unicode characters, wrapped in double quotes, using backslash escapes. Number Integer, long, float The following example shows the JSON representation of an object that describes a person: { “firstName”: “John”, “lastName”: “Smith”, “age”: 25, “address”: { “streetAddress”: “21 2nd Street”, “city”: “New York”, “state”: “NY”, “postalCode”: “10021” }, “phoneNumber”: [ { “type”:…
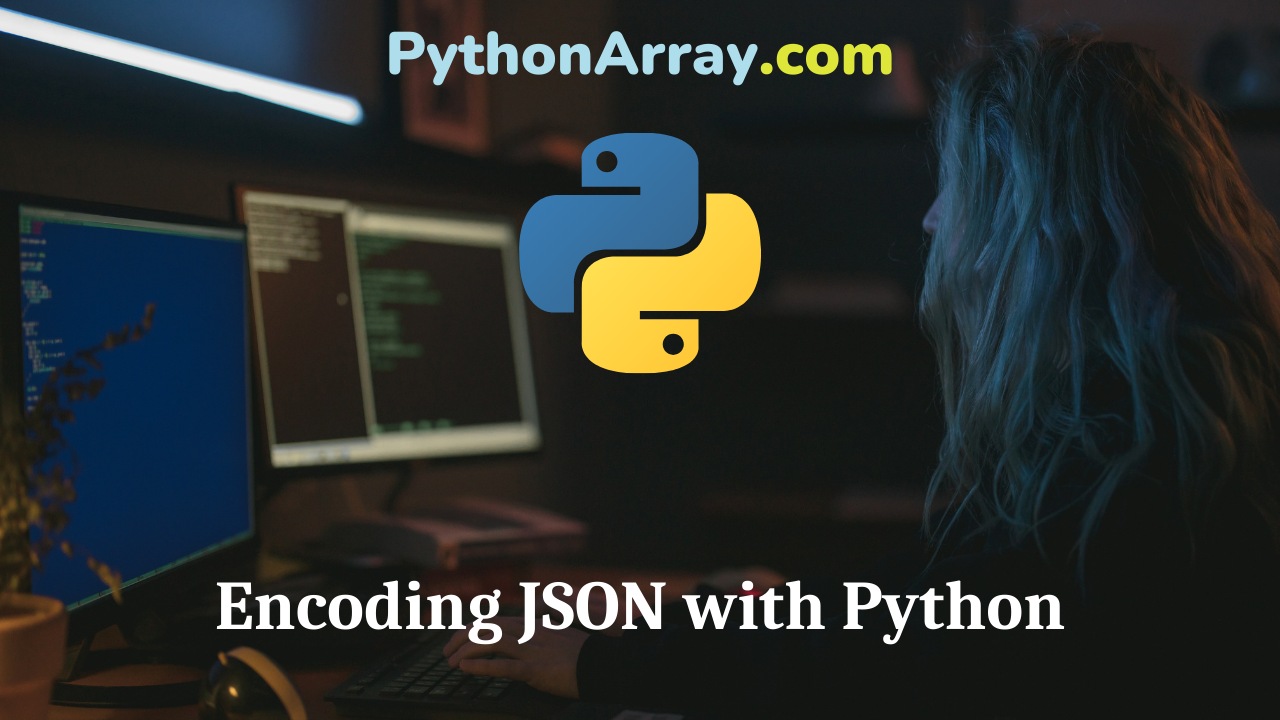
Encoding JSON with Python
Python comes pre-equipped with a JSON encoder and decoder to make it very simple to play nice with JSON in your applications The simplest way to encode JSON is with a dictionary. This basic dictionary holds random values of various datatypes. data = { a: 0, b: 9.6, c: “Hello World”, d: { a: 4 } } Using the Requests Library in Python CommandLineFu with Python Python Command Line IMDB Scraper We then use json.dumps() to convert the dictionary to a JSON object. import json data = { a: 0, b: 9.6, c: “Hello World”, d: { a: 4 } } json_data = json.dumps(data) print(json_data) This will print out {“c”: “Hello World”, “b”: 9.6, “d”: {“e”: [89, 90]}, “a”: 0} Notice how the keys are not sorted by default, you would have to add the sort_keys=True argument to json.dumps() like so. import json data = { a: 0, b: 9.6, c: “Hello World”, d: { a: 4 } } json_data = json.dumps(data, sort_keys=True) print(json_data) Which would then output the sorted keys. {“a”: 0, “b”: 9.6, “c”: “Hello World”, “d”: {“e”: [89, 90]}}
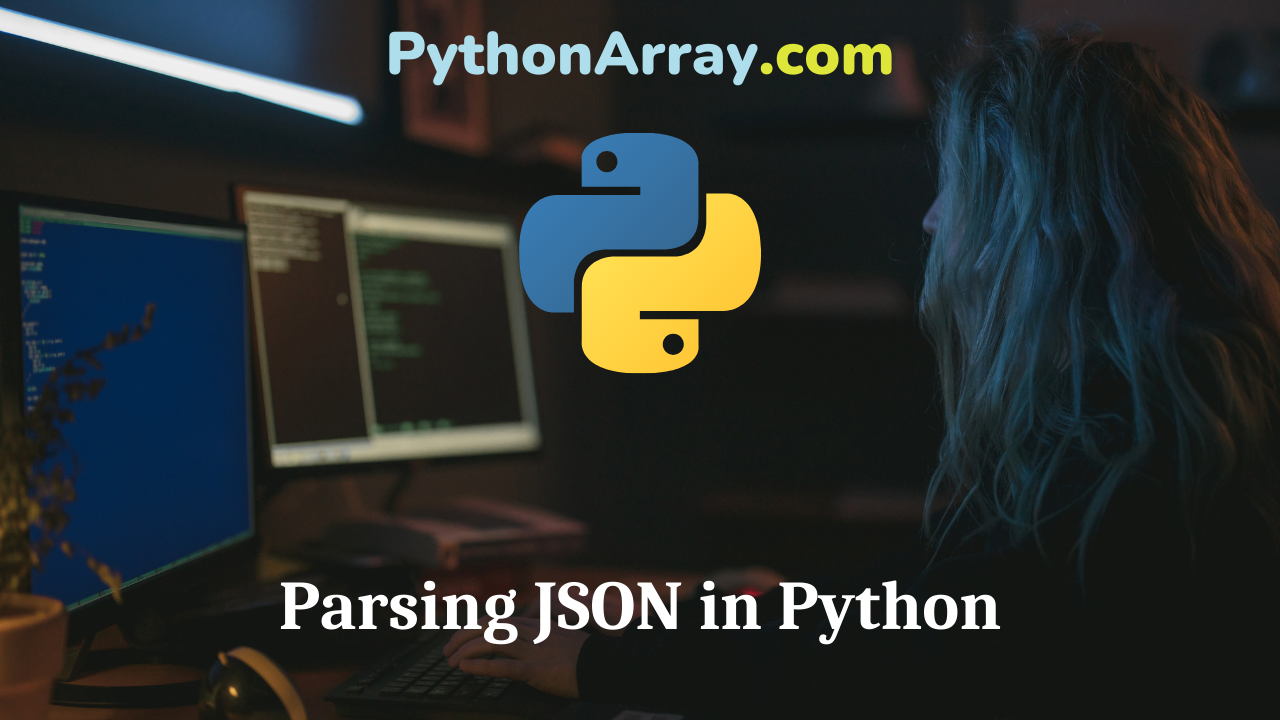
Parsing JSON in Python
Overview Request to an HTTP API is often just the URL with some query parameters. API Response The responses that we get from an API are data, that data can come in various formats, with the most popular being XML and JSON. Many HTTP APIs support multiple response formats so that developers can choose the one they’re more comfortable parsing. CommandLineFu with Python How to use the Vimeo API in Python Extracting YouTube Data With Python Using API Getting Started To get started, lets create a simple data structure and put in some data. First we import the json module into our program. import json # Create a data structure data = [ { ‘Hola’:’Hello’, ‘Hoi’:”Hello”, ‘noun’:”hello” } ] To print the data to screen, is as simple as: print ‘DATA:’, (data) When we print the Data as above, we will see the following output: DATA: [{‘noun’: ‘hello’, ‘Hola’: ‘Hello’, ‘Hoi’: ‘Hello’}] JSON Functions When you use JSON in Python, there are different function that we can make use of Json Dumps The json.dumps function takes a Python data structure and returns it as a JSON string. json_encoded = json.dumps(data) # print to screen print json_encoded OUTPUT: [{“noun”: “hello”, “Hola”:…
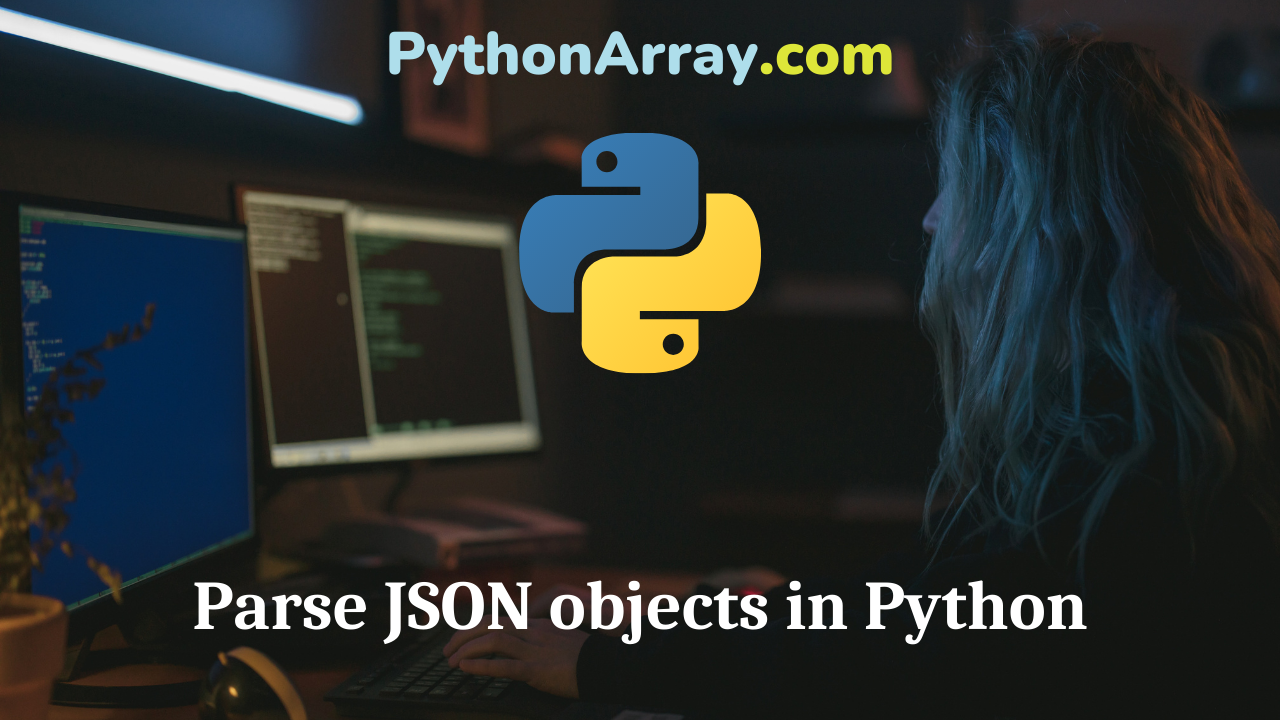
Parse JSON objects in Python
Overview In this post, we will explain how you can parse JSON objects in Python. Knowing how to parse JSON objects is useful when you want to access an API from various web services that give the response in JSON. Getting Started First thing you have to do, is to find an URL to call the API. In my example, I will use the Twitter API. Start with importing the modules that we need for the program. import json import urllib2 Open the URL and the screen name. url = “http://api.twitter.com/1/statuses/user_timeline.json?screen_name=wordpress” Print out the result print data Google Command Line Script CommandLineFu with Python Extracting YouTube Data With Python Using API Using the Twitter API to parse data This is a very simple program, just to give you an idea of how it works. #Importing modules import json import urllib2 # Open the URL and the screen name url = “http://api.twitter.com/1/statuses/user_timeline.json?screen_name=wordpress” # This takes a python object and dumps it to a string which is a JSON representation of that object data = json.load(urllib2.urlopen(url)) #print the result print data If you are interested to see another example of how to use JSON in Python, please have a look at the “IMDB…
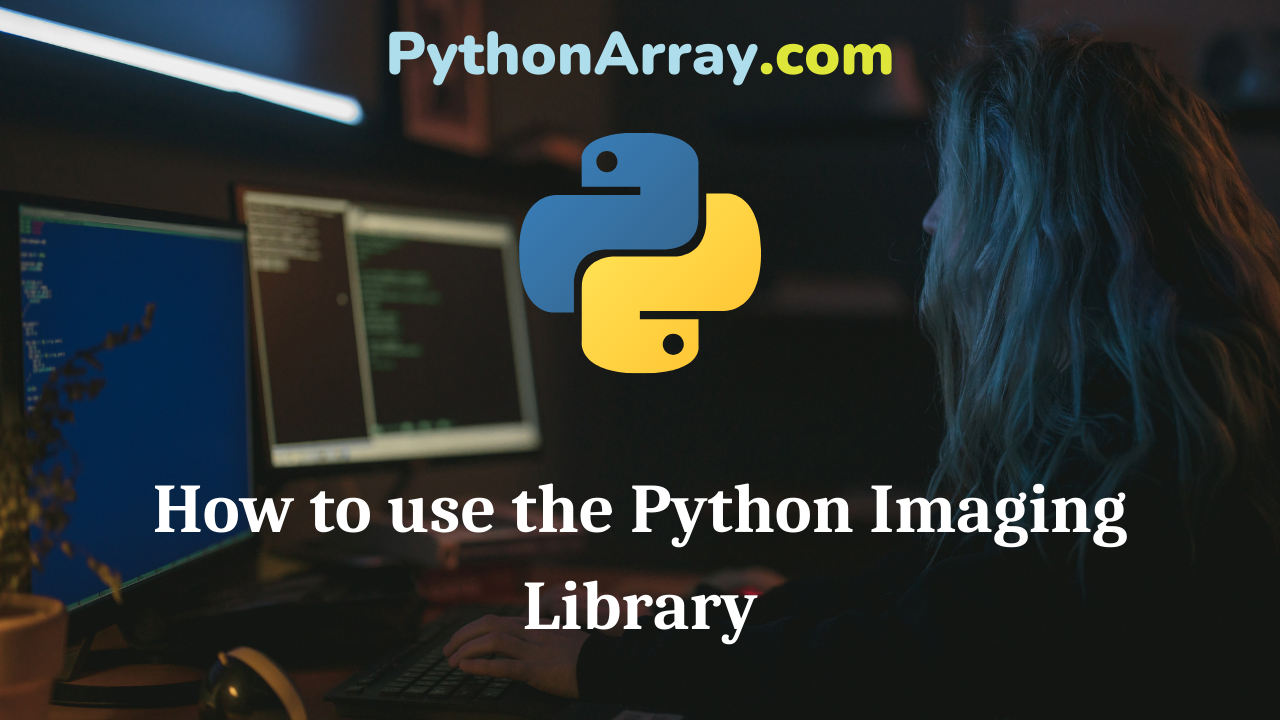
How to use the Python Imaging Library
PIL is deprecated, obsolete. please use Pillow. Make your life easier with Virtualenvwrapper Python – Quick Guide How to Use Python to Convert Miles to Kilometers | Python Program to Convert Miles to KM Unit Measurement Find out How to use the Pillow How to use Pillow, a fork of PIL
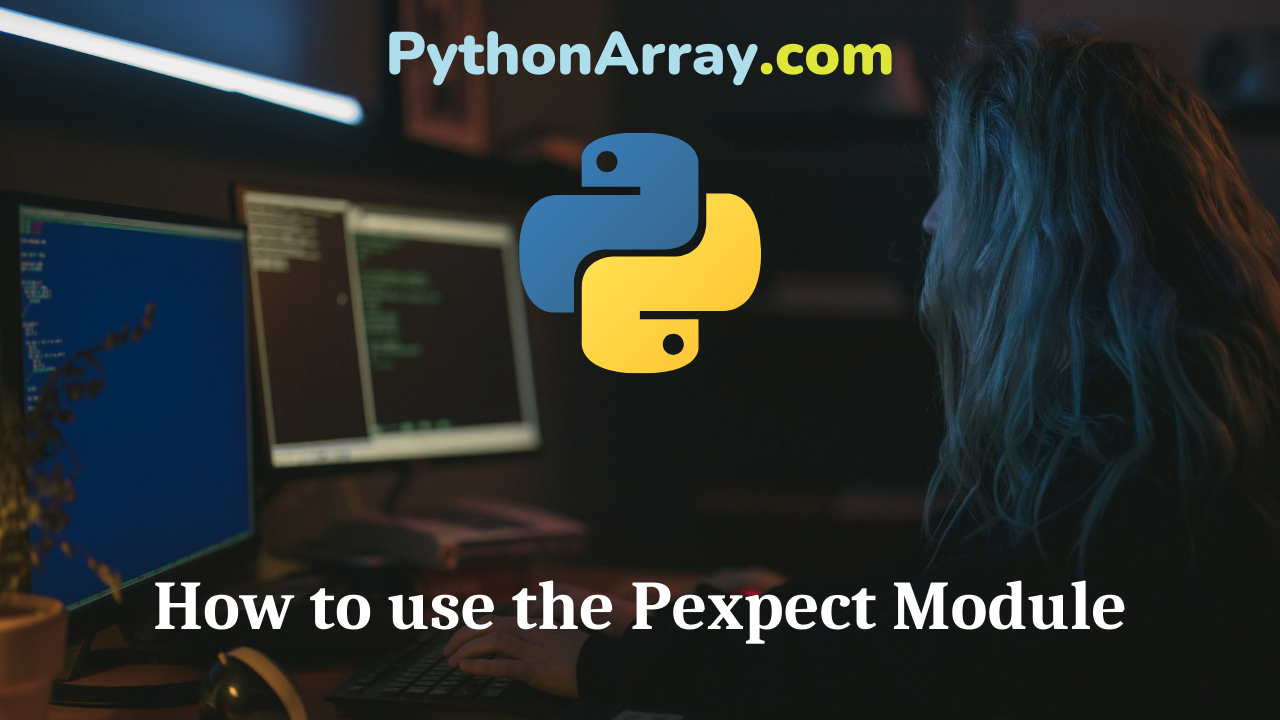
How to use the Pexpect Module
This article is based on documentation from The reason I started to use Pexepect was because I was looking for a module that can take care of some of the automation needs I have (mostly with ssh and ftp). You can use other modules such as subprocess, but I find this module easier to use. Note, this post is not for a Python beginner, but hey it’s always fun to learn new things SSH Connection with Python How to use FTP in Python Python Code Examples What is Pexpect? Pexpect is a pure Python module that makes Python a better tool for controlling and automating other programs. Pexpect is basically a pattern matching system. It runs program and watches output. When output matches a given pattern Pexpect can respond as if a human were typing responses. What can Pexpect be used for? Pexpect can be used for automation, testing, and screen scraping. Pexpect can be used for automating interactive console applications such as ssh, ftp, passwd, telnet, etc. It can also be used to control web applications via `lynx`, `w3m`, or some other text-based web browser. Installing Pexpect The latest version of Pexpect can be found here wget http://pexpect.sourceforge.net/pexpect-2.3.tar.gz tar xzf pexpect-2.3.tar.gz…