This is a new series of articles here at Python for beginners, that are supposed to be a starting point for complete beginners of Python. See it as a cheat sheet, reference, manual or whatever you want. The purpose is to very short write down the basics of Python. This page will describe how to use Modules in Python. Python Modules Python modules makes programming a lot easier. It’s basically a file that consist of already written code. When Python imports a module, it first checks the module registry (sys.modules) to see if the module is already imported. If that’s the case, Python uses the existing module object as is. There are different ways to import a module. This is also why Python comes with battery included How to import modules in Python How To Use sys.arv in Python Command line arguments (sys.argv) Importing Modules Let’s how the different ways to import a module import sys #access module, after this you can use sys.name to refer to things defined in module sys. from sys import stdout # access module without qualiying name. This reads from the module “sys” import “stdout”, so that we would be able to refer “stdout”in our…
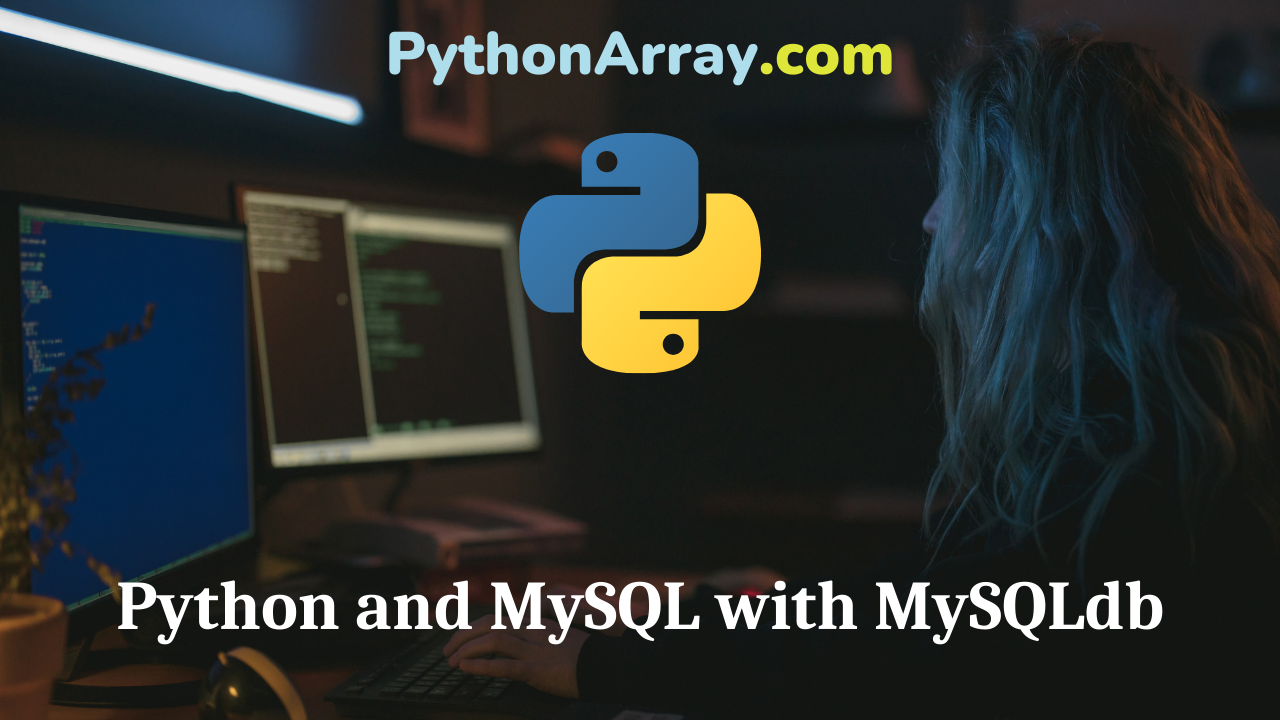
Python and MySQL with MySQLdb
Last week I was looking for a Python module that I could use to interact with a MySQL database server. MySQLdb is doing just that. “MySQLdb is a thin Python wrapper around _mysql which makes it compatible with the Python DB API interface (version 2). In reality, a fair amount of the code which implements the API is in _mysql for the sake of efficiency.” Writing Your First Python Django Application Introduction to SQLite in Python Python’s SQLAlchemy vs Other ORMs To install and use it, simply run: sudo apt-get install python-mysqldb When that is done, you can start importing the MySQLdb module in your scripts. I found this code snippet on Alex Harvey website # Make the connection connection = MySQLdb.connect(host=’localhost’,user=’alex’,passwd=’secret’,db=’myDB’) cursor = connection.cursor() # Lists the tables in demo sql = “SHOW TABLES;” # Execute the SQL query and get the response cursor.execute(sql) response = cursor.fetchall() # Loop through the response and print table names for row in response: print row[0] For more examples on how to use MySQLdb in Python, please take a look on Zetcode.com.
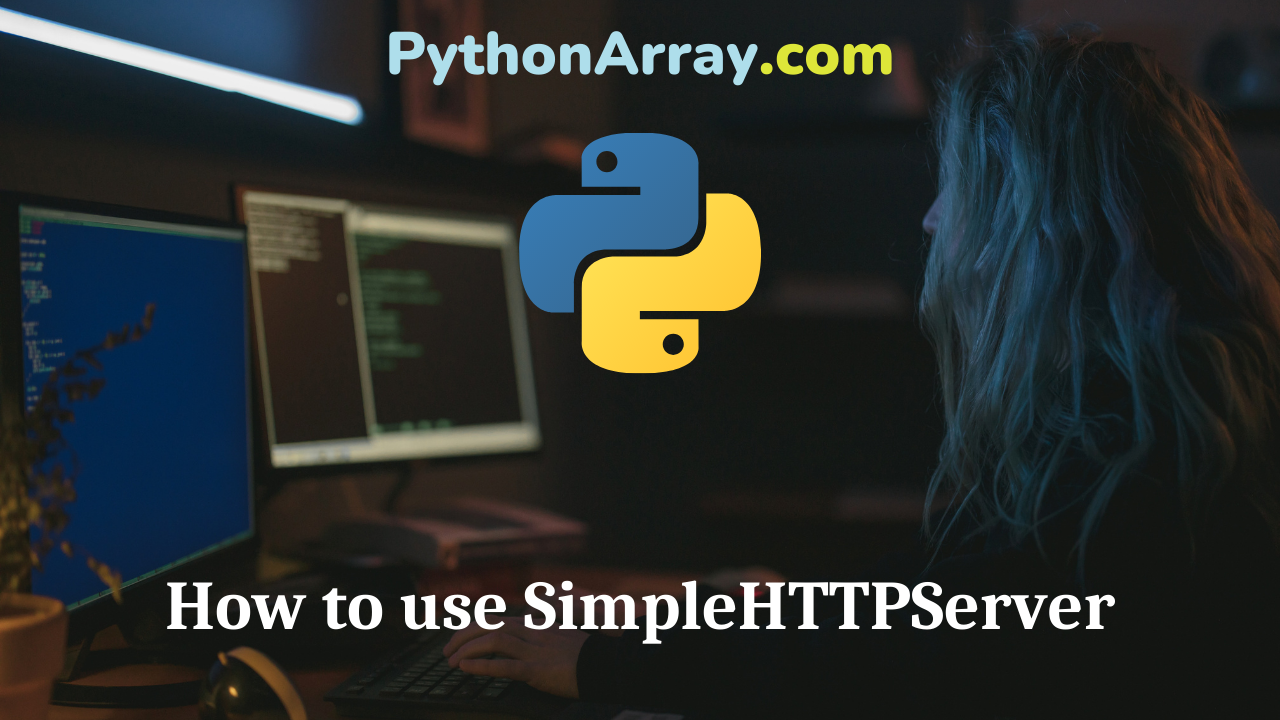
How to use SimpleHTTPServer
Overview In this post, we will look at the built-in web server in Python. What is it? The SimpleHTTPServer module that comes with Python is a simple HTTP server that provides standard GET and HEAD request handlers. Why should I use it? An advantage of the built-in HTTP server is that you don’t have to install and configure anything. The only thing that you need, is to have Python installed. That makes it perfect to use when you need a quick web server running and you don’t want to mess with setting up apache. You can use this to turn any directory in your system into your web server directory. How to use FTP in Python Package Your Python Django Application into a Reusable Component How to Install Django on Windows, Mac and Linux How do I use it? To start a HTTP server on port 8000 (which is the default port), simple type: python -m SimpleHTTPServer [port] This will now show the files and directories which are in the current working directory. You can also change the port to something else: $ python -m SimpleHTTPServer 8080 How to share files and directories In your terminal, cd into whichever directory…
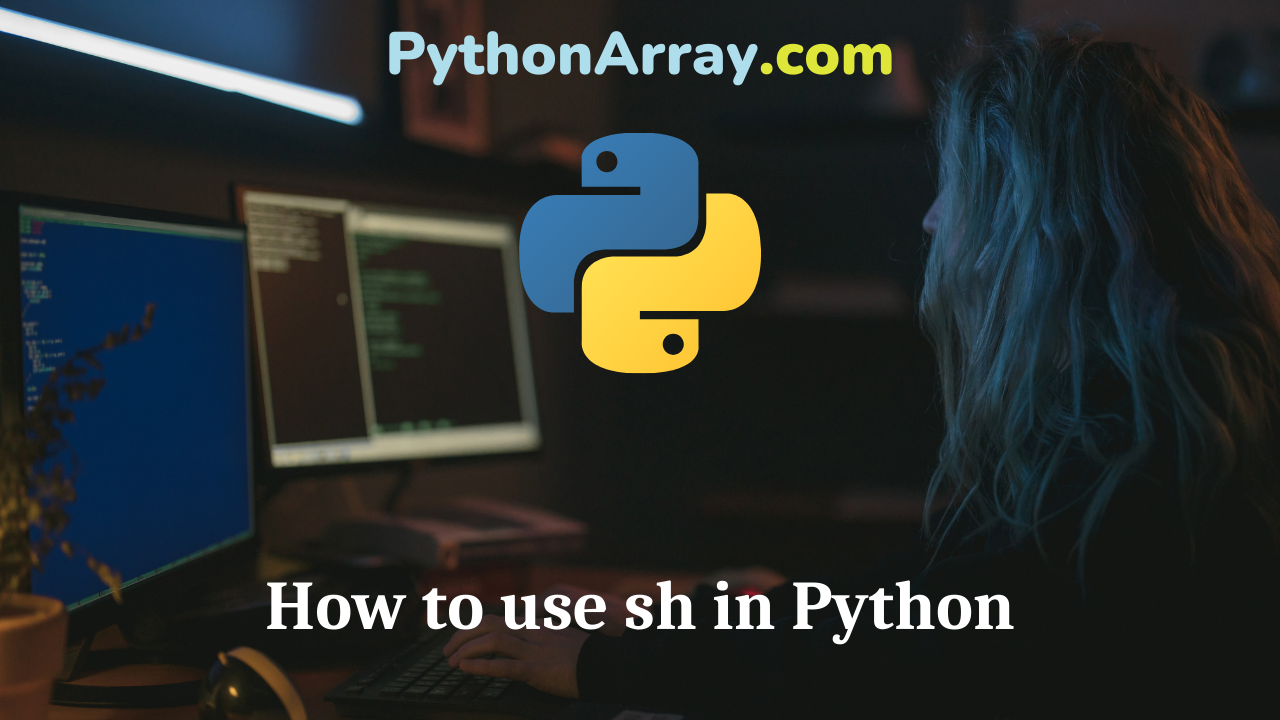
How to use sh in Python
What is sh? sh is a unique subprocess wrapper that maps your system programs to Python functions dynamically. sh helps you write shell scripts in Python by giving you the good features of Bash (easy command calling, easy piping) with all the power and flexibility of Python. [source] Starting with sh sh is a full-fledged subprocess interface for Python that allows you to call any program as if it were a function. sh lets you call just about anything that you could run from a login shell much more neatly than you can with subprocess. Popen, and more importantly lets you capture and parse the output much more readily. Installation The installation of sh is done through the pip command pip install sh How to Use The Envoy Wrapper IPython a short introduction How to use Pip and PyPI Usage The easiest way to get up and running is to import sh directly or import your program from sh. Every command you want to run is imported like any other module. That command is then usable just like a Python statement. Arguments are passed per usual, and output can be captured and worked with in a like fashion. # get interface information import sh print…
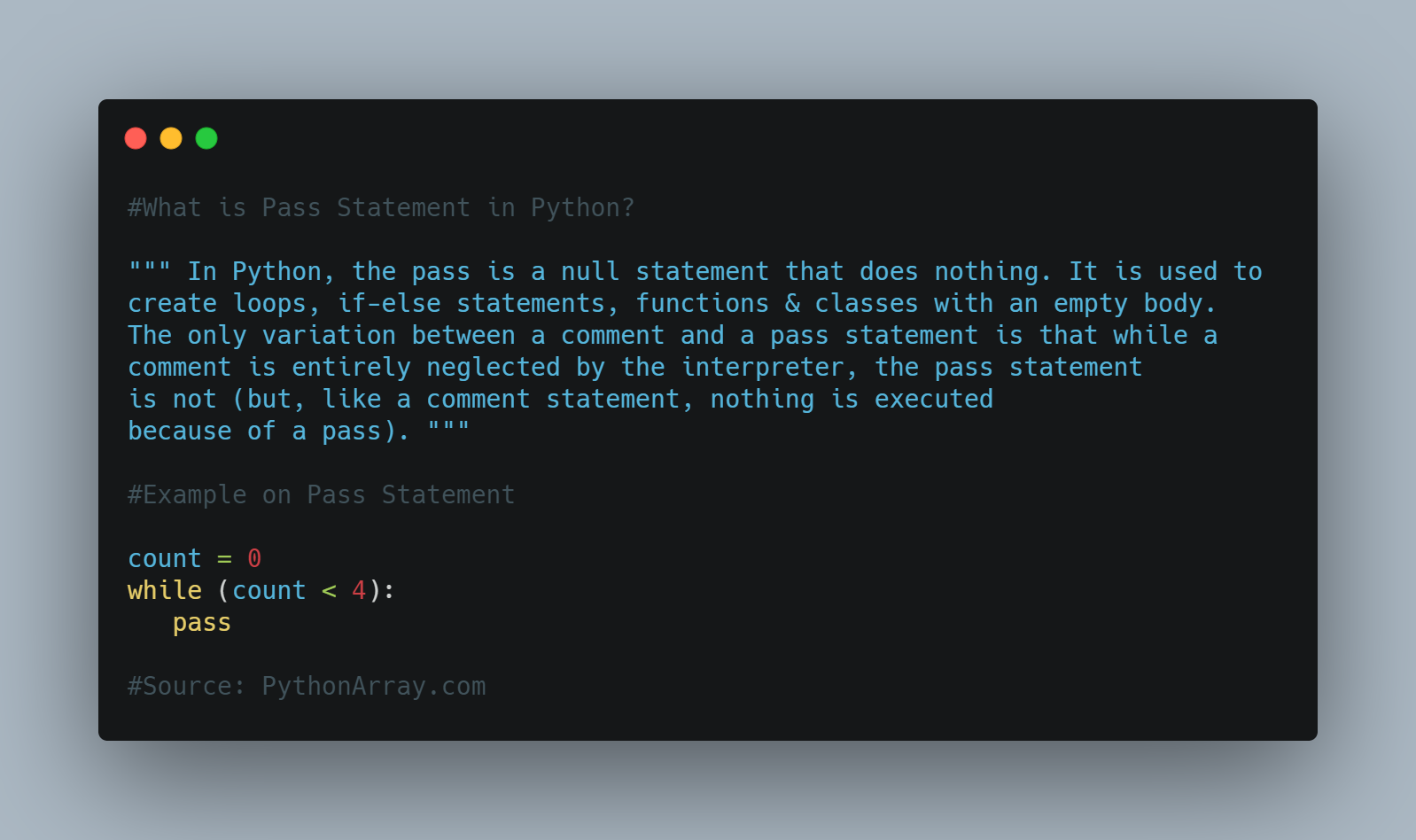
Using Python’s Pass Statement | What is Pass Statement in Python? | Python pass statement example
Hello learners!! I hope your learnings in python programming going well by using our previous tutorials. If you are searching to learn about the python pass statement concept then this tutorial is the one-stop destination for you all. Here we have discussed completely what is python pass statement, the syntax of the pass, python’s pass statement example, etc. Also, you can find some more information on Pass Statement vs Comment from this Pass Statement in Python Tutorial. The Tutorial of Using Python’s Pass Statement includes the following stuff: What is Pass Statement in Python? Syntax of Pass Example on Pass Statement Pass statement vs comment What is Pass Statement in Python? In Python, the pass is a null statement that does nothing. It is used to create loops, if-else statements, functions & classes with an empty body. The only variation between a comment and a pass statement is that while a comment is entirely neglected by the interpreter, the pass statement is not (but, like a comment statement, nothing is executed because of a pass). Python Programming – Python Control Statements Python Programming – Python User Defined Functions Python Programming – Python Anonymous Functions Syntax of Pass The syntax of…
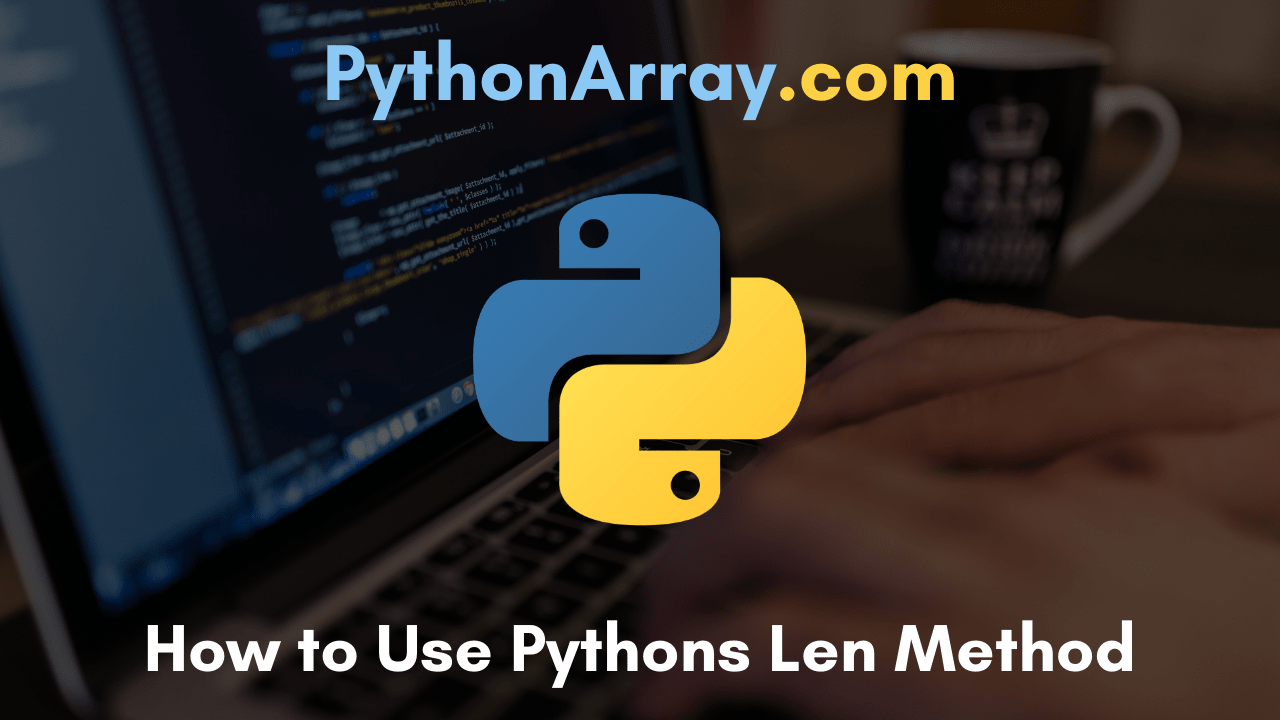
Python String Length | How to Use Python’s len() Method? | Python len() Example
In this tutorial, python beginners and expert coders can easily learn how to use python’s len() method with different objects. Apart from this, you may also discover python len() examples with output in this Python len() function tutorial. Let’s find the length of an object by using this len() method and code perfectly in complex programs. The Tutorial of Python len() Method includes the following stuff: Python String Length | Python len() Function Syntax Parameters of the len() Return Value How to use len() in Python? Python len() Example Internal Working of the len() Function in Python How len() works with tuples, lists, and range? How len() works for custom objects? How len() works with strings and bytes? How len() works with dictionaries and sets? Python String Length | Python len() Function Python’s len() method can be used to easily find the length of a string. It’s a simple and quick way to measure the length of a string (the number of characters) without having to write a lot of code. Syntax The syntax of len() is: len(s) How to Use Python to Multiply Strings | Multiply Strings in Python with Examples How to Multiply Matrices in Python? | Python…
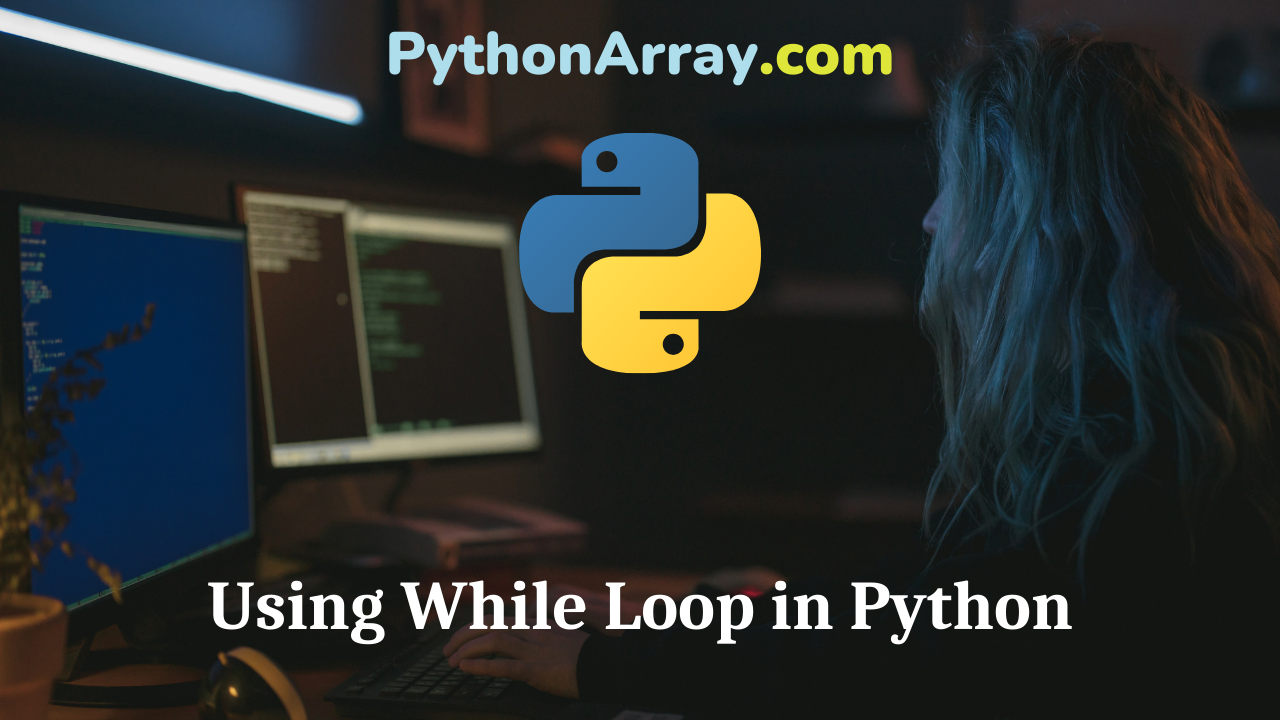
Using While Loop in Python
Overview In this post, I will write about While loops in Python. If you have read earlier posts For and While Loops you will probably recognize a lot of this. Count from 0 to 9 This small script will count from 0 to 9. i = 0 while i < 10: print i i = i + 1 Loops in Python Using math in python Python Programming – Python Control Statements What does it do? Between while and the colon, there is a value that first is True but will later be False. As long as the statement is True , the rest of the code will run. The code that will be run has to be in the indented block. The i = i + 1 adds 1 to the i value for every time it runs. Be careful to not make an eternal loop, which is when the loop continues until you press Ctrl+C. while True: print “Hello World” This loop means that the while loop will always be True and will forever print Hello World.
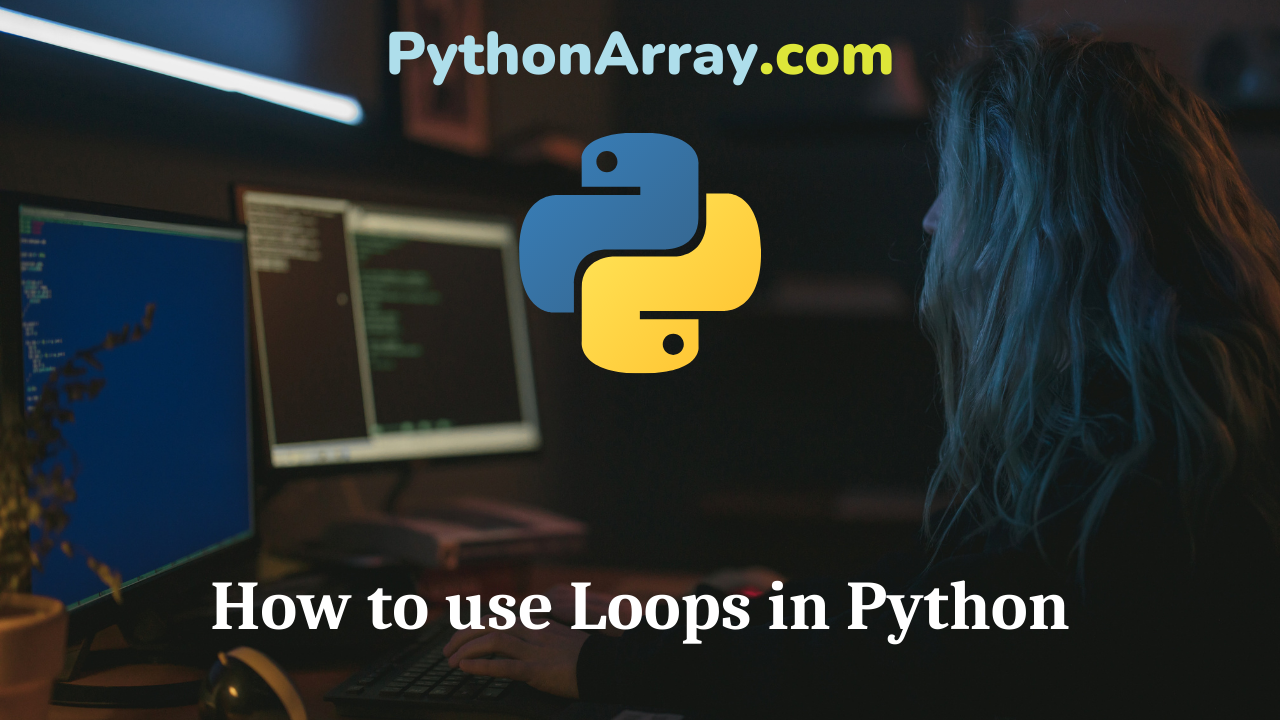
How to use Loops in Python
To keep a computer doing useful work we need repetition, looping back over the same block of code again and again. This post will describe the different kinds of loops in Python. For Loop The for loop that is used to iterate over elements of a sequence, it is often used when you have a piece of code which you want to repeat “n” number of time. It works like this: ” for all elements in a list, do this ” Let’s say that you have a list computer_brands = [“Apple”, “Asus”, “Dell”, “Samsung”] for brands in computer_brands: print brands That reads, for every element that we assign the variable brands, in the list computer_brands, print out the variable brands numbers = [1,10,20,30,40,50] sum = 0 for number in numbers: sum = sum + numbers print sum for i in range(1,10): print i Loops in Python Using Break and Continue Statements in Python Python Programming – Python Loops Break To break out of a loop, you can use the keyword “break”. for i in range(1,10): if i == 3: break print i Continue The continue statement is used to tell Python to skip the rest of the statements in the…
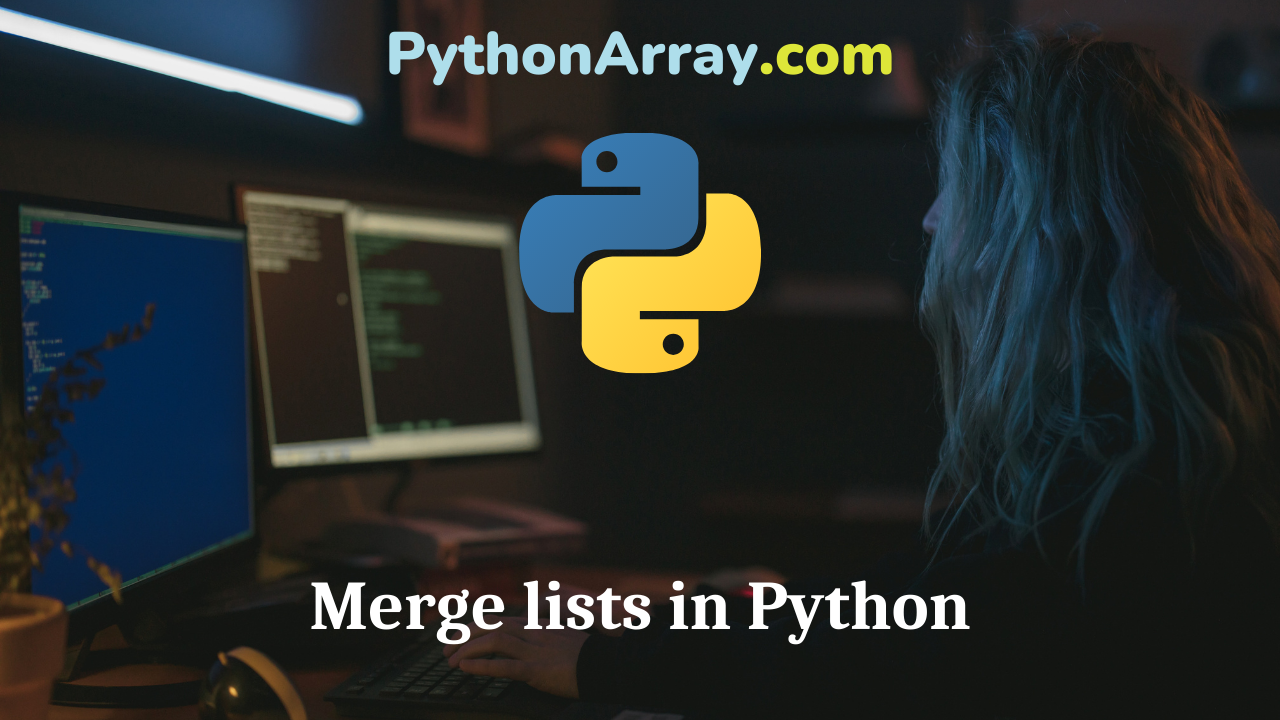
Merge lists in Python
There may be situations while programming where we need to merge two or more lists in python. In this article, we will look at different ways with which we can merge two lists in python. Merge lists using append() method in python We can use the append() method to merge a list into another. The append() method is used to append new elements to an existing list. To merge two lists using the append() method, we will take a list and add elements from another list to the list one by one using a for loop. This can be done as follows. list1=[1,2,3,4] list2=[5,6,7,8] print(“First list is:”) print(list1) print(“Second list is:”) print(list2) for i in list2: list1.append(i) print(“Merged list is:”) print(list1) Output: First list is: [1, 2, 3, 4] Second list is: [5, 6, 7, 8] Merged list is: [1, 2, 3, 4, 5, 6, 7, 8] In the process of merging the lists using append() method, the first input list gets modified as elements from the second input list are added to it. Whereas there is no effect on the second list from which we are taking out elements to append in the first list. Python : List Methods…
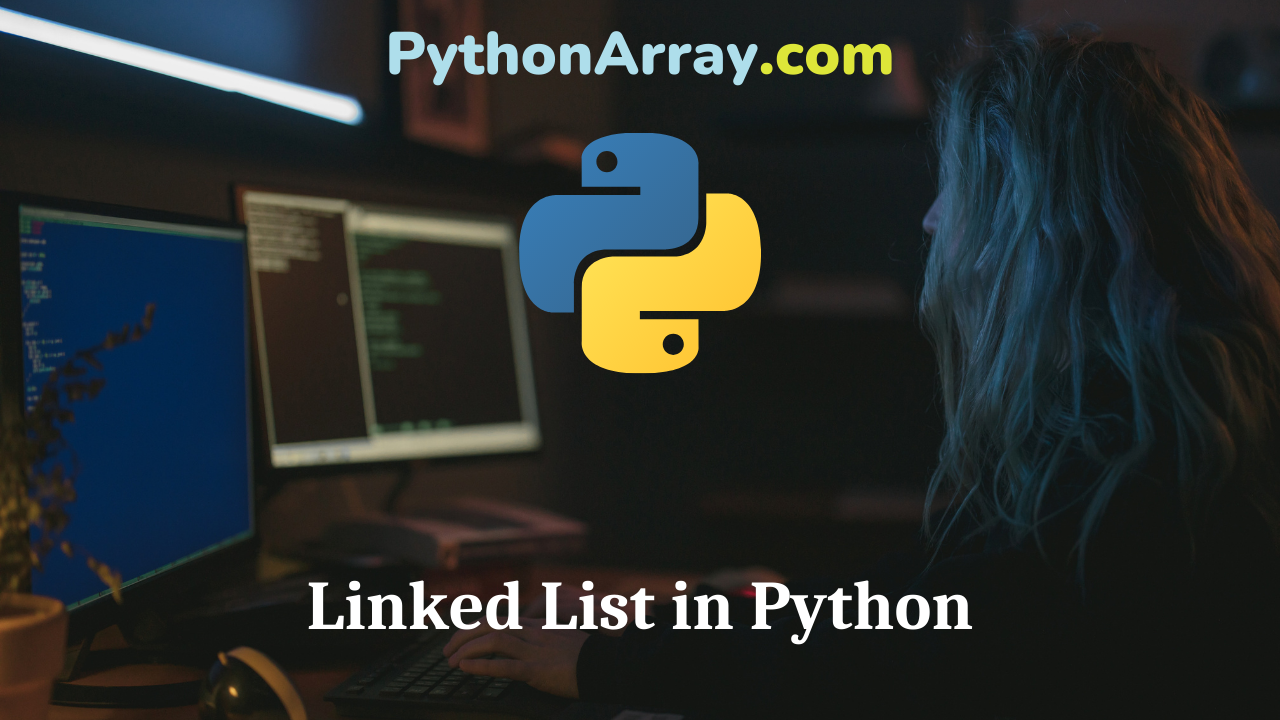
Linked List in Python
Linked list is a data structure which contains data objects which are connected by link. Each linked list consists of nodes which have a data field and a reference to the next node in the linked list. In this article, we will study the underlying concept behind linked list and will implement it in python. What is a node in a linked list? A node is an object which has a data field and a pointer to another node in the linked list. A simple structure of a node can be shown in the following figure. Node of a linked list in python We can implement the above object using a class Node having two fields namely data and next as follows. class Node: def __init__(self,data): self.data=data self.next=None A linked list consists of many such nodes. There is a head pointer in the linked list which points to the first node in the linked list or None when the linked list is empty. The following figure depicts a linked list having three nodes. Linked list in python We can see that the next field of the last node points to None and the reference Head points to the first Node.…