In python programming, we can easily determine that the number is positive, negative, or zero by using different functions and statements. Firstly, get some knowledge on positive numbers and negative numbers along with python if-elif-else statement, nested if statement, for loop, while loop, list comprehensive topics for better understanding of how to check for positive numbers in python. This tutorial of How to Test for Positive Numbers in Python contains the below modules: How to Test for Positive Numbers in Python using if..elif..else statement? Python program to print positive numbers in a list using For Loop Python Program to check if a Number is Positive, Negative or Zero using While Loop Python Code to Test if a number is Positive using List Comprehension Using Nested if Statement to find the Positive Number in Python Python program to print positive numbers in a list Using lambda expressions How to Test for Positive Numbers in Python using if..elif..else statement? Here, we have written a simple and straightforward code to work with any integer or number. In this section, we are testing the inputted number is positive or negative or zero in python using an if…elif…else statement. If the given number is greater…
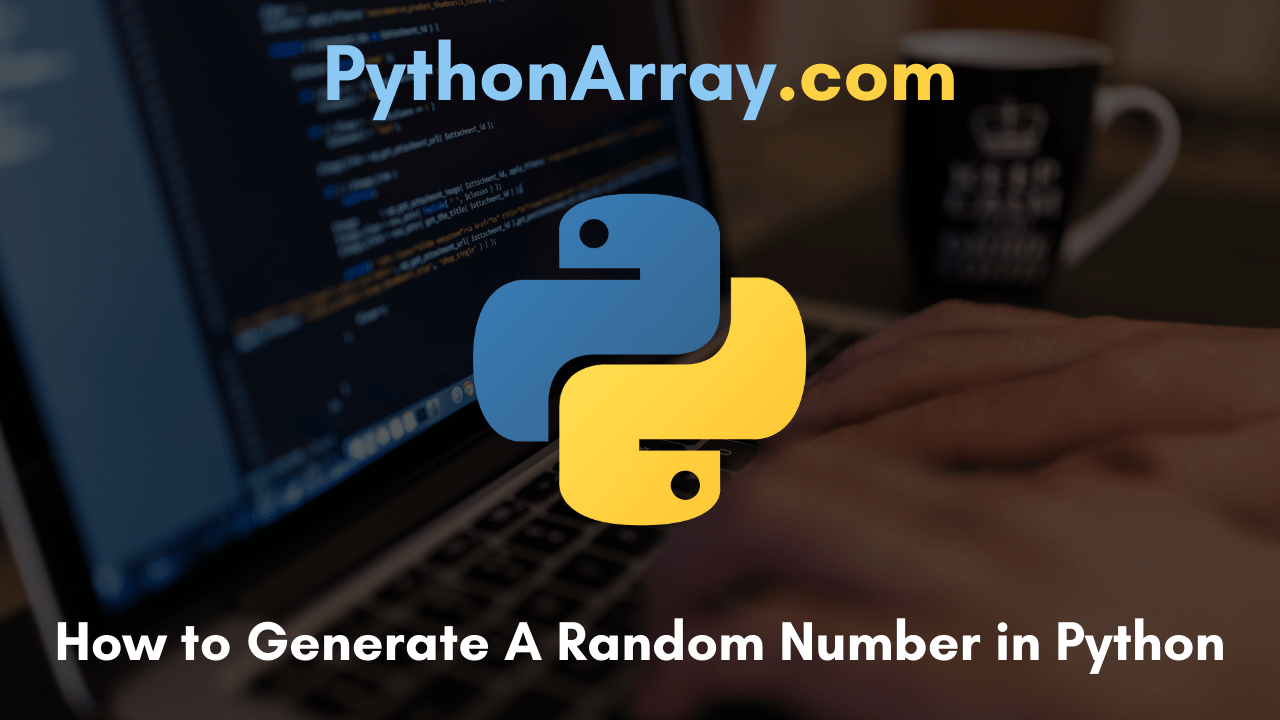
How to Generate a Random Number in Python | Python Program to Generate Random Numbers
Beginners or python experts can learn complete knowledge on how to generate a random number in python from this tutorial. Having knowledge of these Python programming topics like Python Input, Output, and Import, Python Random Module is the best way to understand the concept of Python Random Numbers Generation. If you don’t know the coding part of generating random numbers in python, then you’re not more called a python coder. This tutorial of Python Program to Generate Random Numbers include the following modules: Random Numbers in Python Syntax for Generating Random Number Generating a Single Random Number How to Generate a Random Number in Python Example Python Program to Generate a Random Number Generating Number in a Range Generating a List of Numbers Using For Loop Generate Random Numbers in Python Using random.sample() Random Number Operations Random Numbers in Python Defining a set of functions in python which are utilized to generate or manipulate random numbers via the random module. The random module functions completely rely on a pseudo-random number generator function random(), which generates a random float number between 0.0 and 1.0. In various things such as games, lotteries, or any application requiring a random number generation, we can…
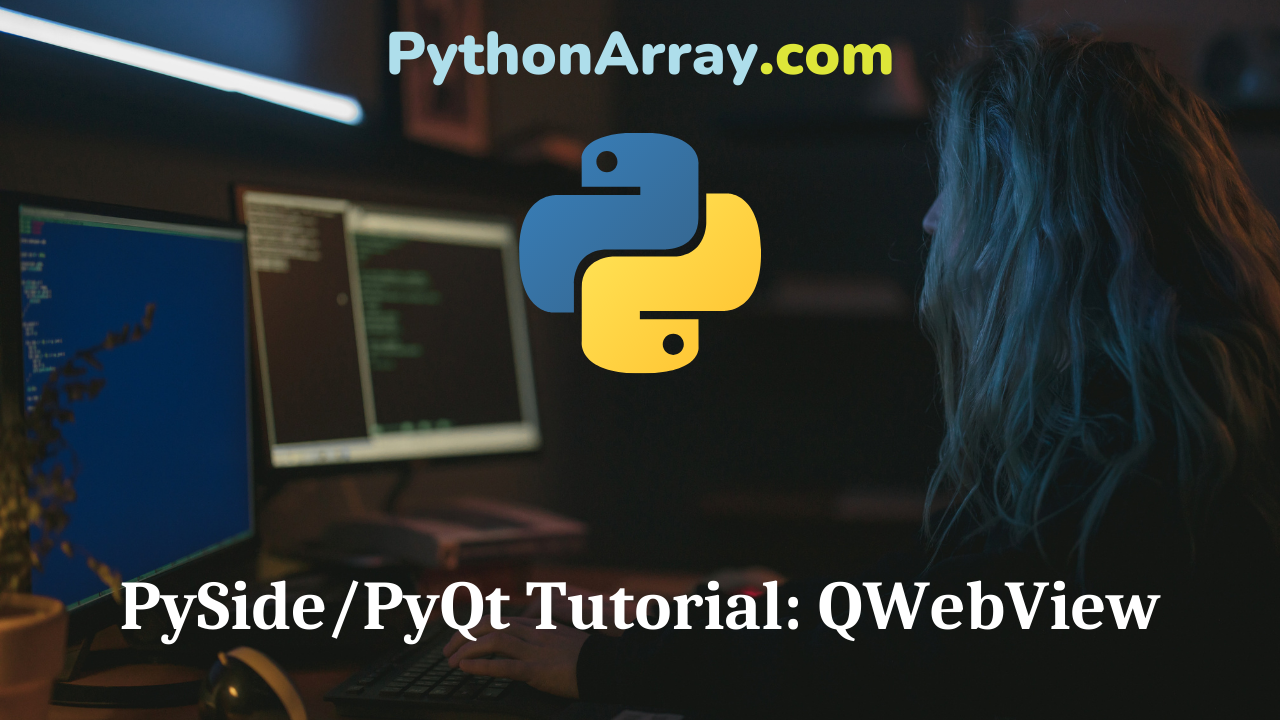
PySide/PyQt Tutorial: QWebView
The QWebView is a highly useful control; it allows you to display web pages from URLs, arbitrary HTML, XML with XSLT stylesheets, web pages constructed as QWebPages, and other data whose MIME types it knows how to interpret. It uses the WebKit web browser engine. WebKit is an up-to-date, standards-compliant rendering engine used by Google’s Chrome, Apple’s Safari, and soon the Opera browser. Creating and Filling a QWebView Content from a QWebView’s URL You instantiate a QWebView like any othe QWidget, with an optional parent. There are a number of ways to put content into it, however. The simplest — and possibly the most obvious — is its load method, which takes a QUrl; the simplest way to construct a QUrl is with a unicode URL string: web_view.load(QUrl(‘http://www.www.pythoncentral.io’)) That loads Python Central’s main page in the QWebView control. Equivalent would be to use the setUrl method, like so: web_view.setUrl(QUrl(‘http://www.www.pythoncentral.io’)) HTML Parser: How to scrape HTML content | Parsing HTML in Python with BeautifulSoup Writing Views to Upload Posts for Your First Python Django Application How to Use Python Django Forms Load Arbitrary HTML with QWebView There are other interesting ways to load content into a QWebView. You can load generated HTML into it using the setHtml method. For example, you can do something like this: html = ”'<html> <head> <title>A…
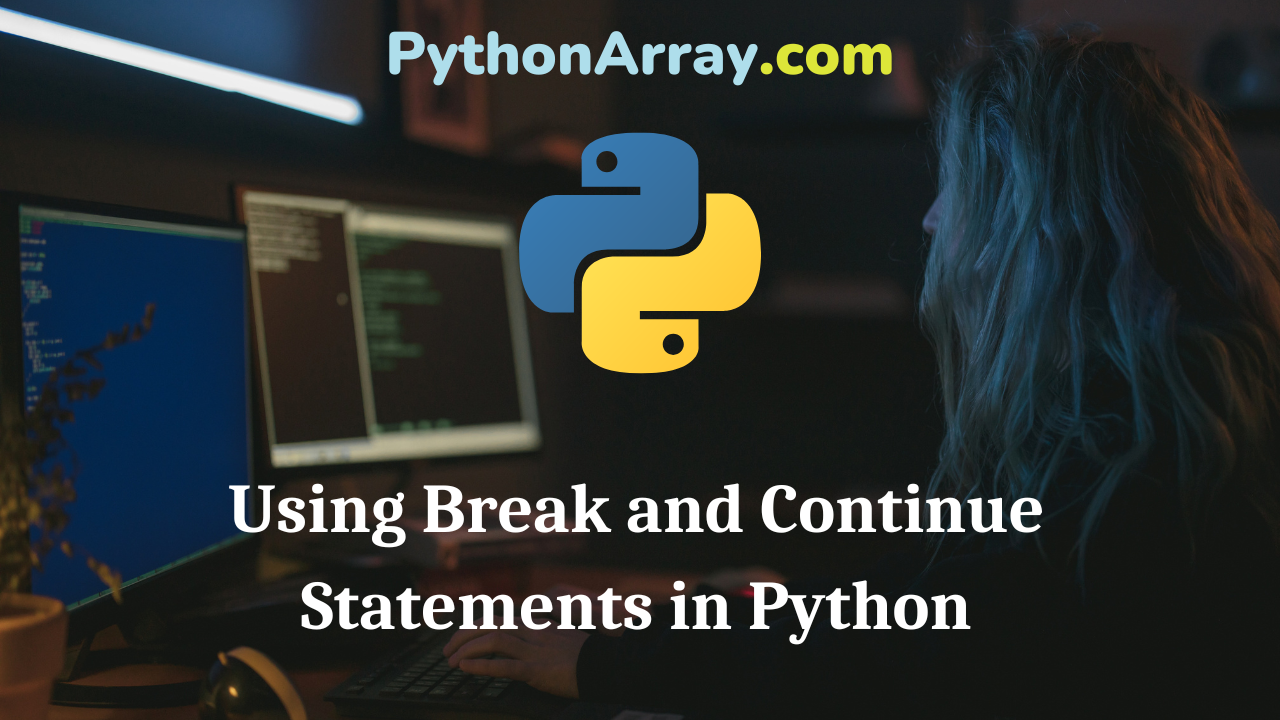
Using Break and Continue Statements in Python
In Python, break statements are used to exit (or “break) a conditional loop that uses “for” or “while”. After the loop ends, the code will pick up from the line immediately following the break statement. Here’s an example: even_nums = (2, 4, 6) num_sum = 0 count = 0 for x in even_nums: num_sum = num_sum + x count = count + 1 if count == 4 break In the example above, the code will break when the count variable is equal to 4. Python Programming – Python Control Statements Python Programming – Python Loops Python While Loop The continue statement is used to skip over certain parts of a loop. Unlike break, it doesn’t cause a loop to be ended or exited, but rather it allows for certain iterations of the loop to be omitted, like this: for y in range(7) if (y==5): continue print(y) In this example, all iterations of the loop (numbers 0-7) will be printed except for the number 5, because by using the continue statement the loop was instructed to skip over y when it was equal to 5. Practice on your own to see how you can use the break and continue statements. Understanding the difference and the…
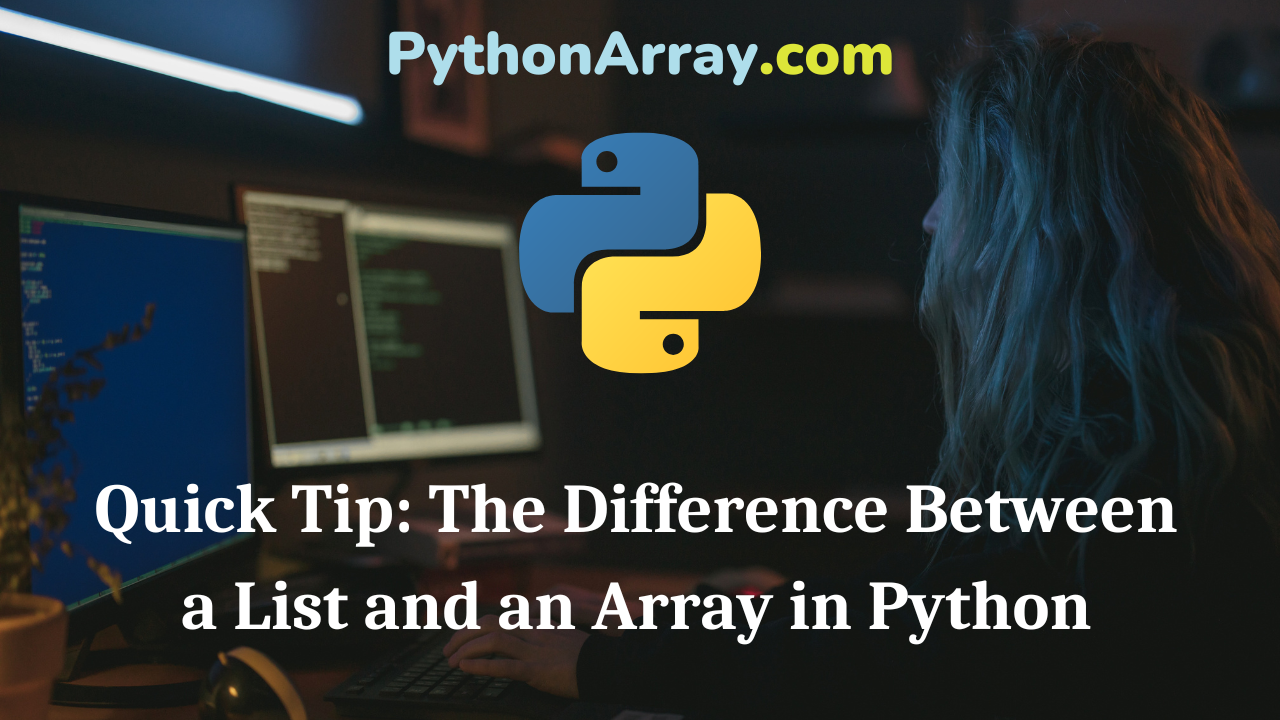
Quick Tip: The Difference Between a List and an Array in Python
Arrays and lists are both used in Python to store data, but they don’t serve exactly the same purposes. They both can be used to store any data type (real numbers, strings, etc), and they both can be indexed and iterated through, but the similarities between the two don’t go much further. The main difference between a list and an array is the functions that you can perform to them. For example, you can divide an array by 3, and each number in the array will be divided by 3 and the result will be printed if you request it. If you try to divide a list by 3, Python will tell you that it can’t be done, and an error will be thrown. Python Programming – Introduction To Numpy Python Programming – Array Creation Python Programming – Arithmetic Operations On Array Here’s how it would work: x = array([3, 6, 9, 12]) x/3.0 print(x) In the above example, your output would be: array([1, 2, 3, 4]) If you tried to do the same with a list, it would very similar: y = [3, 6, 9, 12] y/3.0 print(y) It’s almost exactly like the first example, except you wouldn’t get a valid…
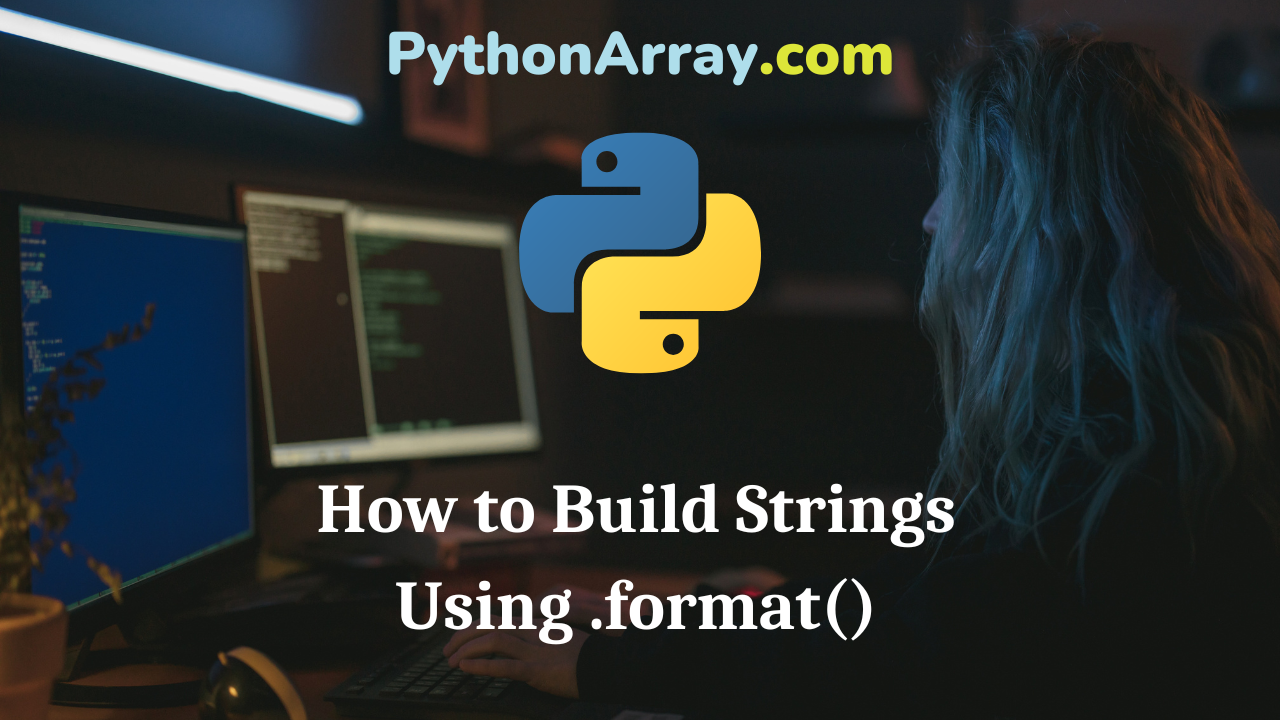
How to Build Strings Using .format()
Often developers try to build strings in Python by concatenating a bunch of strings together to make one long string, which definitely works but isn’t exactly ideal. Python’s .format() method allows you to perform essentially the same effect as concatenating strings (adding a bunch of strings together) but does so in a much more efficient and lightweight manner. Let’s say you want to add together the following strings to create a sentence: name = “Jon” age = 28 fave_food = “burritos” fave_color = “green” To print the strings above you always have the option to concatenate them, like this: string = “Hey, I’m ” + name + “and I’m ” + str(age) + ” years old. I love ” + fave_food + ” and my favorite color is ” + fave_color “.” print(string) Python Programming – Special String Operators Python Programming – Python Strings Python Basics: Strings and Printing OR you can create your string by using the .format() method, like this: string = “Hey, I’m {0} and I’m {1} years old. I love {2} and my favorite color is {3}.”.format(name, age, fave_food, fave_color) print(string) Your output for both of the above examples would be the same: Hey, I’m Jon…
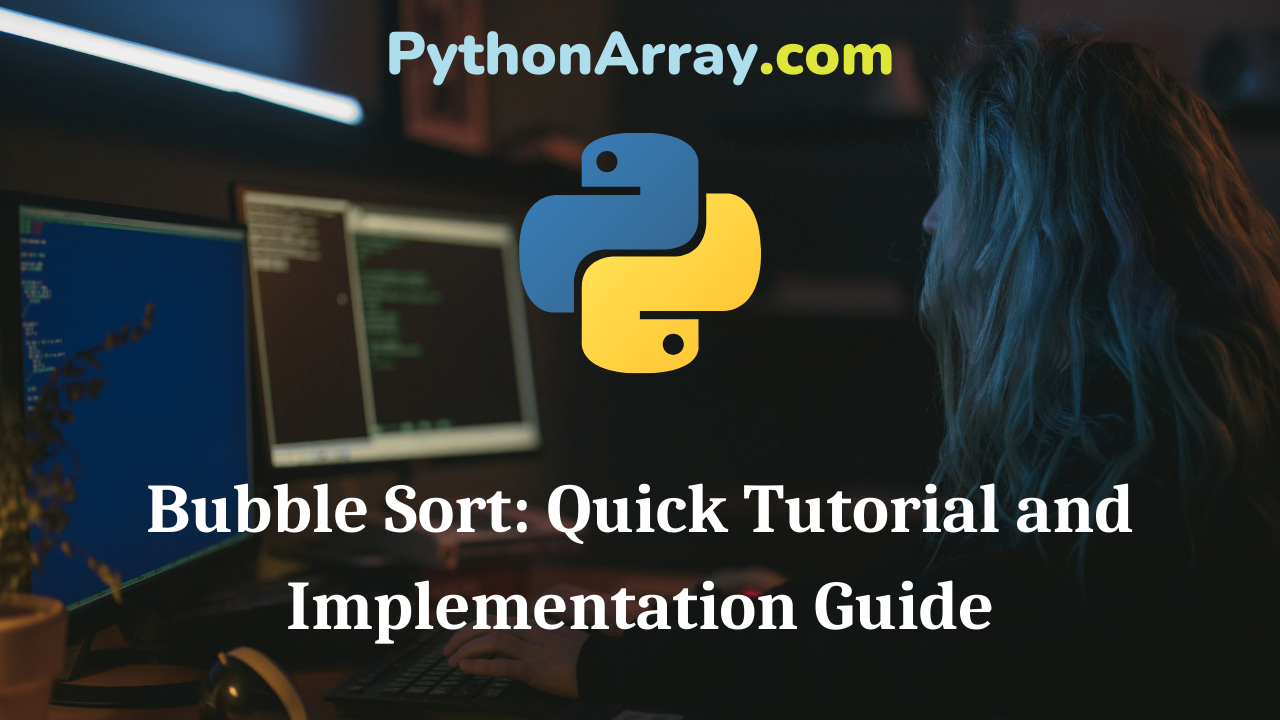
Bubble Sort: Quick Tutorial and Implementation Guide
Prerequisites T0 learn about Bubble Sort, you must know: Python 3 Python data structures – Lists What is Bubble Sort? In the last tutorial, we saw how to search for an element from unsorted and sorted lists. We discovered that search efficiency is greater when the lists are sorted. So how do we sort a list? In this tutorial and in the tutorials to follow we are going to examine some common algorithms for list sorting. Each algorithm has its pros and cons which we will discuss as we go. How to Sort Python Dictionaries by Key or Value Python Programming – Python Native Data Types How to Sort a List, Tuple or Object (with sorted) in Python Note: All these algorithms focus on sorting the list in ascending order (descending order is considered unsorted). First, let’s look at Bubble Sort, one of the most basic and simple algorithms. It may not be the most efficient, but it is very easy to implement. A bubble sort takes in an unsorted list and keeps comparing each element with its right side neighbour in order to sort the data. Whichever element is smaller gets shifted to the left. After completion of one…
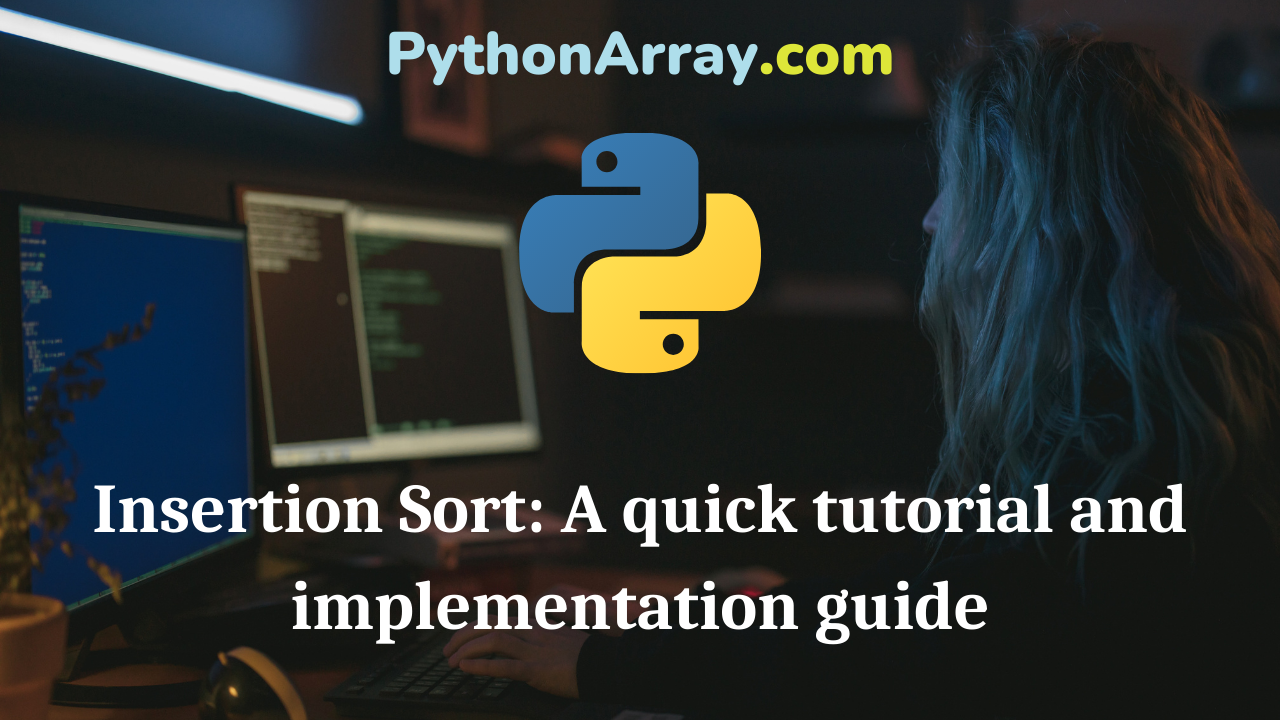
Insertion Sort: A quick tutorial and implementation guide
Prerequisites To learn about Insertion Sort, you must know: Python 3 Python data structures – Lists What is Insertion Sort? We are in the second tutorial of the sorting series. The previous tutorial talks about Bubble Sort which is a very simple sorting algorithm. If you haven’t read that, you can find it here. Like all sorting algorithms, we consider a list to be sorted only if it is in ascending order. Descending order is considered the worst unsorted case. As the name suggests, in Insertion Sort, an element gets compared and inserted into the correct position in the list. To apply this sort, you must consider one part of the list to be sorted and the other to be unsorted. To begin, consider the first element to be the sorted portion and the other elements of the list to be unsorted. Now compare each element from the unsorted portion with the element/s in the sorted portion. Then insert it in the correct position in the sorted part. Remember, the sorted portion of the list remain sorted at all times. Let us see this using an example, alist = [5,9,1,2,0] Round 1: sorted – 5 and unsorted – 9,1,2,0 5<9: nothing…
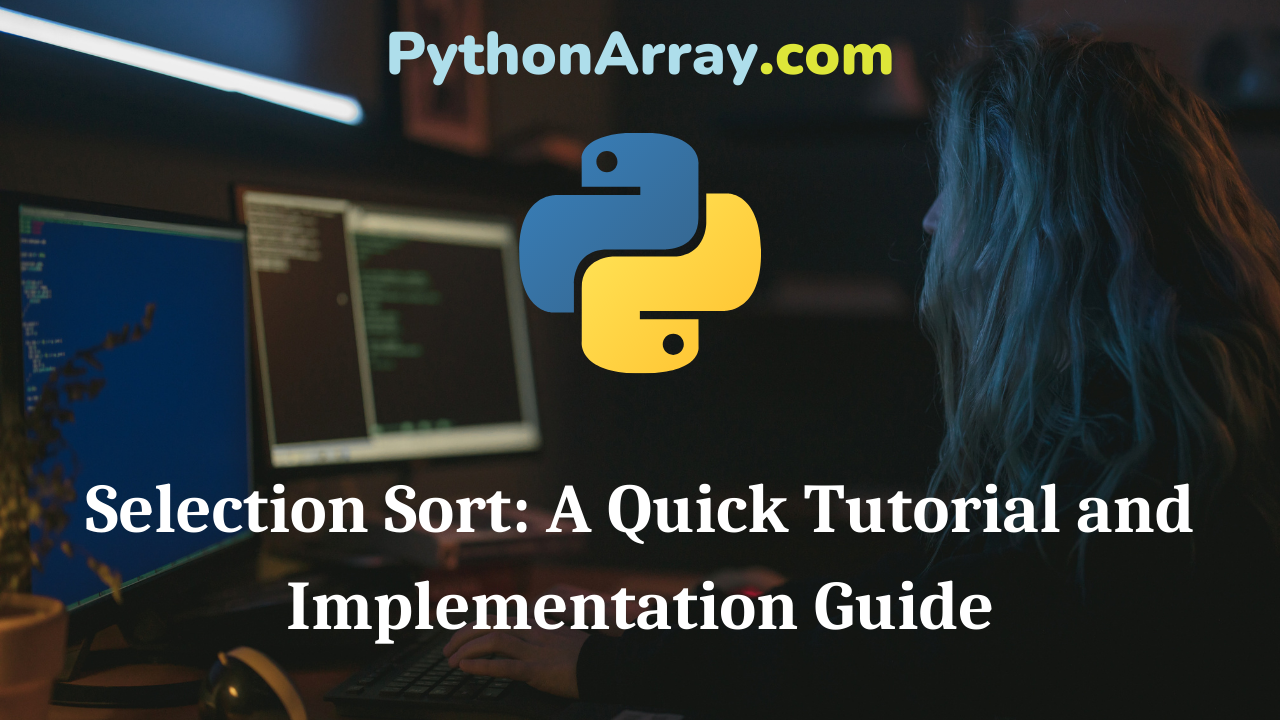
Selection Sort: A Quick Tutorial and Implementation Guide
Prerequisites To learn about Selection Sort, you must know: Python 3 Python data structures – Lists What is Selection Sort? We are in the third tutorial of the sorting series. The previous tutorial talks about Bubble Sort and Insertion Sort. If you haven’t read that, please do as we will be building off of those concepts. Like all sorting algorithms, we consider a list to be sorted only if it is in ascending order. Descending order is considered the worst unsorted case. Similar to Insertion Sort, we begin by considering the first element to be sorted and the rest to be unsorted. As the algorithm proceeds, the sorted portion of the list will grow and the unsorted portion will continue to shrink. Selection Sort is about picking/selecting the smallest element from the list and placing it in the sorted portion of the list. Initially, the first element is considered the minimum and compared with other elements. During these comparisons, if a smaller element is found then that is considered the new minimum. After completion of one full round, the smallest element found is swapped with the first element. This process continues till all the elements are sorted. Bubble Sort: Quick…
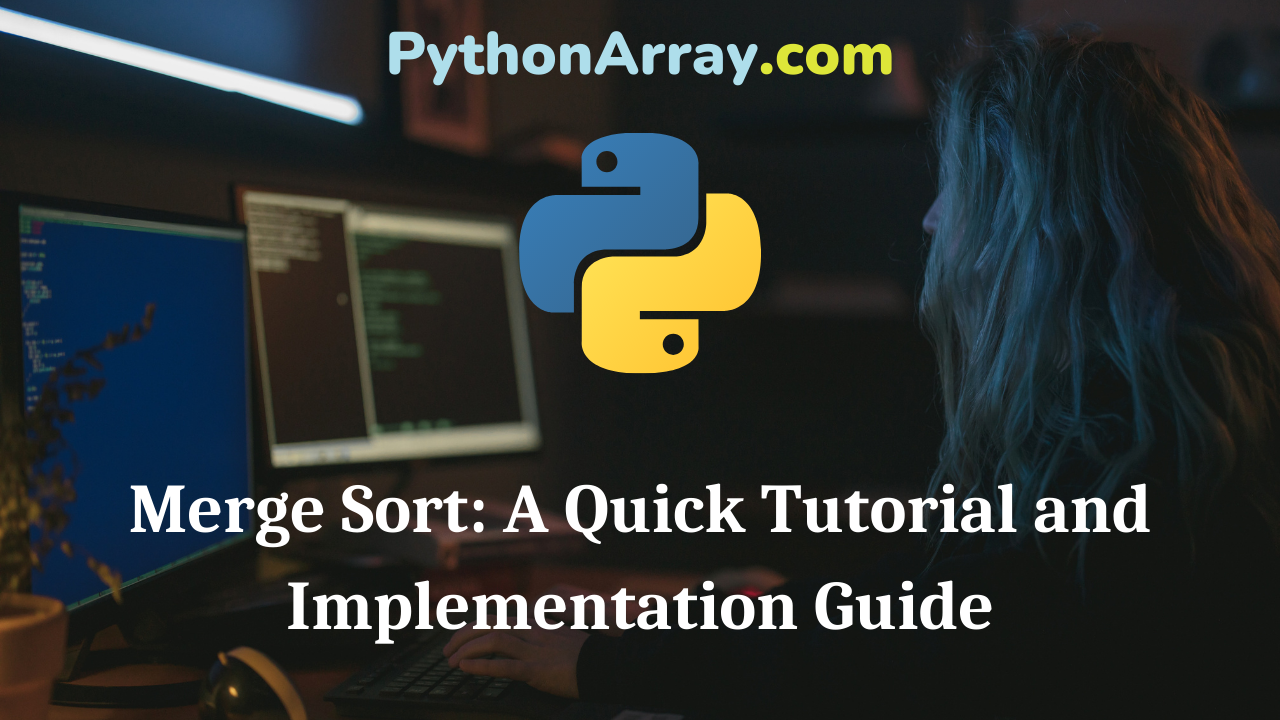
Merge Sort: A Quick Tutorial and Implementation Guide
Prerequisites To learn about Merge Sort, you must know: Python 3 Python data structures – Lists Recursion What is Merge Sort? We are in the fourth tutorial of the sorting series. The previous tutorials cover Bubble Sort, Insertion Sort, and Selection Sort. If you haven’t read these, please do as we will be building off of those concepts. Like all sorting algorithms, we consider a list to be sorted only if it is in ascending order. Descending order is considered the worst unsorted case. Merge sort is very different than the other sorting techniques we have seen so far. Merge Sort can be used to sort an unsorted list or to merge two sorted lists. Insertion Sort: A quick tutorial and implementation guide Bubble Sort: Quick Tutorial and Implementation Guide Python Programming – Python Native Data Types Sort an unsorted list The idea is to split the unsorted list into smaller groups until there is only one element in a group. Then, group two elements in the sorted order and gradually build the size of the group. Every time the merging happens, the elements in the groups must be compared one by one and combined into a single list in sorted order. This…