Sorting The easiest way to sort is with the sorted(list) function, which takes a list and returns a new list with those elements in sorted order. The original list is not changed. The sorted() function can be customized though optional arguments. The sorted() optional argument reverse=True, e.g. sorted(list, reverse=True), makes it sort backwards. Python Lists Cheat Sheet Python Programming – List Functions And Methods How to Sort Python Dictionaries by Key or Value Examples Sorting Examples Create a list with some numbers in it numbers = [5, 1, 4, 3, 2, 6, 7, 9] prints the numbers sorted print sorted(numbers) the original list of numbers are not changed print numbers my_string = [‘aa’, ‘BB’, ‘zz’, ‘CC’, ‘dd’, “EE”] if no argument is used, it will use the default (case sensitive) print sorted(my_string) using the reverse argument, will print the list reversed print sorted(strs, reverse=True) ## [‘zz’, ‘aa’, ‘CC’, ‘BB’] More Reading Please see the python wiki for more things to do with sorting.
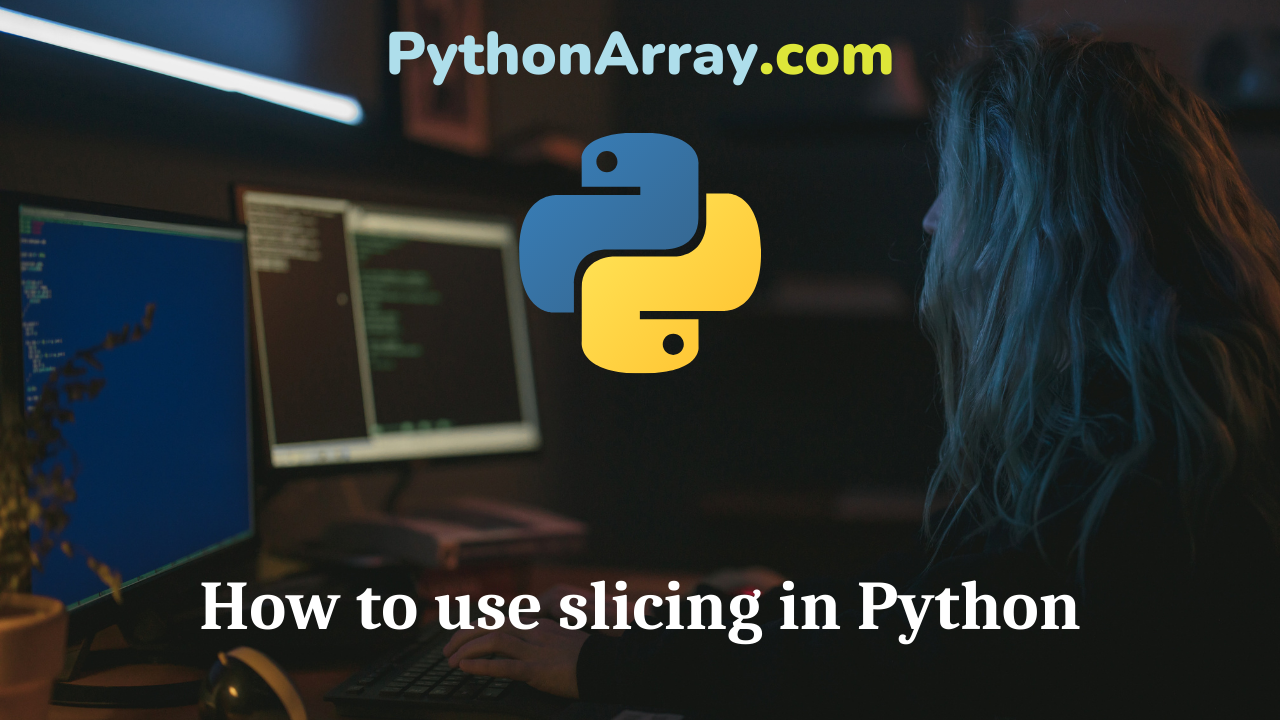
How to use slicing in Python
Slicing in Python When you want to extract part of a string, or some part of a list, you use a slice The first character in string x would be x[0] and the nth character would be at x[n-1]. Python also indexes the arrays backwards, using negative numbers. The last character has index -1, the second to last character has index -2. Python Programming – String Slices Cutting and slicing strings in Python Python Programming – Accessing Lists Example x = “my string” x[start:end] # items start through end-1 x[start:] # items start through the rest of the list x[:end] # items from the beginning through end-1 x[:] # a copy of the whole list One way to remember how slices work is to think of the indices as pointing betweencharacters, with the left edge of the first character numbered 0. Then the right edge of the last character of a string of n characters has index n +—+—+—+—+—+ | H | e | l | p | A | +—+—+—+—+—+ 0 1 2 3 4 5 -5 -4 -3 -2 -1 The first row of numbers gives the position of the indices 0…5 in the string, the second row…
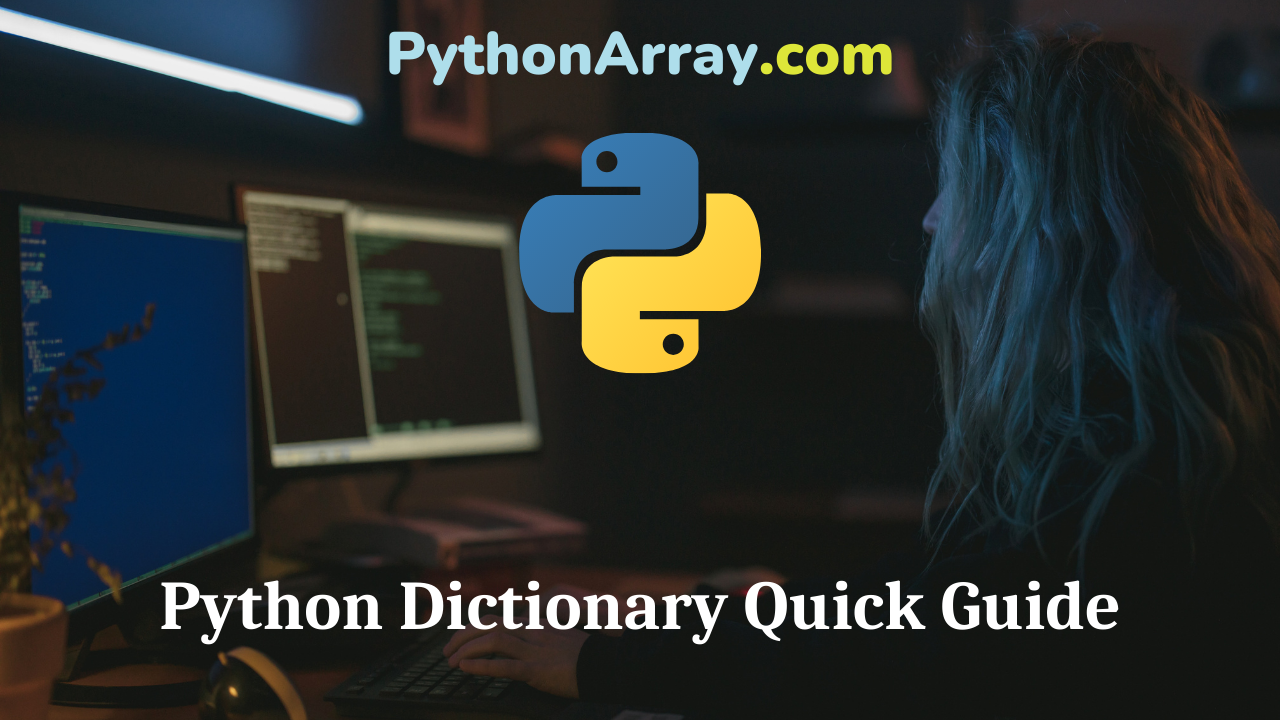
Python Dictionary Quick Guide
As the title says, this is a Python dictionary quick guide. Please check out the Dictionary Tutorial for more articles about dictionaries. What is a Dictionary in Python? Python Programming – Creation, Initialization and Accessing The Elements In A Dictionary How to Sort Python Dictionaries by Key or Value # key/value pairs declaration dict = { ‘key1′:’value1′, ‘key2′:’value2′, ‘key3′:’value3′ } #Get all keys dict.keys() #Get all values dict.values() #Modifying dict[‘key2’] = ‘value8′ #Accessing print dict[‘key1’] # prints ‘value2′ print dict[‘key2’] # empty declaration + assignment of key-value pair emptyDict = {} emptyDict[‘key4’]=’value4′ # looping through dictionaries (keys and values) for key in dict: print dict[key] # sorting keys and accessing their value in order keys = dict.keys() keys.sort() for key in keys: print dict[key] # looping their values directory (not in order) for value in dict.values(): print value # getting both the keys and values at once for key,value in dict.items(): print “%s=%s” % (key,value) # deleting an entry del dict[‘key2’] # delete all entries in a dictionary dict.clear() # size of the dictionary len(dict)
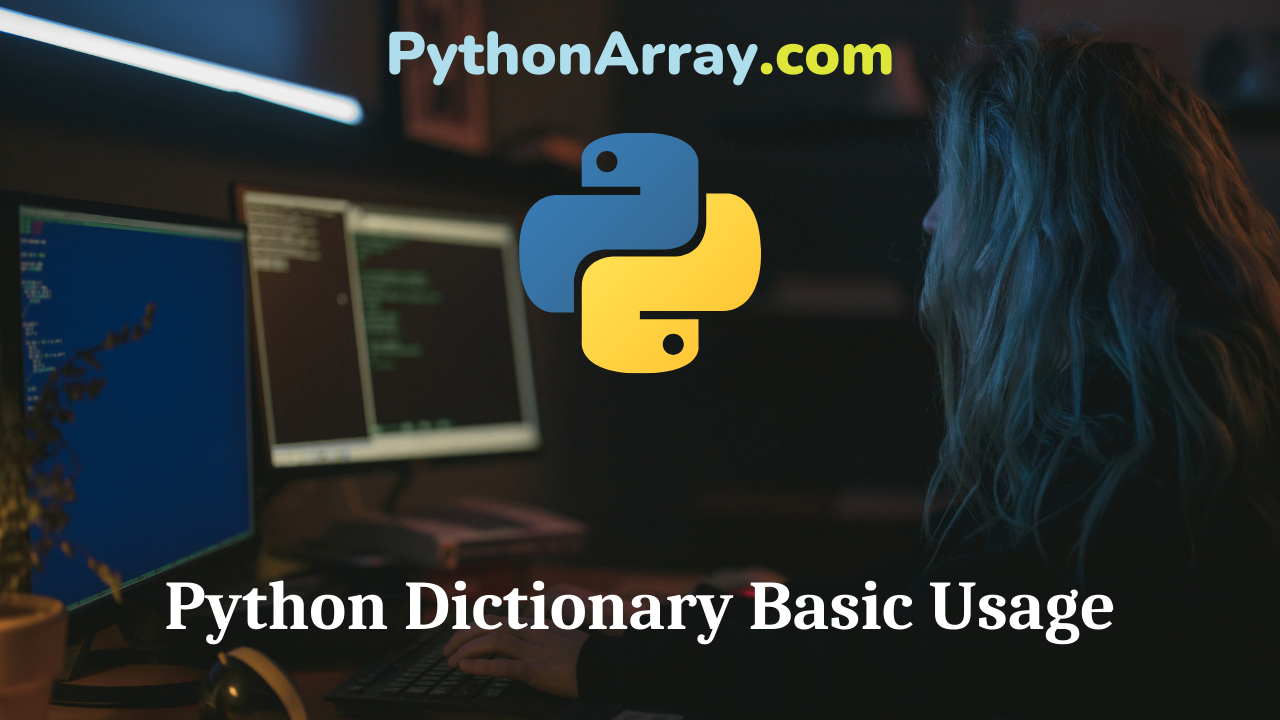
Python Dictionary Basic Usage
Dictionary is another data type in Python. Python Programming – Dictionaries Dictionary Comprehension in Python Python Programming – Dictionary Functions and Methods Dictionary Overview A dictionary maps a set of key to a set of values. Dictionaries in Python are also known as hash tables. It requires that the keys are unique (within one dictionary), while the values maynot. The values of a dictionary can be of any type, but the keys must be of an immutable data type such as strings, numbers, or tuples. Dictionaries are like lists mutable A dictionary is created with a pair of braces {}. For example, dic = {} creates an empty dictionary. Each key is separated from its value by a colon (:) Placing a comma-separated list of key:value pairs within the braces adds initial key:value pairs to the dictionary; this is also the way dictionaries are written on output. The main operations on a dictionary are storing a value with some key and extracting the value given the key. If you store using a key that is already in use, the old value associated with that key is forgotten. Don’t forget to check out the Dictionary Cheat Sheet
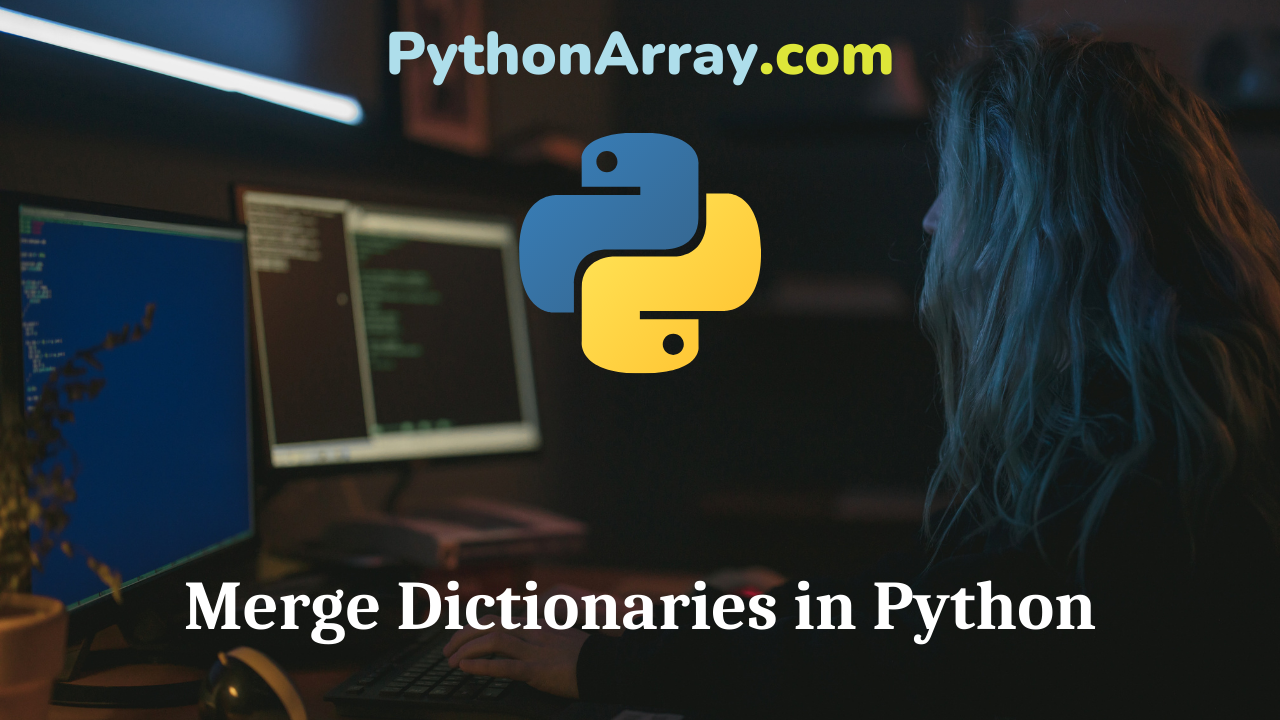
Merge Dictionaries in Python
While programming, there may be situations where we need to merge two or more dictionaries in python. In this article, we will study how we can merge two or more dictionaries in python using different ways. Merge dictionaries using items() method. We can merge two dictionaries using items() method by adding items of a dictionary to another dictionary one by one. The items() method when invoked on a dictionary, returns a list of tuples containing the key-value pair. If we have to merge two dictionaries, we will take items of a dictionary one by one and add it to another dictionary as follows. dict1={1:1,2:4,3:9} print(“First dictionary is:”) print(dict1) dict2={4:16,5:25,6:36} print(“Second dictionary is:”) print(dict2) for key,value in dict2.items(): dict1[key]=value print(“Merged dictionary is:”) print(dict1) Output: First dictionary is: {1: 1, 2: 4, 3: 9} Second dictionary is: {4: 16, 5: 25, 6: 36} Merged dictionary is: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36} While using the above method, we have to consider the point that if there are common keys between the two dictionaries, the final dictionary will have common keys with values from the second dictionary from which we have extracted the items. Also only the…
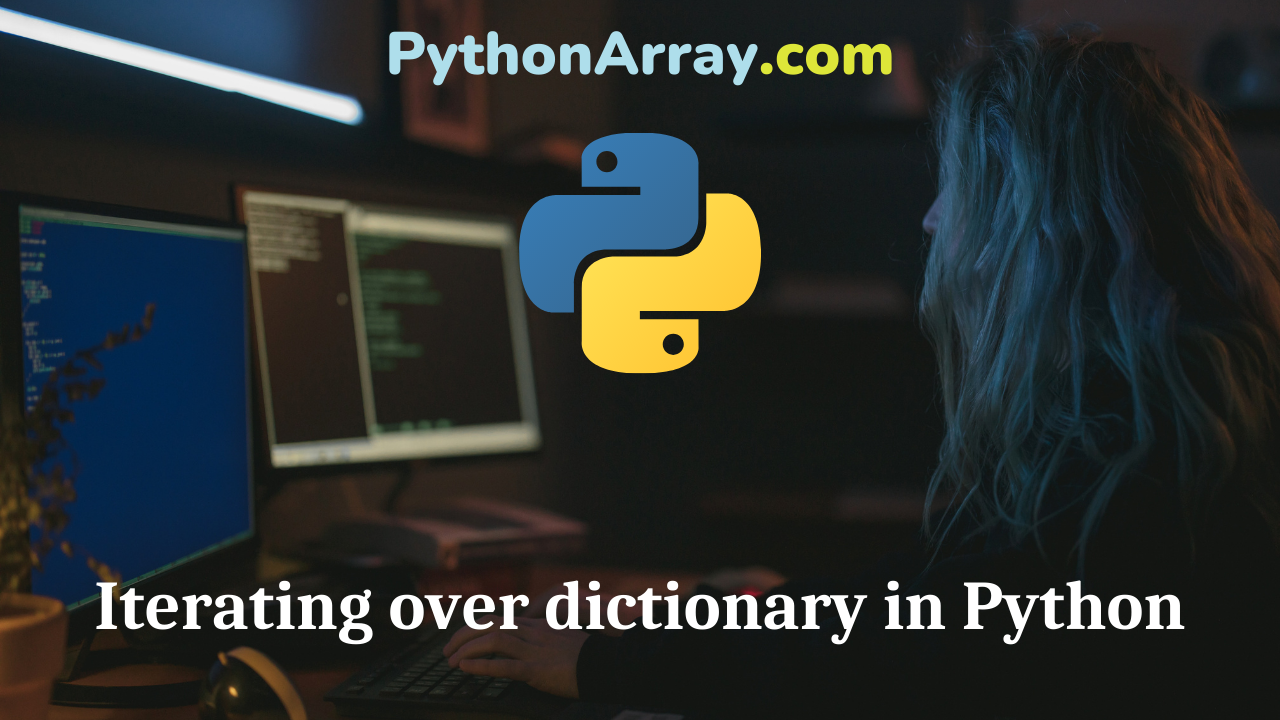
Iterating over dictionary in Python
Dictionaries are one of the most frequently used data structures in python. It contains data in the form of key-value pairs. While processing the data with dictionaries, we may need to iterate over the items in the dictionary to change the values or read the values present in the dictionary. In this article, we will see various ways for iterating over a dictionary in python. Iterating over a dictionary using for loop As we iterate through lists or tuples using for loops in python, we can also iterate through a python dictionary using a for loop. When we try to iterate over a dictionary using for loop,it implicitly calls __iter__() method. The __iter__() method returns an iterator with the help of which we can iterate over the entire dictionary. As we know that dictionaries in python are indexed using keys, the iterator returned by __iter__() method iterates over the keys in the python dictionary. So, with a for loop, we can iterate over and access all the keys of a dictionary as follows. myDict={“name”:”PythonForBeginners”,”acronym”:”PFB”,”about”:”Python Tutorials Website”} print(“The dictionary is:”) print(myDict) print(“The keys in the dictionary are:”) for x in myDict: print(x) Output: The dictionary is: {‘name’: ‘PythonForBeginners’, ‘acronym’: ‘PFB’, ‘about’: ‘Python Tutorials Website’} The keys in…
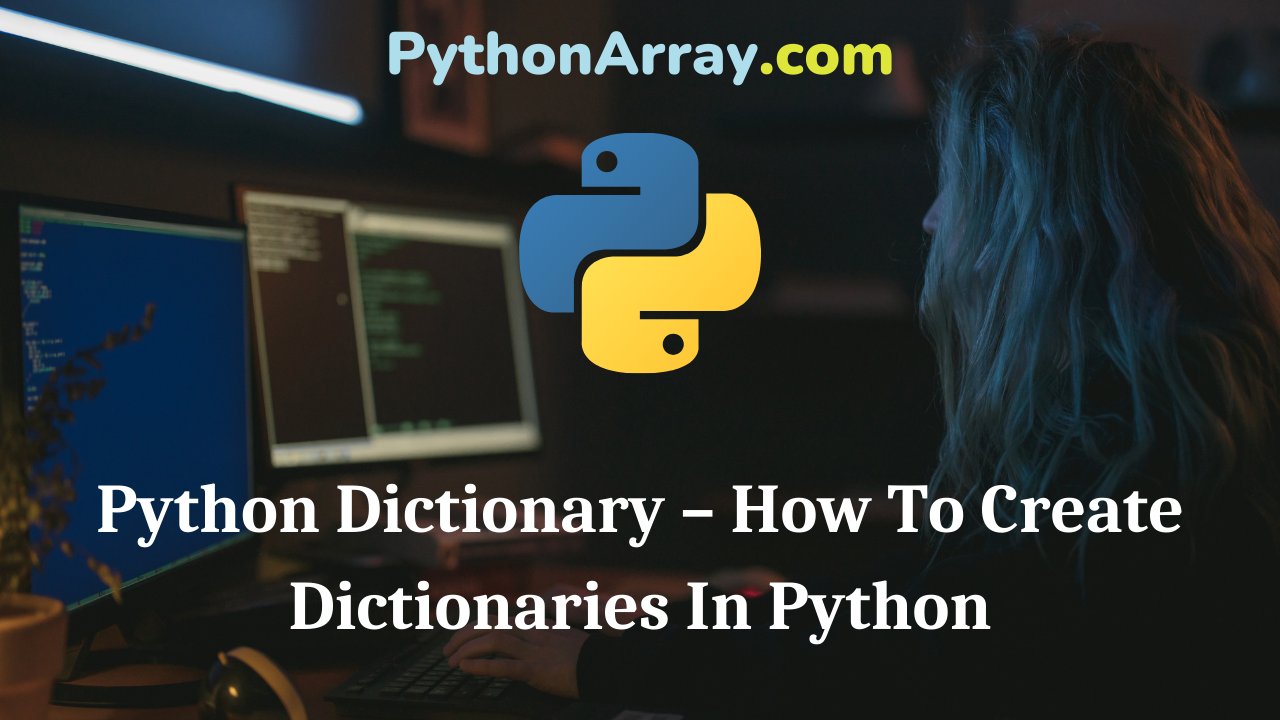
Python Dictionary – How To Create Dictionaries In Python
Dictionaries in Python are a list of items that are unordered and can be changed by use of built in methods. Dictionaries are used to create a map of unique keys to values. About Dictionaries in Python To create a Dictionary, use {} curly brackets to construct the dictionary and [] square brackets to index it. Separate the key and value with colons : and commas, between each pair. Keys must be quoted, for instance: “title” : “How to use Dictionaries in Python” As with lists we can print out the dictionary by printing the reference to it. A dictionary maps a set of objects (keys) to another set of objects (values) so you can create an unordered list of objects. Dictionaries are mutable, which means they can be changed. The values that the keys point to can be any Python value. Dictionaries are unordered, so the order that the keys are added doesn’t necessarily reflect what order they may be reported back. Because of this, you can refer to a value by its key name. Create a new dictionary # In order to construct a dictionary you can start with an empty one. >>> mydict={} # This will create…
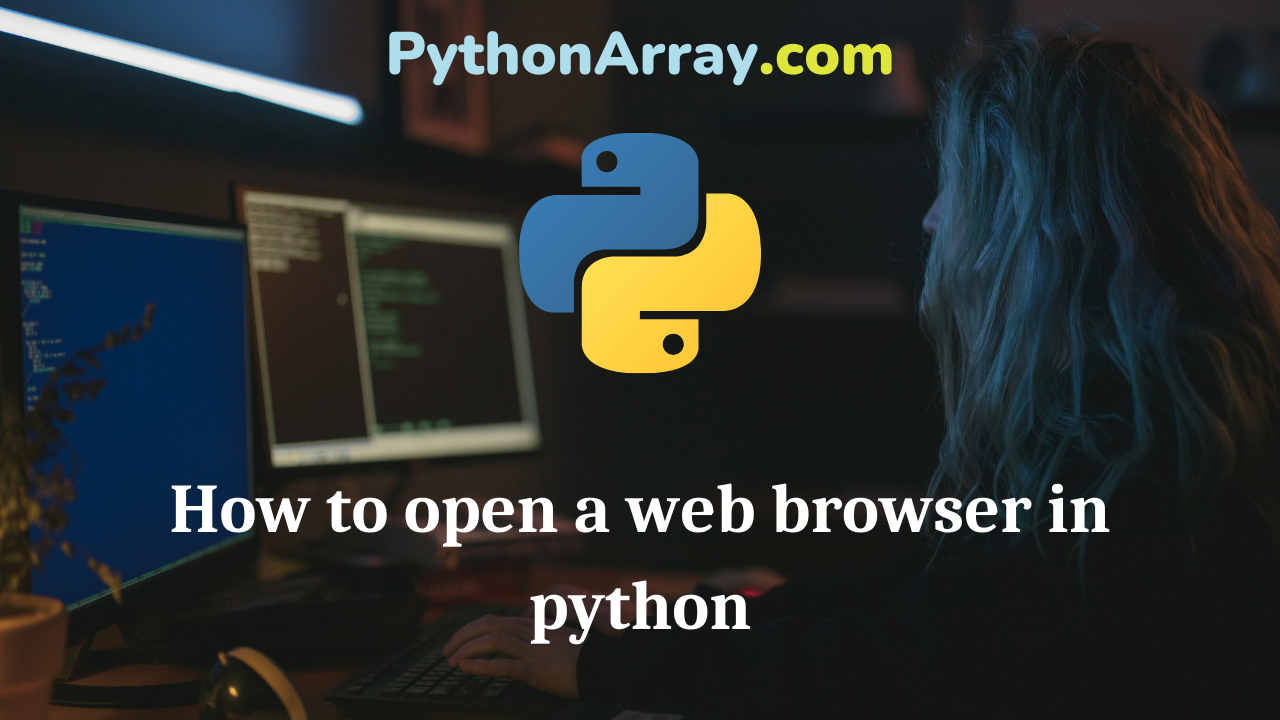
How to open a web browser in python
The webbrowser module in Python provides an interface to display Web-based documents. Webbrowser Under most circumstances, simply calling the open() function from this module will do the right thing. IN Unix, graphical browsers are preferred under X11, but text-mode browsers will be used if graphical browsers are not available or an X11 display isn’t available. If text-mode browsers are used, the calling process will block until the user exits the browser. Python Programming – Introduction to Selenium Python Mechanize Cheat Sheet Loops in Python Usage Examples webbrowser.open_new(url) Open url in a new window of the default browser, if possible, otherwise, open url in the only browser window. webbrowser.open_new_tab(url) Open url in a new page (“tab”) of the default browser, if possible, otherwise equivalent to open_new(). Webbrowser Script This example will ask the user to enter a search term. A new browser tab will open with the search term in googles search field. import webbrowser google = raw_input(‘Google search:’) webbrowser.open_new_tab(‘http://www.google.com/search?btnG=1&q=%s’ % google) The script webbrowser can be used as a command-line interface for the module. It accepts an URL as the argument. It accepts the following optional parameters: -n opens the URL in a new browser window -t opens the URL…
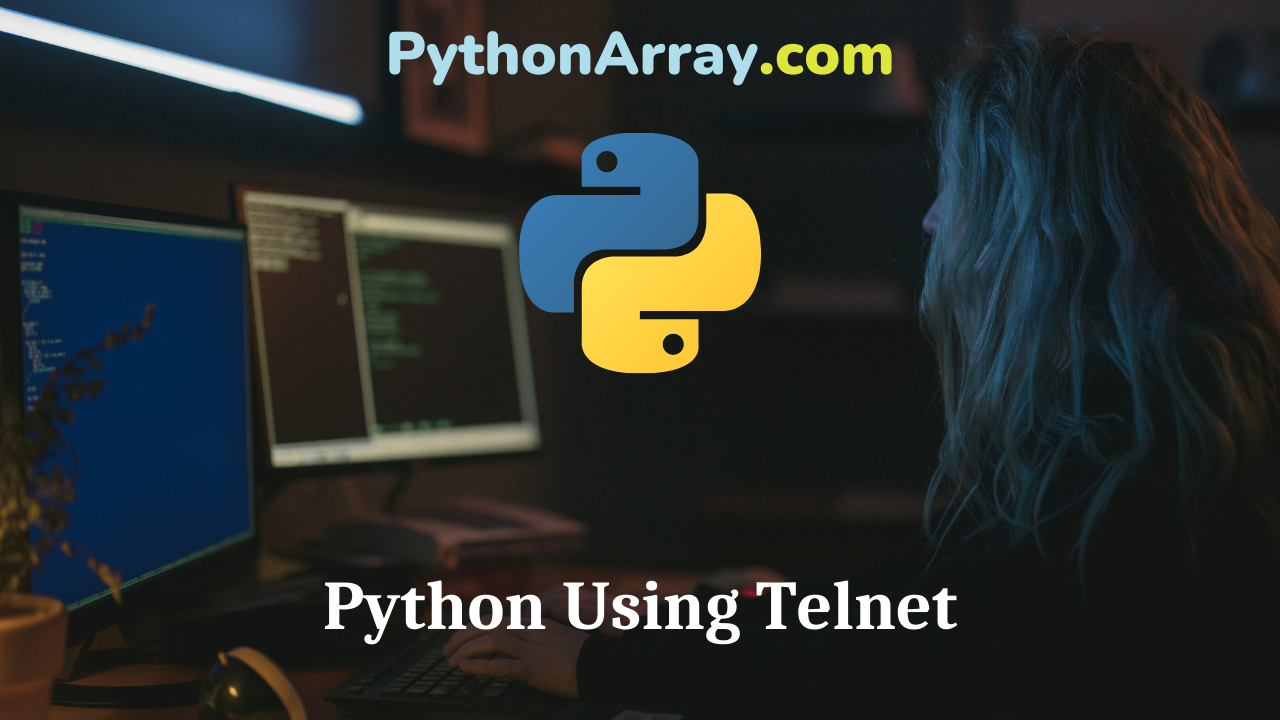
Python Using Telnet
Using Telnet in Python To make use of Telnet in Python, we can use the telnetlib module. That module provides a Telnet class that implements the Telnet protocol. The Telnet module have several methods, in this example I will make use of these: read_until, read_all() and write() How to use ConfigParser in Python Python Code Examples Python Snippets: How to Generate Random String | Python Program for Generation Random String or Password Telnet Script in Python Let’s make a telnet script import getpass import sys import telnetlib HOST = “hostname” user = raw_input(“Enter your remote account: “) password = getpass.getpass() tn = telnetlib.Telnet(HOST) tn.read_until(“login: “) tn.write(user + ” “) if password: tn.read_until(“Password: “) tn.write(password + ” “) tn.write(“ls “) tn.write(“exit “) print tn.read_all() At ActiveState you can find more Python scripts using the telnetlib, for example this script. For more information about using the Telnet client in Python, please see the official documentation.
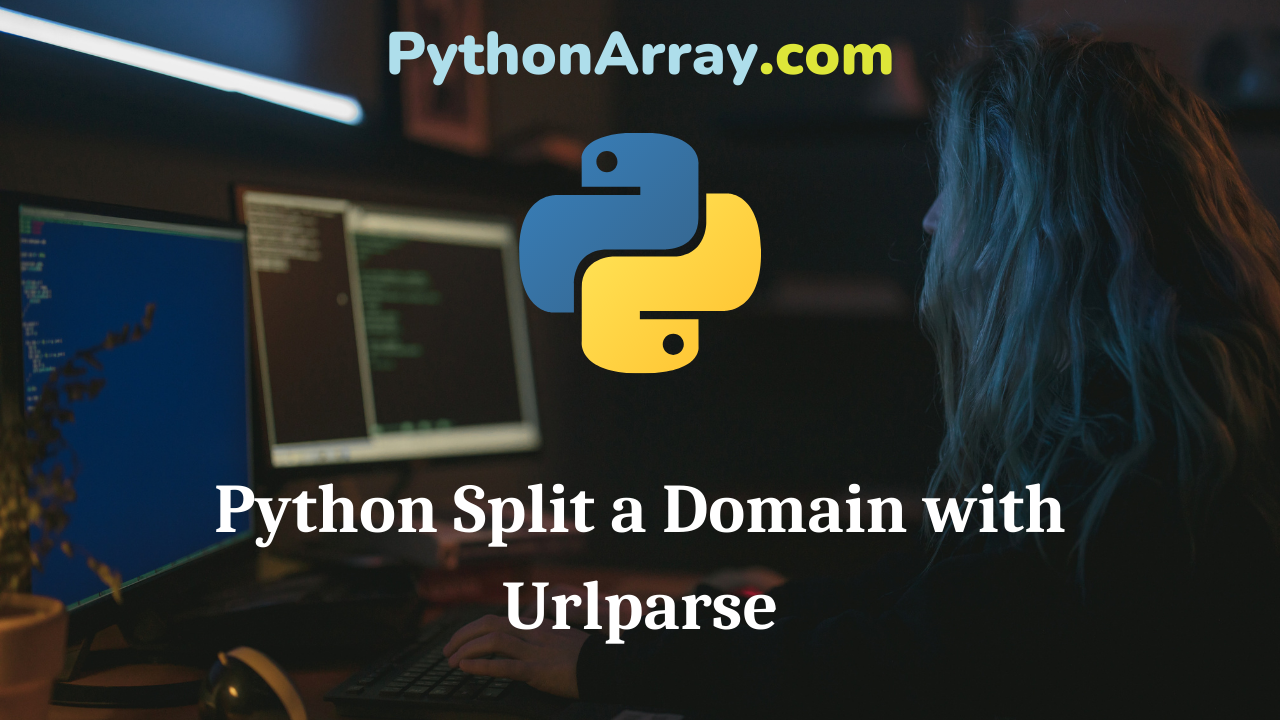
Python Split a Domain with Urlparse
Split a domain How to use the Hacker News API Writing Simple Views for Your First Python Django Application How to split string variables in Python This is a simple script to split the domain name from a URL. It does that by usingPythons urlparse module. import urlparse url = “http://python.org” domain = urlparse.urlsplit(url)[1].split(‘:’)[0] print “The domain name of the url is: “, domain