You can learn about Strings in Python Programs with Outputs helped you to understand the language better. Python Programming – Logical and Physical Line The string is an ordered sequence of letters/characters. It is enclosed in single (“) or double (” “) or triple quotation(‘”) marks. The quotes are not a part of the string. They only tell the computer where the string constant begins and ends. They can have any character or sign, including space in between them. ‘Hello, World!’ is a string; it is so-called because it contains a ‘string’ of letters. You can identify strings because they are enclosed in quotation marks. If you are not sure which type of value it is, the interpreter can tell you. >>> type(‘Hello, World!’) <class ‘str’> It is possible to change one type of value/variable to another type. It is known as type conversion or typecasting. The conversion can be done explicitly (the programmer specifies the conversion) or implicitly (the interpreter automatically converts the data type). An empty string contains no characters and has a length 0, represented by two quotation marks. The string with length 1 represents a character in Python. For example ‘a’, ‘b’, ‘c’ are strings of length one.…
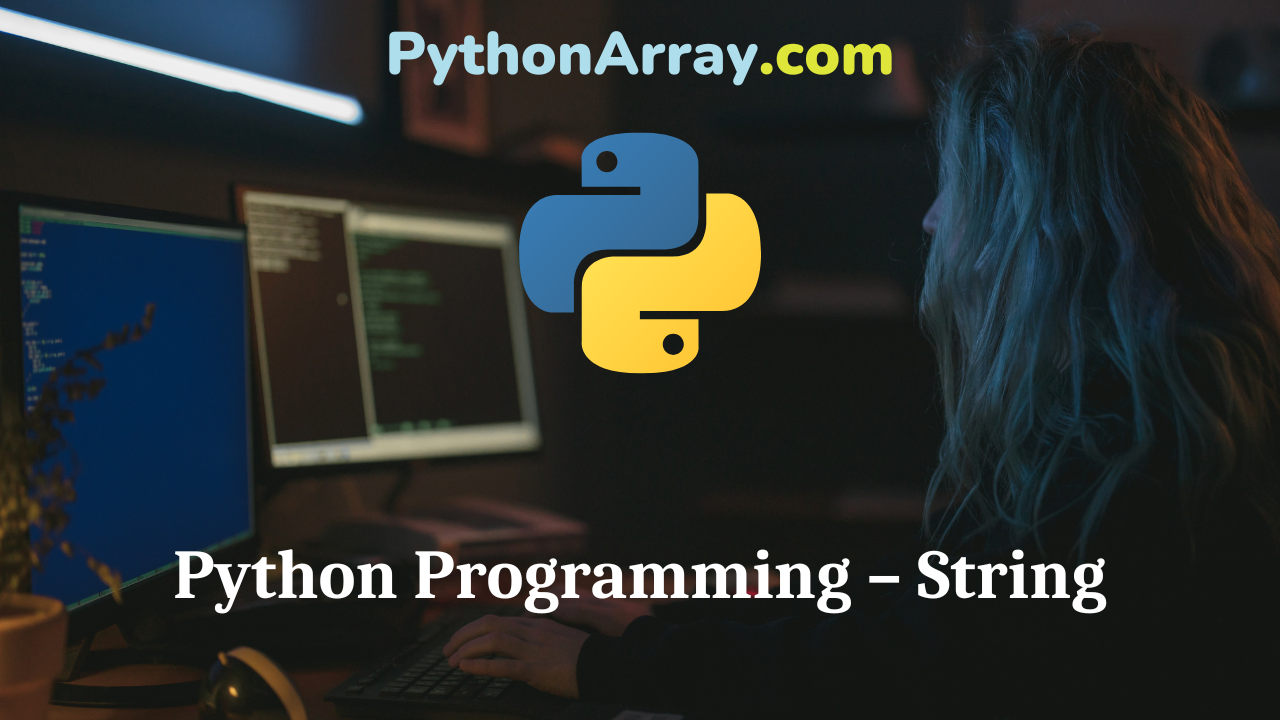
Python Programming – String
You can learn about Strings in Python Programs with Outputs helped you to understand the language better. Python Programming – String A string is a sequence of one or more characters (letters, numbers, symbols) that can be either a constant or a variable. Strings are the form of data used in programming for storing and manipulating text, such as words, names, and sentences. They are immutable data types, i.e., they are unchanging. You can write them in Python using single quotes, double quotes, or triple quotes. The quotes are not part of the string. They only tell the computer where the string constant begins and ends. String literals are written by enclosing a sequence of characters in single quotes (‘hello’), double quotes (“hello”) or triple quotes (‘”hello”‘ or “””hello”””). Strings are objects of Python’s built-in class ‘str’. A string value is a collection of one or more characters put in single, double, or triple quotes. Creating String To create a string, put the sequence of characters inside single quotes, double quotes, or triple quotes and then assign it to a variable. The single quotes and double quotes work in the same manner for the string creation. Triple quotes can be used in…
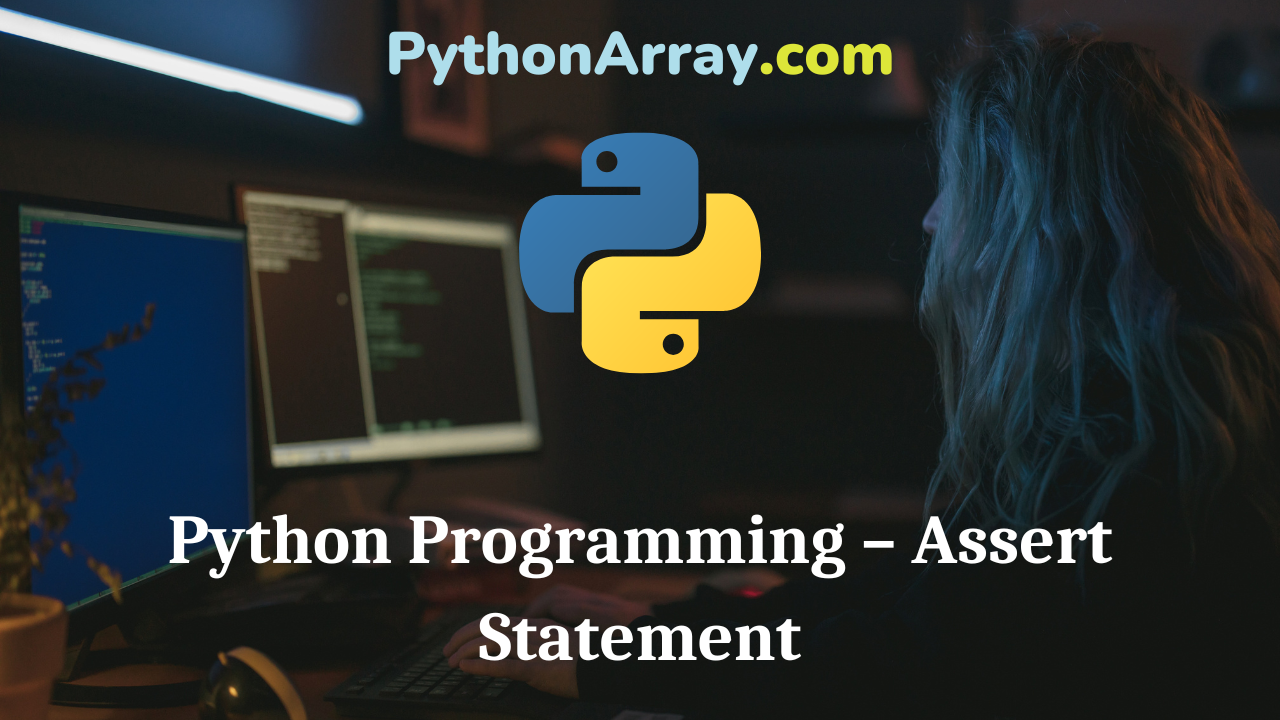
Python Programming – Assert Statement
You can learn about Control Statements in Python Programs with Outputs helped you to understand the language better. Python Programming – Assert Statement The assert statement checks if a given logical expression is true; or false. The program executes only if the expression is true and otherwise it raises the AssertionError. The syntax of assert is: assert Expression[, Arguments] Example Demo of an assert statement. # Demo of the assert statement num=int(input(‘Enter a number: ‘)) assert num>=0 print(‘You entered: nun) RUN >>> Enter a number: 8 You entered: 8 >>> >>> Enter a number: -4 Traceback (most recent call last): File “C:\Users\shash\AppData\Local\Programs\Python\Python37-32\assert.py”, line 2, in <module> assert num>=0 AssertionError >>> Python Programming – If Statement Python Programming – If-Else Statement Python Programming – The While Statement The print statement will display if the entered number is greater than or equal to 0. Negative numbers result in aborting the program after showing the AssertionError as shown in Figure 5.56. So, Assertions are simply boolean expressions that check if the condition returns true or not. If it is true, the program does nothing and moves to the next line of code. However, if it’s false, the program stops and throws an error. Python assert…
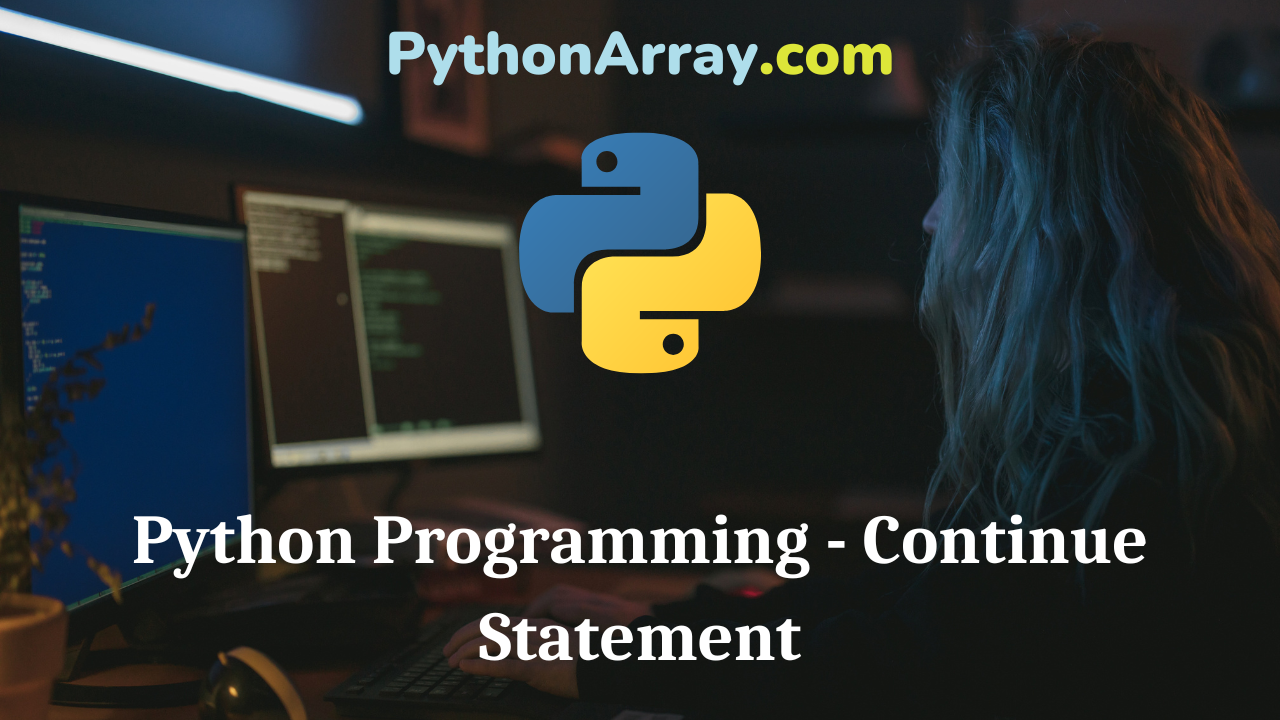
Python Programming – Continue Statement
You can learn about Control Statements in Python Programs with Outputs helped you to understand the language better. Python Programming – Continue Statement The continue statement forces the next iteration of the loop to take place, skipping any statement(s) that follows the continue statement in the current iteration, i.e., the continue statement jumps back to the top of the loop for the next iteration. Example 46. Demo of continue statement. # Demo of continue statement count=0 while True: count= count +1 # End loop if count is greater than 10 if (count > 10): break # Skip 5 if (count == 5): continue print(count)RUN >>> 1 2 3 4 5 6 7 8 9 10 >>> Figure 5.53 shows the use of the continue statement. At the top of the loop, the while condition is tested, and the loop is entered, if it’s true. Here, when the count is equal to 5, the program does not get to the print count) statement. So, the number 5 is skipped with the continue statement, and the loop ends with the break statement.’ Note in Figure 5.54, U is skipped due to the use of the continue statement. Python Programming – Conditional and Iterative Statements…
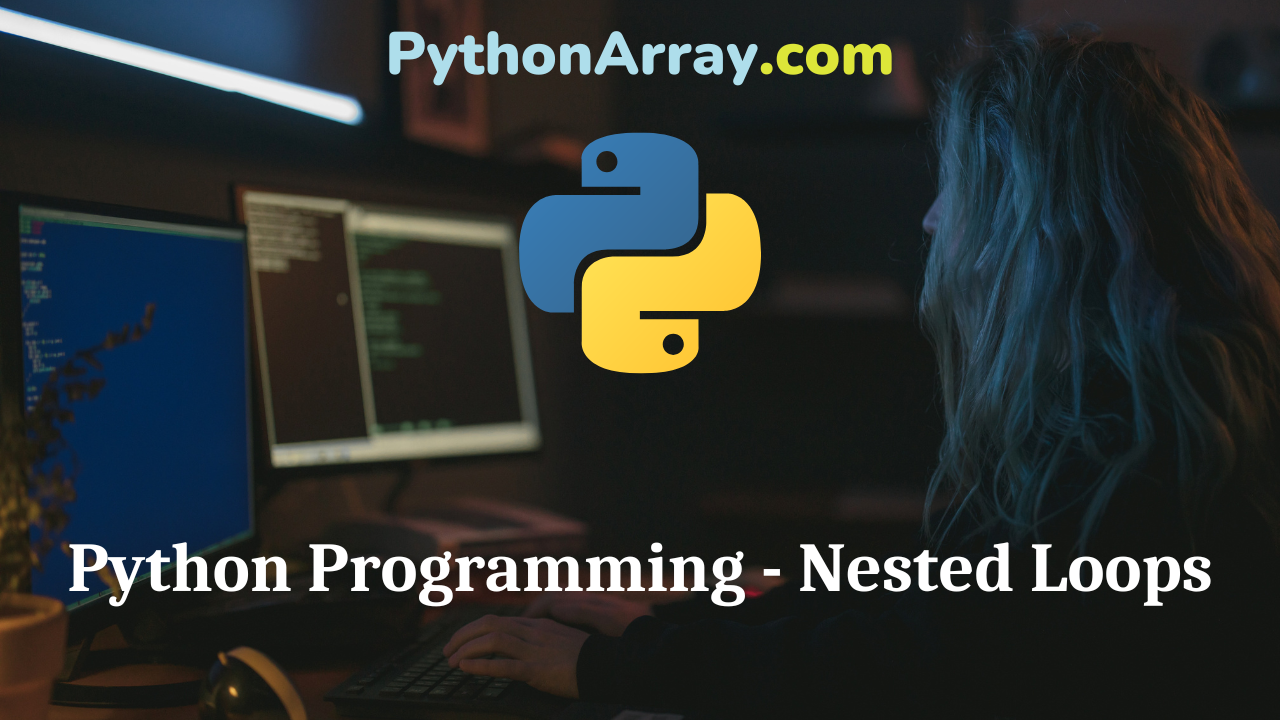
Python Programming – Nested Loops
You can learn about Control Statements in Python Programs with Outputs helped you to understand the language better. Python Programming – Nested Loops A loop may also contain another loop within its body. This form of a loop inside another loop is called a nested loop. In a nested loop construction, the inner loop must terminate before the ending of the outer loop. Example 36. Program to print pyramid of stars using for loop. * * * * * * * * * * * * * * * # Nested for loop n = int(input(“Enter a number to form pyramid :”)) for i in range(l,n): for j in range(l,i): print(“*”,end =” “) print( ) Python Programming – Conditional and Iterative Statements Python Programming – Flowcharts for Sequential, Decision-Based and Iterative Processing Introduction to Python Programming – Testing and Debugging Example37. Program to print inverted triangle of stars using for loop. * * * * * * * * * * * * * * * # Print the pattern for a in range(5, 0, -1): for b in range(1,a+1): print(“*”, end=’ ‘) print(” “) Example 38. Program to print patterns of stars. * * * * * * * * *…
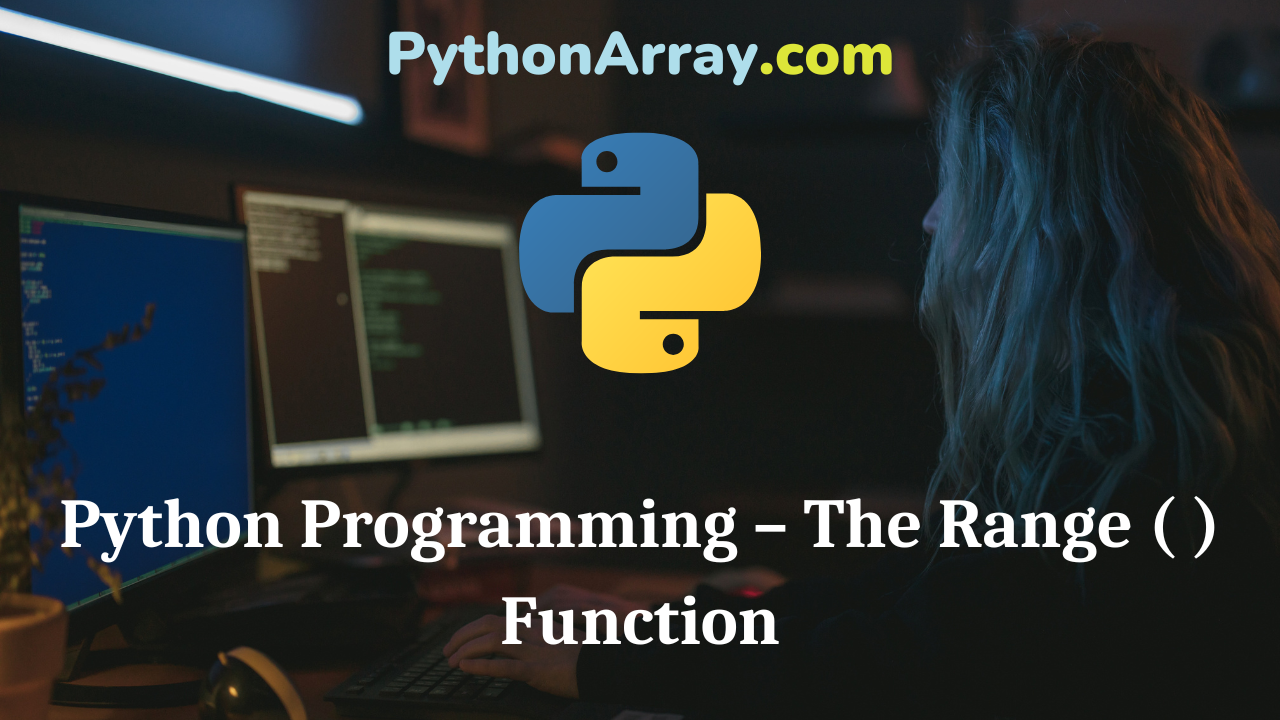
Python Programming – The Range ( ) Function
You can learn about Control Statements in Python Programs with Outputs helped you to understand the language better. Python Programming – The Range ( ) Function Python range ( ) function generates a list of numbers, which is generally used to iterate over with for loops. Python range ( ) function examples: >>> # One parameter >>> for i in range(5): . . . print(i) . . . 0 1 2 3 4 >>> # Two parameters >>> for i in range(3, 6): . . . print(i) . . . 3 4 5 >>> # Three parameters >>> for i in range(4, 10, 2): . . . print(i) . . . >>> # Going backwards >>> for i in range(0, -10, -2) . . . print(i) . . . 0 -2 -4 -6 -8 Python Programming – Print Statement Python Programming – Standard Data Types Python Programming – Accepting Input From The Console Let’s Try What is the output of the following code fragments? >>> for i in range(10): . . . print(i) >>> for i in range(0, -12, -2): . . . print(i) sum = 0 for i in range(16,2,-2): sum+ = i print (sum) for x in range(-300; 300, 100):…
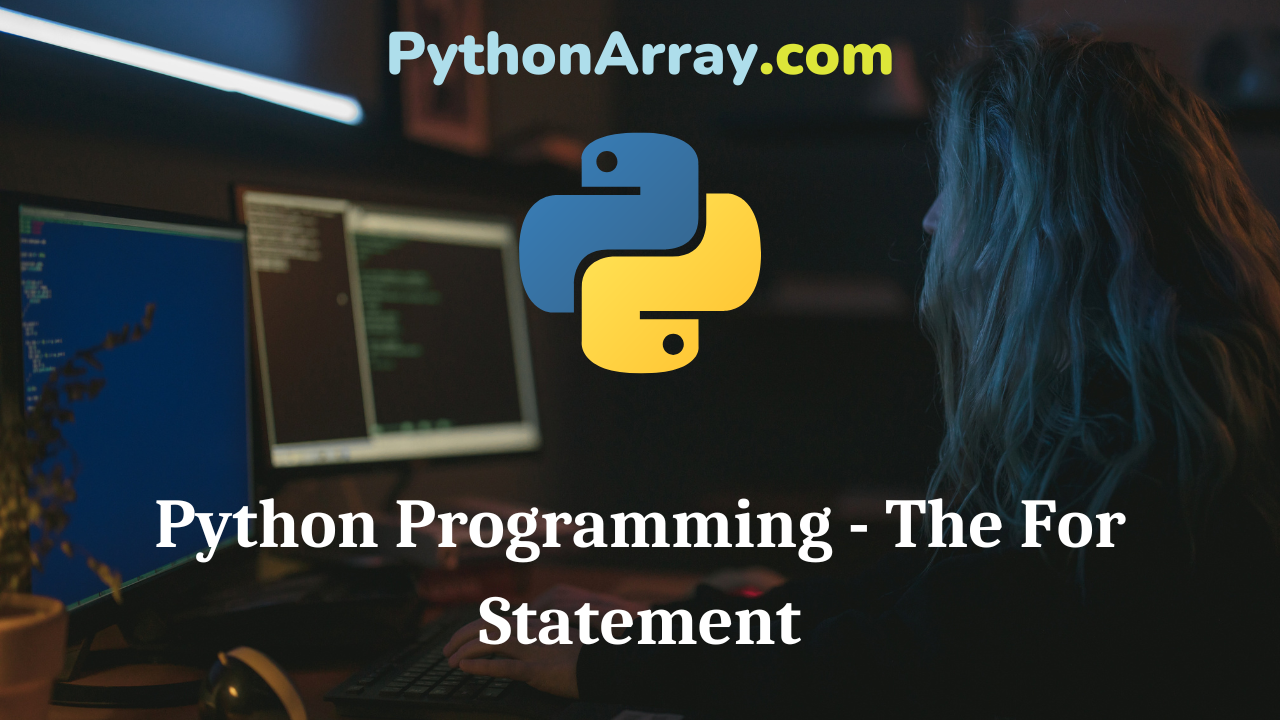
Python Programming – The For Statement
You can learn about Control Statements in Python Programs with Outputs helped you to understand the language better. Python Programming – The For Statement Python provides for statements as another form for accomplishing control using loops. The for loop statement is used to repeat a statement or a block of statements a specified number of times. A Python for loop has this general form, for <var> in <sequence>: <body> The body of the loop can be a sequence of Python statements. The start and end of the body can be indicated by its indentation under the loop heading. The variable after the keyword is called the loop index. It takes on each successive value in the sequence, and the statements in the body are executed once for each value. Python Programming – Flowcharts for Sequential, Decision-Based and Iterative Processing Python Programming – Python Character Set Python Programming – Conditional and Iterative Statements Usually, the sequence portion is a list of values. You can build a simple list by placing a sequence of expressions in square brackets. Some interactive examples help to illustrate the point: >>> for i in [ 0 , 1 , 2 , 3 ]: print ( i ) 0…
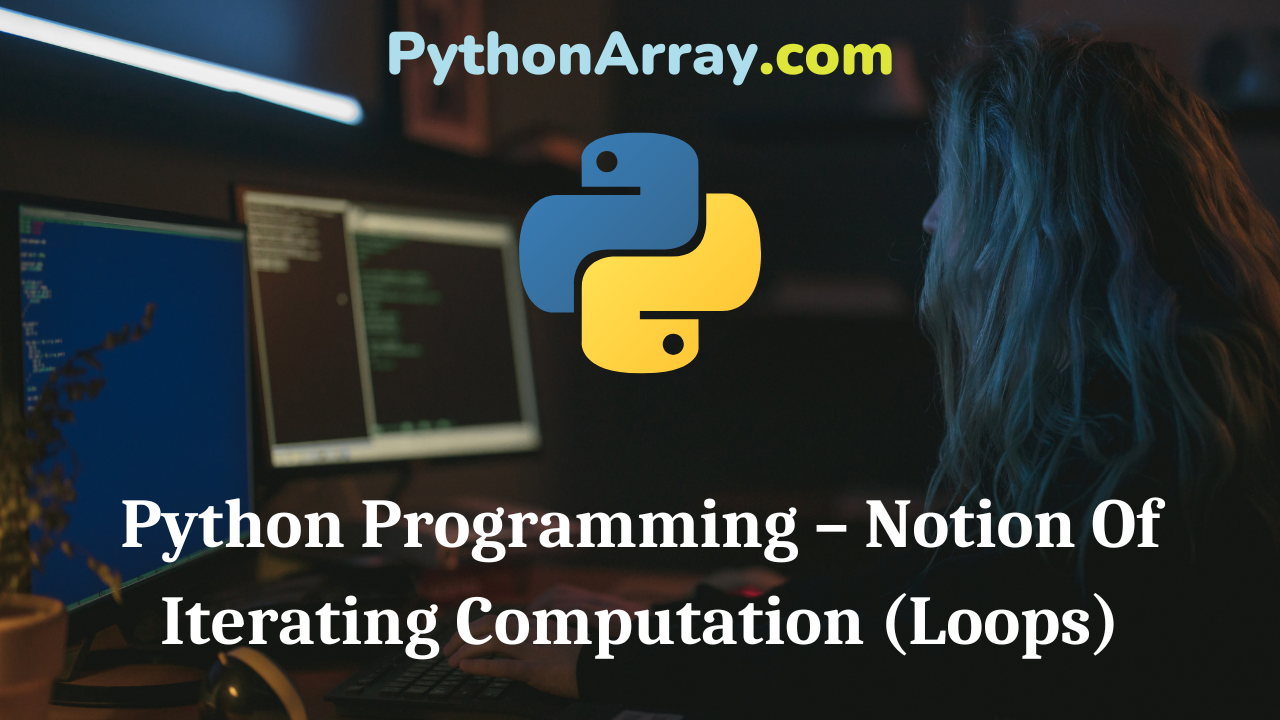
Python Programming – Notion Of Iterating Computation (Loops)
You can learn about Control Statements in Python Programs with Outputs helped you to understand the language better. Python Programming – Notion Of Iterating Computation (Loops) Many jobs that are required to be done with the help of a computer are repetitive in nature. For example, the calculation of wages of different workers in a factory is done using the formula (No. of hours worked) x (wage rate). This calculation will be performed by an accountant for each worker every month. Such types of repetitive calculations can easily be done with the help of a program that has a built-in loop. Figure 5.21 shows the flow diagram of the loop statement. Python Programming – Flowcharts for Sequential, Decision-Based and Iterative Processing Python Programming – Conditional and Iterative Statements Introduction to Python Programming – Interpreter What is a Loop? A loop is defined as a block of instructions repeated a certain number of times. Figure 5.22 illustrates a flowchart showing the concept of looping. It shows the flowchart for printing values 1, 2, 3…, 20. In Step 5 of Figure 5.22, the current value of A is compared with 21. If the current value of A is less than 21, steps 3 and…
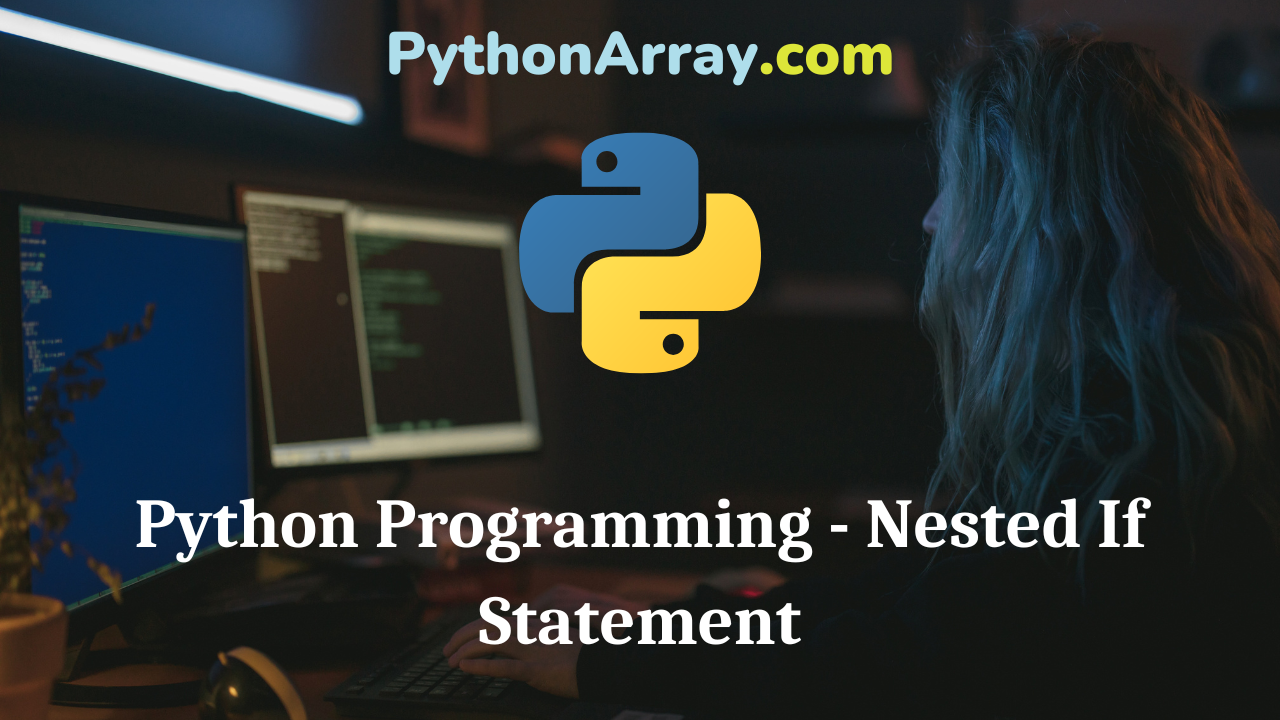
Python Programming – Nested If Statement
You can learn about Control Statements in Python Programs with Outputs helped you to understand the language better. Python Programming – Nested If Statement There may be a situation when you want to check for another condition after one condition evaluates to true. In such a situation, you can use the nested if construct. In a nested, if construct, you can have an if…elif…else construct inside another if…elif..else construct. Python Programming – Conditional and Iterative Statements Python Programming – Standard Data Types Python Programming – Flowcharts for Sequential, Decision-Based and Iterative Processing Example 16. Program to test whether the entered character is an alphabetic character or not. Suppose you want to test the ASCII code of an entered character to check if it is an alphabetic character. After checking this, you would like to further check if it is in lowercase or uppercase. Knowing that the ASCII codes for the uppercase letters are in the range of 65-90, and those for lowercase letters are in the range of 97-122, a program shown in Figure 5.20 establishes this logic. # Program to test – character is alphabetic character or not ch = input(“Enter an alphabetic character: “) if (ch>=’A’ and ch<=’Z’): print(“The character…
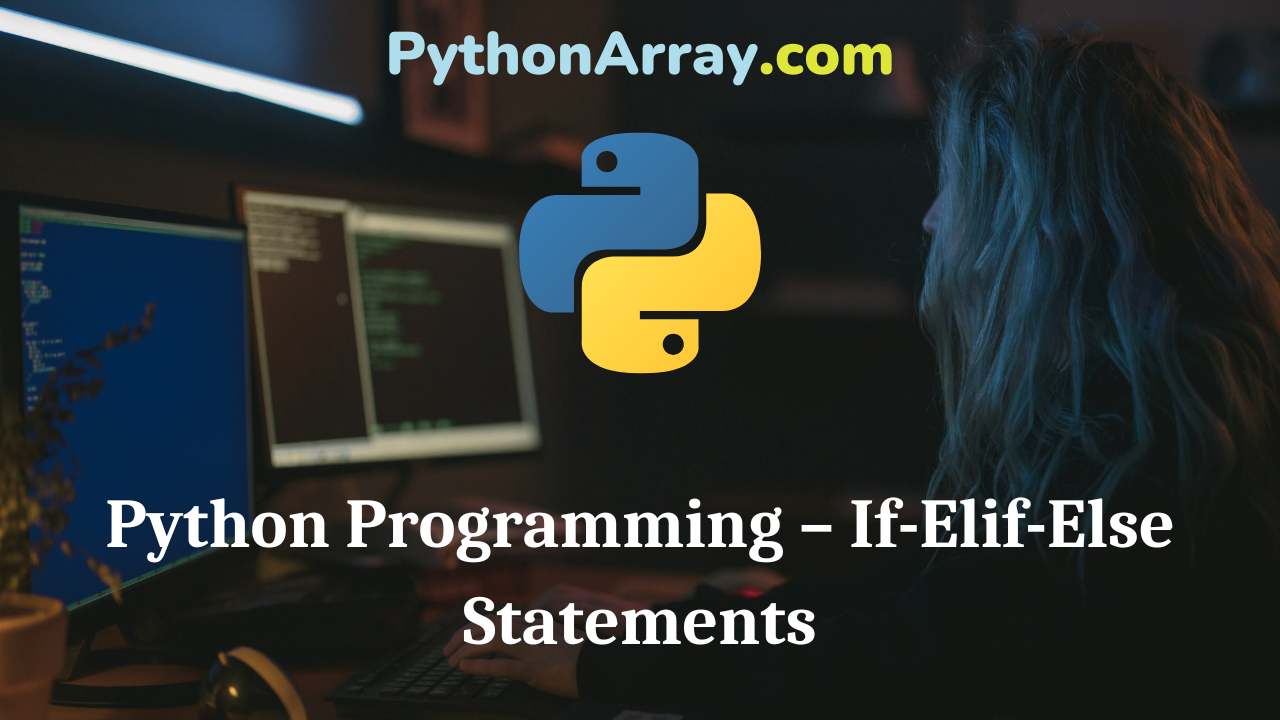
Python Programming – If-Elif-Else Statements
You can learn about Control Statements in Python Programs with Outputs helped you to understand the language better. Python Programming – If-Elif-Else Statements In the if statement, the statement following the condition or the keyword else is a single executable statement, such as an assignment statement. If multiple tasks are to be performed depending on a condition, then they can be grouped together within the block to form a single compound statement. Thus, you can use more than one statement by embedding the statements in a block. In the compound statement, there is no limit to the number of statements that can appear inside the body, but there has to be at least one. Indentation level is used to tell Python which statement(s) belongs to which block. Python Programming – Conditional and Iterative Statements Python Programming – Conditional Statements Python Programming – How To Start Python If you want to check for several conditions, you can use elif, which is short for “else if”. It is a combination of an if clause and an else clause. Like the else, the elif statement is optional. However, unlike else for which there can be at the most one statement, there can be unlimited number…